Time Limit: 4000/2000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others)
Total Submission(s): 43088 Accepted Submission(s): 19128
Problem Description
A ring is compose of n circles as shown in diagram. Put natural number 1, 2, ..., n into each circle separately, and the sum of numbers in two adjacent circles should be a prime.
Note: the number of first circle should always be 1.
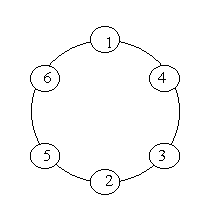
Note: the number of first circle should always be 1.
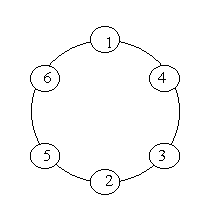
Input
n (0 < n < 20).
Output
The output format is shown as sample below. Each row represents a series of circle numbers in the ring beginning from 1 clockwisely and anticlockwisely. The order of numbers must satisfy the above requirements. Print solutions in lexicographical order.
You are to write a program that completes above process.
Print a blank line after each case.
You are to write a program that completes above process.
Print a blank line after each case.
Sample Input
6
8
Sample Output
Case 1:
1 4 3 2 5 6
1 6 5 2 3 4
Case 2:
1 2 3 8 5 6 7 4
1 2 5 8 3 4 7 6
1 4 7 6 5 8 3 2
1 6 7 4 3 8 5 2
#include<iostream> #include<cmath> #include<string.h> #include<stdio.h> using namespace std; const int N=25; int a[N]; int vis[N],ans[N]; bool prime(int x) { int i; for(i=2;i<=sqrt(x);i++) { if(x%i==0) break; } if(i>sqrt(x)) return true; else return false; } void dfs(int k,int n) { if(k==n+1&&prime(ans[n]+ans[1]))//不要忘记他是一个环,首尾也不能相加是素数哦! { for(int i=1;i<=n;i++) { if(i!=1) cout<<" "<<ans[i]; else cout<<ans[i]; } cout<<endl; return ; } for(int i=2;i<=n;i++) { if(prime(ans[k-1]+a[i])&&!vis[a[i]]) { vis[a[i]]=1; ans[k]=a[i]; dfs(k+1,n); vis[a[i]]=0; } } } int main() { int n; int cas=1; while(cin>>n) { memset(a,0,sizeof(a)); memset(ans,0,sizeof(ans)); memset(vis,0,sizeof(vis)); for(int i=1;i<=n;i++) { a[i]=i; } ans[1]=1;vis[1]=1; printf("Case %d: ",cas++); dfs(2,n); printf(" "); } return 0; }