Bone Collector
Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)
Total Submission(s): 12318 Accepted Submission(s): 4787
Problem Description
Many years ago , in Teddy’s hometown there was a man who was called “Bone Collector”. This man like to collect varies of bones , such as dog’s , cow’s , also he went to the grave …
The bone collector had a big bag with a volume of V ,and along his trip of collecting there are a lot of bones , obviously , different bone has different value and different volume, now given the each bone’s value along his trip , can you calculate out the maximum of the total value the bone collector can get ?
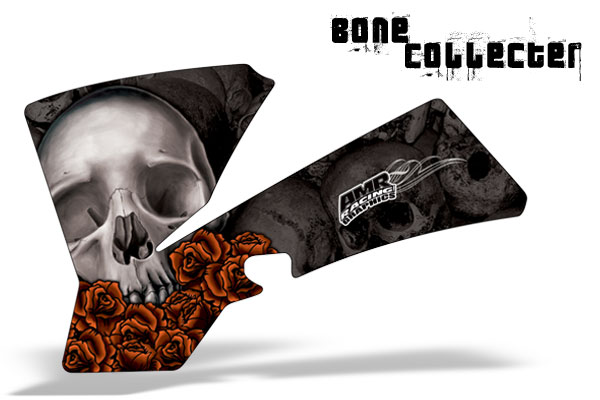
The bone collector had a big bag with a volume of V ,and along his trip of collecting there are a lot of bones , obviously , different bone has different value and different volume, now given the each bone’s value along his trip , can you calculate out the maximum of the total value the bone collector can get ?
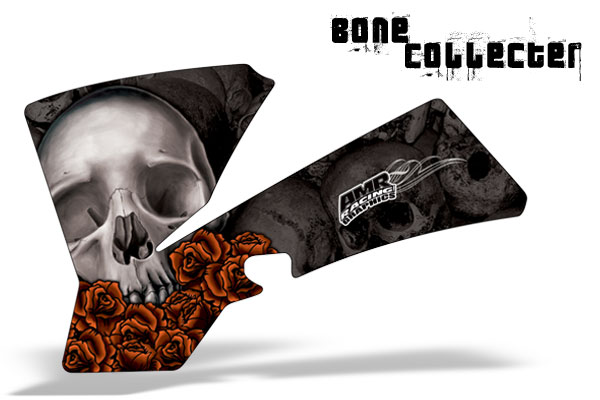
Input
The first line contain a integer T , the number of cases.
Followed by T cases , each case three lines , the first line contain two integer N , V, (N <= 1000 , V <= 1000 )representing the number of bones and the volume of his bag. And the second line contain N integers representing the value of each bone. The third line contain N integers representing the volume of each bone.
Followed by T cases , each case three lines , the first line contain two integer N , V, (N <= 1000 , V <= 1000 )representing the number of bones and the volume of his bag. And the second line contain N integers representing the value of each bone. The third line contain N integers representing the volume of each bone.
Output
One integer per line representing the maximum of the total value (this number will be less than 231).
Sample Input
1
5 10
1 2 3 4 5
5 4 3 2 1
Sample Output
14
Author
Teddy
Source
Recommend
lcy
1 // Project name : 2602 ( Bone Collector ) 2 // File name : main.cpp 3 // Author : Izumu 4 // Date & Time : Sat Jul 14 10:03:36 2012 5 6 7 #include <iostream> 8 #include <stdio.h> 9 #include <string> 10 #include <cmath> 11 #include <algorithm> 12 using namespace std; 13 14 #define MAXN 1010 15 16 int value[MAXN]; 17 int cost[MAXN]; 18 19 int dp[MAXN][MAXN]; 20 21 int n, v; 22 23 int max(int a, int b) 24 { 25 return (a > b)? a: b; 26 } 27 28 void DynamicProgramming() 29 { 30 // init for dp[][] 31 for (int i = 0; i <= n; i++) 32 { 33 for (int j = 0; j <= v; j++) 34 { 35 dp[i][j] = 0; 36 } 37 } 38 39 for (int i = 1; i <= n; i++) 40 { 41 for (int j = 0; j <= v; j++) 42 { 43 if (cost[i] <= j) 44 { 45 dp[i][j] = max( 46 dp[i-1][j], 47 dp[i-1][j-cost[i]] + value[i] 48 ); 49 } 50 else 51 { 52 dp[i][j] = dp[i-1][j]; 53 } 54 } 55 } 56 57 58 } 59 int main() 60 { 61 int t; 62 cin >> t; 63 while (t--) 64 { 65 cin >> n >> v; 66 for (int i = 1; i <= n; i++) 67 { 68 cin >> value[i]; 69 } 70 for (int i = 1; i <= n; i++) 71 { 72 cin >> cost[i]; 73 } 74 75 DynamicProgramming(); 76 77 cout << dp[n][v] << endl; 78 } 79 return 0; 80 } 81 82 // end 83 // ism