第三天笔记
1 课程计划
后台管理商品的添加功能
1、商品分类选择
2、上传图片
3、富文本编辑器(kindEditor)
4、实现商品的添加
5、课后作业(商品的修改、删除)
2 商品添加功能说明
3 类目选择
3.1 需求
点击类目选择按钮弹出类目选择窗口,窗口中是一个树形视图。分级展示商品分类。当选择商品分类的叶子节点后,关闭窗口并将选中的商品分类的名称显示到网页上。
1、初始化tree的url:
/item/cat/list
2、请求的参数
Id(当前节点的id,根据此id查询子节点)
3、返回数据的格式json数据:
[{
"id": 1, //当前节点的id
"text": "Node 1", //节点显示的名称
"state": "closed" //节点的状态,如果是closed就是一个文件夹形式,
// 当打开时还会 做一次请求。如果是open就显示为叶子节点。
},{
"id": 2,
"text": "Node 2",
"state": "closed"
}]
3.2 Mapper
3.2.1 数据中的表:
3.2.2 Sql语句
SELECT * FROM `tb_item_cat` where parent_id=父节点id;
可以使用 逆向工程生成的mapper。
3.3 Service层
功能:根据parentId查询商品分类列表。
参数:parentId
返回值:返回tree所需要的数据结构,是一个节点列表。
可以创建一个tree node的pojo表示节点的数据,也可以使用map。
List<TreeNode>
3.3.1 创建一个tree node的pojo:
是一个通用的pojo可以放到taotao-common中。
1 public class TreeNode { 2 3 private long id; 4 5 private String text; 6 7 private String state; 8 9 public TreeNode(long id, String text, String state) { 10 11 this.id = id; 12 13 this.text = text; 14 15 this.state = state; 16 17 } 18 19 public long getId() { 20 21 return id; 22 23 } 24 25 public void setId(long id) { 26 27 this.id = id; 28 29 } 30 31 public String getText() { 32 33 return text; 34 35 } 36 37 public void setText(String text) { 38 39 this.text = text; 40 41 } 42 43 public String getState() { 44 45 return state; 46 47 } 48 49 public void setState(String state) { 50 51 this.state = state; 52 53 } 54 55 } 56 |
3.3.2 代码实现
1 @Service 2 3 public class ItemCatServiceImpl implements ItemCatService { 4 5 @Autowired 6 7 private TbItemCatMapper itemCatMapper; 8 9 @Override 10 11 public List<TreeNode> getItemCatList(long parentId) { 12 13 //根据parentId查询分类列表 14 15 TbItemCatExample example = new TbItemCatExample(); 16 17 //设置查询条件 18 19 Criteria criteria = example.createCriteria(); 20 21 criteria.andParentIdEqualTo(parentId); 22 23 //执行查询 24 25 List<TbItemCat> list = itemCatMapper.selectByExample(example); 26 27 //分类列表转换成TreeNode的列表 28 29 List<TreeNode> resultList = new ArrayList<>(); 30 31 for (TbItemCat tbItemCat : list) { 32 33 //创建一个TreeNode对象 34 35 TreeNode node = new TreeNode(tbItemCat.getId(), tbItemCat.getName(), 36 37 tbItemCat.getIsParent()?"closed":"open"); 38 39 resultList.add(node); 40 41 } 42 43 return resultList; 44 45 } 46 47 } 48 |
3.4 表现层
功能:接收页面传递过来的id,作为parentId查询子节点。
参数:Long id
返回值:要返回json数据要使用@ResponseBody。List<TreeNode>
