该demo由前端请求后台服务器获取数据进行渲染
- 使用到的技术点
- 1.使用到的vue指令:
{{}}
v-if
v-for
v-model
- 2.使用到的事件:
@click
点击事件,@keyup.enter
回车- 3.使用到的事件修饰符:
.prevent
阻止事件默认行为- 4.使用
vue-resource
发起请求获取服务端返回的数据- 5.使用生命周期函数
created()
,即在页面渲染前请求用户列表- 6.自定义私有过滤器
- 7.js base64编码:
window.btoa()
, base64解码:window.atob()
- 后台接口说明
获取用户信息列表
key | value | 备注 |
---|---|---|
url | http://192.168.1.40:9001/hubtest/user/getUserList |
|
method | get |
7 |
请求体
无
响应体
{
"code":0,
"data":[
{
"id":0,
"password":"123456",
"username":"macy"
}
],
"message":"success"
}
添加用户
key | value | 备注 |
---|---|---|
url | http://192.168.1.40:9001/hubtest/user/addUser |
|
method | post |
|
Content-Type | application/json |
请求体
{
"username":"macy",
"password":"123"
}
响应体
{
"code":0,
"data":{
"id":1566550425674,
"password":"123",
"username":"macy"
},
"message":"success"
}
根据用户id删除用户
key | value | 备注 |
---|---|---|
url | http://192.168.1.40:9001/hubtest/user/deleteUserById/{userId} |
|
method | get |
请求体
无
响应体
{
"code":0,
"data":{
"id":0,
"password":"123456",
"username":"macy"
},
"message":"success"
}
- 实例
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>用户管理案例</title>
<link rel="stylesheet" href="./css/bootstrap-4.3.1.css">
<script src="./js/vue-2.4.0.js"></script>
<script src="./js//vue-resource.js"></script>
<style>
body{
font-size: 14px;
}
</style>
</head>
<body>
<div id = "app">
<!-- 提示信息 -->
<!-- 当提示信息不为空且昵称为空时,则显示 -->
<div class="alert alert-danger mt-2" v-if = "errMsg != ''">
<span>{{ errMsg }}</span>
<!-- 把提示信息置为空,则不显示 -->
<button type="button" class="close" @click = "errMsg = ''">
<span aria-hidden="true">×</span>
</button>
</div>
<!-- 添加用户 -->
<form class="form-inline mb-2 mt-2">
<div class="form-group ml-2">
<label>用户名:</label>
<input type="text" class="form-control" v-model = "username">
</div>
<div class="form-group ml-2">
<label>密码:</label>
<input type="text" class="form-control" v-model = "password">
</div>
<div class="form-group ml-2">
<input type="button" class="btn btn-primary btn-sm" value="添加" @click = "addUser">
</div>
<div class="form-group ml-2">
<!-- 回车搜索 -->
<input type="text" class="form-control" placeholder="search..." v-model = "keyword" @keyup.enter= "getFilterUserList">
</div>
</form>
<!-- 显示用户列表 -->
<table class="table table-bordered" >
<thead>
<tr>
<th>编号</th>
<th>用户名</th>
<th>密码</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr v-for = "item in filterUserList" :key = "item.id">
<td>{{ item.id }}</td>
<td>{{ item.username }}</td>
<!-- 使用过滤器对密码进行格式化 -->
<td>{{ item.password | passwordFormat }}</td>
<td>
<!-- .prevent禁止默认行为,如果不加上,vue会报语法错误 -->
<a href="javascript:void()" class="btn btn-link btn-sm" @click.prevent = "delUser(item.id)">删除</a>
</td>
</tr>
</tbody>
</table>
</div>
<script>
var vue = new Vue({
el : '#app',
data : {
errMsg : '',//错误提示信息
keyword : '',//搜索关键字
username : '',//用户名
password : '',//密码
userList : [],//用户列表
filterUserList :[]//过滤后的用户列表
},
// 生命周期函数,当创建vue实例后,调用获取用户列表的接口
created(){
this.getUserList();
},
methods : {
//查询用户列表
getUserList(){
//调用获取用户列表接口
this.$http.get("http://192.168.1.40:9001/hubtest/user/getUserList").then(
resp => {//成功时回调
// 获取服务端返回的数据赋值到data属性的userList中
this.userList = this.filterUserList = resp.body.data;
},
resp => {//失败时回调
}
);
},
//添加用户
addUser(){
//1.对字段进行判空
if (this.username === ''){
return this.errMsg = '用户名不为空';
}
if (this.password === ''){
return this.errMsg = '密码不为空';
}
//2.调用添加用户接口
this.$http.post("http://192.168.1.40:9001/hubtest/user/addUser",{username:this.username,password:this.password}).then(
resp => {
//当添加用户成功时,重新加载用户列表
this.getUserList();
},
resp => {}
);
//3.去除错误提示信息和用户信息
this.errMsg = this.username = this.password = '';
},
//删除用户
delUser(userId){
this.$http.get("http://192.168.1.40:9001/hubtest/user/deleteUserById/"+userId).then(
resp => {
//删除用户成功时,重新加载用户列表
this.getUserList();
},
resp => {}
);
},
// 根据关键字过滤用户
getFilterUserList(){
//过滤前把用户列表添加到过滤的列表,否则一次过滤后,该数组的记录不是最初的数据
this.filterUserList = this.userList;
//根据关键字过滤
this.filterUserList = this.filterUserList.filter((item) => {
if (item.username.includes(this.keyword)){
return item;
}
});
}
},
filters:{
// 定义密码base64编码过滤器
passwordFormat(input){
//把密码明文使用base64编码
//编码:window.btoa()
//解码:window.atob()
return btoa(input);
}
}
});
</script>
</body>
</html>
- 效果 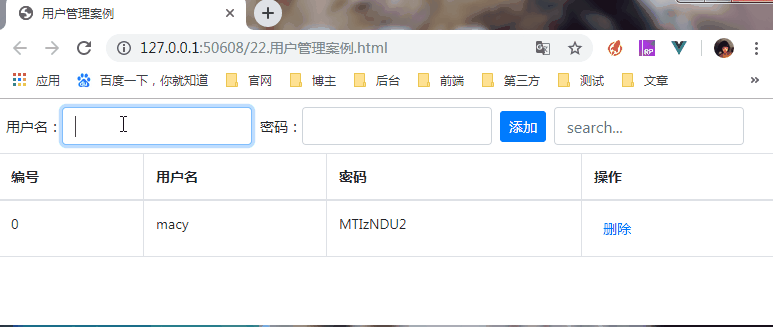