Coder
Time Limit: 20000/10000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)
Total Submission(s): 2547 Accepted Submission(s): 1013
Problem Description
In mathematics and computer science, an algorithm describes a set of
procedures or instructions that define a procedure. The term has become
increasing popular since the advent of cheap and reliable computers.
Many companies now employ a single coder to write an algorithm that will
replace many other employees. An added benefit to the employer is that
the coder will also become redundant once their work is done. 1
You are now the signle coder, and have been assigned a new task writing code, since your boss would like to replace many other employees (and you when you become redundant once your task is complete).
Your code should be able to complete a task to replace these employees who do nothing all day but eating: make the digest sum.
By saying “digest sum” we study some properties of data. For the sake of simplicity, our data is a set of integers. Your code should give response to following operations:
1. add x – add the element x to the set;
2. del x – remove the element x from the set;
3. sum – find the digest sum of the set. The digest sum should be understood by
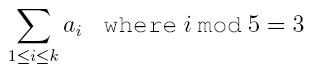
where the set S is written as {a1, a2, ... , ak} satisfying a1 < a2 < a3 < ... < ak
Can you complete this task (and be then fired)?
------------------------------------------------------------------------------
1 See http://uncyclopedia.wikia.com/wiki/Algorithm
You are now the signle coder, and have been assigned a new task writing code, since your boss would like to replace many other employees (and you when you become redundant once your task is complete).
Your code should be able to complete a task to replace these employees who do nothing all day but eating: make the digest sum.
By saying “digest sum” we study some properties of data. For the sake of simplicity, our data is a set of integers. Your code should give response to following operations:
1. add x – add the element x to the set;
2. del x – remove the element x from the set;
3. sum – find the digest sum of the set. The digest sum should be understood by
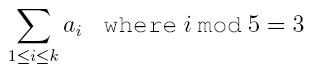
where the set S is written as {a1, a2, ... , ak} satisfying a1 < a2 < a3 < ... < ak
Can you complete this task (and be then fired)?
------------------------------------------------------------------------------
1 See http://uncyclopedia.wikia.com/wiki/Algorithm
Input
There’re several test cases.
In each test case, the first line contains one integer N ( 1 <= N <= 105 ), the number of operations to process.
Then following is n lines, each one containing one of three operations: “add x” or “del x” or “sum”.
You may assume that 1 <= x <= 109.
Please see the sample for detailed format.
For any “add x” it is guaranteed that x is not currently in the set just before this operation.
For any “del x” it is guaranteed that x must currently be in the set just before this operation.
Please process until EOF (End Of File).
In each test case, the first line contains one integer N ( 1 <= N <= 105 ), the number of operations to process.
Then following is n lines, each one containing one of three operations: “add x” or “del x” or “sum”.
You may assume that 1 <= x <= 109.
Please see the sample for detailed format.
For any “add x” it is guaranteed that x is not currently in the set just before this operation.
For any “del x” it is guaranteed that x must currently be in the set just before this operation.
Please process until EOF (End Of File).
Output
For each operation “sum” please print one line containing exactly one
integer denoting the digest sum of the current set. Print 0 if the set
is empty.
Sample Input
9
add 1
add 2
add 3
add 4
add 5
sum
add 6
del 3
sum
6
add 1
add 3
add 5
add 7
add 9
sum
Sample Output
3
4
5
线段树:
区间一个维护该集合的个数,一个维护模5之后该集合对应1,2,3,4,0即sum[0,1,2,3,4](一一对应记录)的和。当添加一个数时,对应区间更新之后再向上更新。更新父区间时,等于左区间的Lsum[0,1,2,3,4]+右区间的Rsum[0,1,2,3,4]加上左区间个数来说。比如左区间有num个数,假设num%5 = 3,则sum[0] = Lsum[0] + Rsum[2]因为右区间的第三个数相当于整个左右区间模5余1。
sum[1] = Lsum[1] + Rsum[3],
sum[2] = Lsum[2] + Rsum[4],
sum[3] = Lsum[3] + Rsum[0],
sum[4] = Lsum[4] + Rsum[1];
即:sum[i] = Lsum[i] + Rsum[(((i - (num%5)) % 5 + 5) % 5]
1 #include <stdio.h> 2 #include <string.h> 3 #include <algorithm> 4 #include <iomanip> 5 #include <set> 6 #include <map> 7 #include <vector> 8 #include <queue> 9 #define N 100005 10 #define llt long long int 11 using namespace std; 12 13 int num[N << 2];//记录区间的个数 14 llt segtree[N << 2][5];// 15 struct node 16 { 17 int x; 18 char s[4]; 19 }a[N]; 20 int b[N]; 21 map<int, int> mp;//使用map离散化 22 void build(int l, int r, int p) 23 { 24 memset(segtree[p], 0, sizeof(segtree[p])); 25 num[p] = 0; 26 if (l < r) 27 { 28 int mid = (l + r) >> 1, pp = p << 1; 29 build(l, mid, pp); 30 build(mid + 1, r, pp + 1); 31 } 32 } 33 void update(int l, int r, int p, int pos, int v) 34 { 35 if (pos == l && pos == r) 36 { 37 segtree[p][0] += 1ll * v; 38 num[p] = v > 0 ? 1 : 0; 39 return; 40 } 41 int mid = (l + r) >> 1, pp = p << 1; 42 if (mid >= pos) 43 update(l, mid, pp, pos, v); 44 else 45 update(mid + 1, r, pp + 1, pos, v); 46 for (int i = 0; i < 5; i++) 47 segtree[p][i] = segtree[pp][i] + segtree[pp + 1][((i - num[pp]) % 5 + 5) % 5]; 48 num[p] = num[pp] + num[pp + 1]; 49 } 50 int main() 51 { 52 int n, i, k; 53 while (~scanf("%d", &n)) 54 { 55 k = 1; 56 mp.clear(); 57 for (i = 1; i <= n; i++) 58 { 59 scanf("%s", a[i].s); 60 if (a[i].s[0] == 'a') 61 { 62 scanf("%d", &a[i].x); 63 b[k++] = a[i].x; 64 } 65 else 66 if (a[i].s[0] == 'd') 67 { 68 scanf("%d", &a[i].x); 69 a[i].x = -a[i].x; 70 } 71 } 72 build(1, k - 1, 1); 73 sort(b + 1, b + k); 74 for (i = 1; i < k; i++) 75 mp[b[i]] = i;//重新编号 76 for (i = 1; i <= n; i++) 77 { 78 if (a[i].s[0] == 's') 79 printf("%I64d ", segtree[1][2]); 80 else 81 update(1, k - 1, 1, mp[abs(a[i].x)], a[i].x); 82 } 83 } 84 return 0; 85 }