1 /** 2 3 * 商品分类管理 4 5 * <p>Title: ItemCatController</p> 6 7 * <p>Description: </p> 8 9 * <p>Company: www.itcast.com</p> 10 11 * @author 入云龙 12 13 * @date 2015年8月15日上午9:49:18 14 15 * @version 1.0 16 17 */ 18 19 @Controller 20 21 @RequestMapping("/item/cat") 22 23 public class ItemCatController { 24 25 @Autowired 26 27 private ItemCatService itemCatService; 28 29 @RequestMapping("/list") 30 31 @ResponseBody 32 33 public List<TreeNode> getItemCatList(@RequestParam(value="id", defaultValue="0")Long parentId) { 34 35 List<TreeNode> list = itemCatService.getItemCatList(parentId); 36 37 return list; 38 39 } 40 41 } 42 |
4 图片上传
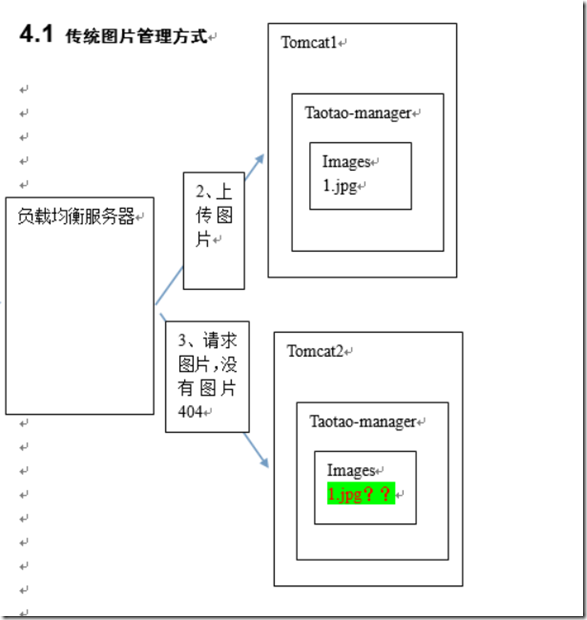
如果把图片放到工程中,在集群环境下,会出现找不到图片的情况。
4.2 集群环境下的上传图片
图片服务器两个服务:
http:可以使用nginx做静态资源服务器。也可以使用apache。推荐使用nginx,效率更高。
Nginx:
1、http服务
2、反向代理
3、负载均衡
ftp服务:
使用linux做服务器,在linux中有个ftp组件vsftpd。
4.3 图片服务器
4.3.1 搭建
<具体搭建步骤请参考以下文章《centos7 nginx图片 服务器可以访问ftp用户上传的图片资源的配置》、《CentOS7 搭建FTP服务器》和 《centos7_ linux : Nginx安装手册》《CentOS7 搭建FTP服务器》、《处理ftp服务器 在重启后ftp客户端不能连接访问的问题》>
要求安装vmware虚拟机。
Linux:CentOS6.4(32)
Nginx:1.8.0
Vsftpd:需要在线安装。
4.3.1.1 Linux关闭图像界面:
[root@bogon ~]# vim /etc/inittab
手动启动图像界面:
#startx
4.3.1.2 Nginx安装
1、nginx安装环境(详见nginx安装手册)
2、把nginx安装包上传到服务器。
3、解压缩
[root@bogon ~]# tar -zxf nginx-1.8.0.tar.gz
4、配置makefile
1 ./configure 2 3 --prefix=/usr/local/nginx 4 5 --pid-path=/var/run/nginx/nginx.pid 6 7 --lock-path=/var/lock/nginx.lock 8 9 --error-log-path=/var/log/nginx/error.log 10 11 --http-log-path=/var/log/nginx/access.log 12 13 --with-http_gzip_static_module 14 15 --http-client-body-temp-path=/var/temp/nginx/client 16 17 --http-proxy-temp-path=/var/temp/nginx/proxy 18 19 --http-fastcgi-temp-path=/var/temp/nginx/fastcgi 20 21 --http-uwsgi-temp-path=/var/temp/nginx/uwsgi 22 23 --http-scgi-temp-path=/var/temp/nginx/scgi 24
注意:上边将临时文件目录指定为/var/temp/nginx,需要在/var下创建temp及nginx目录
1 [root@bogon nginx-1.8.0]# mkdir /var/temp/nginx -p
5、编译安装
make
make install
6、启动nginx
7、关闭
1 [root@bogon sbin]# ./nginx -s stop
8、重新加载配置文件
1 [root@bogon sbin]# ./nginx -s reload
9、关闭防火墙
1)关闭
1 [root@bogon sbin]# service iptables stop 2 3 iptables: Flushing firewall rules: [ OK ] 4 5 iptables: Setting chains to policy ACCEPT: filter [ OK ] 6 7 iptables: Unloading modules: [ OK ] 8
2)也可以修改防火墙配置文件:
1 [root@bogon sbin]# vim /etc/sysconfig/iptables
修改后需要重启防火墙:
1 [root@bogon sbin]# service iptables restart
10、访问nginx服务
4.3.2 图片服务器的配置
<具体搭建步骤请参考以下文章《centos7 nginx图片 服务器可以访问ftp用户上传的图片资源的配置》、《CentOS7 搭建FTP服务器》和 《centos7_ linux : Nginx安装手册》《CentOS7 搭建FTP服务器》、《处理ftp服务器 在重启后ftp客户端不能连接访问的问题》>
4.3.2.1 测试ftp:
1、使用ftp客户端测试。
2、使用java代码访问ftp服务器
使用apache的FTPClient工具访问ftp服务器。需要在pom文件中添加依赖:
1 <dependency> 2 3 <groupId>commons-net</groupId> 4 5 <artifactId>commons-net</artifactId> 6 7 <version>${commons-net.version}</version> 8 9 </dependency> 10 |
