Problem Description
A ring is compose of n circles as shown in diagram. Put natural number 1, 2, ..., n into each circle separately, and the sum of numbers in two adjacent circles should be a prime.
Note: the number of first circle should always be 1.
Note: the number of first circle should always be 1.
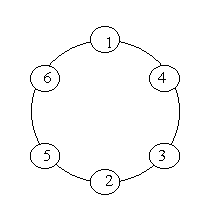
Input
n (0 < n < 20).
Output
The output format is shown as sample below. Each row represents a series of circle numbers in the ring beginning from 1 clockwisely and anticlockwisely. The order of numbers must satisfy the above requirements. Print solutions in lexicographical order.
You are to write a program that completes above process.
Print a blank line after each case.
You are to write a program that completes above process.
Print a blank line after each case.
Sample Input
6
8
Sample Output
Case 1:
1 4 3 2 5 6
1 6 5 2 3 4
Case 2:
1 2 3 8 5 6 7 4
1 2 5 8 3 4 7 6
1 4 7 6 5 8 3 2
1 6 7 4 3 8 5 2
简单DFS,不多说什么了
#include <stdio.h> #include <string.h> int n,prime[50],a[50],vis[50]; void isprime() { int i,j; for(i = 0;i<50;i++) prime[i] = 1; prime[0] = prime[1] = 0; for(i = 2;i<50;i++) { if(prime[i]) for(j = i+i;j<50;j+=i) prime[j] = 0; } } void dfs(int step) { int i,j; if(step == n+1 && prime[a[n]+a[1]])//结束条件 { for(i = 1;i<n;i++) printf("%d ",a[i]); printf("%d\n",a[n]); return ; } for(i = 2;i<=n;i++) { if(!vis[i] && prime[i+a[step-1]])//此数未用并且与上一个放到环中的数相加是素数 { a[step] = i; vis[i] = 1; dfs(step+1); vis[i] = 0; } } } int main() { int cas = 1; a[1] = 1; isprime(); while(~scanf("%d",&n)) { memset(vis,0,sizeof(vis)); printf("Case %d:\n",cas++); dfs(2); printf("\n"); } return 0; }