1 public class FTPClientTest { 2 3 @Test 4 5 public void testFtp() throws Exception { 6 7 //1、连接ftp服务器 8 9 FTPClient ftpClient = new FTPClient(); 10 11 ftpClient.connect("192.168.25.133", 21); 12 13 //2、登录ftp服务器 14 15 ftpClient.login("ftpuser", "ftpuser"); 16 17 //3、读取本地文件 18 19 FileInputStream inputStream = new FileInputStream(new File("D:\Documents\Pictures\images\2010062119283578.jpg")); 20 21 //4、上传文件 22 23 //1)指定上传目录 24 25 ftpClient.changeWorkingDirectory("/home/ftpuser/www/images"); 26 27 //2)指定文件类型 28 29 ftpClient.setFileType(FTPClient.BINARY_FILE_TYPE); 30 31 //第一个参数:文件在远程服务器的名称 32 33 //第二个参数:文件流 34 35 ftpClient.storeFile("hello.jpg", inputStream); 36 37 //5、退出登录 38 39 ftpClient.logout(); 40 41 } 42 43 } 44 |
4.3.2.2 Nginx配置
需要把nginx的根目录指向ftp上传文件的目录。
需要nginx重新加载配置文件:
1 [root@bogon sbin]# ./nginx -s reload
4.4 把ftp代码封装成工具类
把工具类放到taotao-common中。
4.5 图片上传实现
4.5.1 需求分析
Common.js
1、绑定事件
2、初始化参数
3、上传图片的url:
/pic/upload
4、上图片参数名称:
uploadFile
5、返回结果数据类型json
参考文档:
http://kindeditor.net/docs/upload.html
返回格式(JSON)
//成功时 { "error" : 0, "url" : "http://www.example.com/path/to/file.ext" } //失败时 { "error" : 1, "message" : "错误信息" } |
4.5.2 Service
功能:接收controller层传递过来的图片对象,把图片上传到ftp服务器。给图片生成一个新的名字。
参数:MultiPartFile uploadFile
返回值:返回一个pojo,应该是PictureResult。
4.5.2.1 PictureResult
应该把此pojo放到taotao-common工程中。
1 /** 2 3 * 上传图片的返回结果 4 5 * <p>Title: PictureResult</p> 6 7 * <p>Description: </p> 8 9 * <p>Company: www.itcast.com</p> 10 11 * @author 入云龙 12 13 * @date 2015年8月15日下午2:53:21 14 15 * @version 1.0 16 17 */ 18 19 public class PictureResult { 20 21 private int error; 22 23 private String url; 24 25 private String message; 26 27 private PictureResult(int error, String url, String message) { 28 29 this.error = error; 30 31 this.url = url; 32 33 this.message = message; 34 35 } 36 37 //成功时调用的方法 38 39 public static PictureResult ok(String url) { 40 41 return new PictureResult(0, url, null); 42 43 } 44 45 //失败时调用的方法 46 47 public static PictureResult error(String message) { 48 49 return new PictureResult(1, null, message); 50 51 } 52 53 public int getError() { 54 55 return error; 56 57 } 58 59 public void setError(int error) { 60 61 this.error = error; 62 63 } 64 65 public String getUrl() { 66 67 return url; 68 69 } 70 71 public void setUrl(String url) { 72 73 this.url = url; 74 75 } 76 77 public String getMessage() { 78 79 return message; 80 81 } 82 83 public void setMessage(String message) { 84 85 this.message = message; 86 87 } 88 89 } 90 |
4.5.2.2 Service实现
1 /** 2 3 * 上传图片处理服务实现类 4 5 * <p>Title: PictureService</p> 6 7 * <p>Description: </p> 8 9 * <p>Company: www.itcast.com</p> 10 11 * @author 入云龙 12 13 * @date 2015年8月15日下午2:59:38 14 15 * @version 1.0 16 17 */ 18 19 @Service 20 21 public class PictureServiceImpl implements PictureService { 22 23 @Value("${FTP_ADDRESS}") 24 25 private String FTP_ADDRESS; 26 27 @Value("${FTP_PORT}") 28 29 private Integer FTP_PORT; 30 31 @Value("${FTP_USER_NAME}") 32 33 private String FTP_USER_NAME; 34 35 @Value("${FTP_PASSWORD}") 36 37 private String FTP_PASSWORD; 38 39 @Value("${FTP_BASE_PATH}") 40 41 private String FTP_BASE_PATH; 42 43 @Value("${IMAGE_BASE_URL}") 44 45 private String IMAGE_BASE_URL; 46 47 @Override 48 49 public PictureResult uploadPicture(MultipartFile uploadFile) { 50 51 //判断上传图片是否为空 52 53 if (null == uploadFile || uploadFile.isEmpty()) { 54 55 return PictureResult.error("上传图片为空"); 56 57 } 58 59 //取文件扩展名 60 61 String originalFilename = uploadFile.getOriginalFilename(); 62 63 String ext = originalFilename.substring(originalFilename.lastIndexOf(".")); 64 65 //生成新文件名 66 67 //可以使用uuid生成新文件名。 68 69 //UUID.randomUUID() 70 71 //可以是时间+随机数生成文件名 72 73 String imageName = IDUtils.genImageName(); 74 75 //把图片上传到ftp服务器(图片服务器) 76 77 //需要把ftp的参数配置到配置文件中 78 79 //文件在服务器的存放路径,应该使用日期分隔的目录结构 80 81 DateTime dateTime = new DateTime(); 82 83 String filePath = dateTime.toString("/yyyy/MM/dd"); 84 85 try { 86 87 FtpUtil.uploadFile(FTP_ADDRESS, FTP_PORT, FTP_USER_NAME, FTP_PASSWORD, 88 89 FTP_BASE_PATH, filePath, imageName + ext, uploadFile.getInputStream()); 90 91 } catch (Exception e) { 92 93 e.printStackTrace(); 94 95 return PictureResult.error(ExceptionUtil.getStackTrace(e)); 96 97 } 98 99 //返回结果,生成一个可以访问到图片的url返回 100 101 return PictureResult.ok(IMAGE_BASE_URL + filePath + "/" + imageName + ext); 102 103 } 104 105 } 106 |
4.5.3 Controller
功能:接收页面传递过来的图片。调用service上传到图片服务器。返回结果。
参数:MultiPartFile uploadFile
返回值:返回json数据,应该返回一个pojo,PictureResult对象。
1 @Controller 2 3 public class PictureController { 4 5 @Autowired 6 7 private PictureService pictureService; 8 9 @RequestMapping("/pic/upload") 10 11 @ResponseBody 12 13 public PictureResult upload(MultipartFile uploadFile) { 14 15 PictureResult result = pictureService.uploadPicture(uploadFile); 16 17 return result; 18 19 } 20 21 } 22 |
4.5.4 Springmvc.xml
1 <!-- 定义文件上传解析器 --> 2 3 <bean id="multipartResolver" 4 5 class="org.springframework.web.multipart.commons.CommonsMultipartResolver"> 6 7 <!-- 设定默认编码 --> 8 9 <property name="defaultEncoding" value="UTF-8"></property> 10 11 <!-- 设定文件上传的最大值5MB,5*1024*1024 --> 12 13 <property name="maxUploadSize" value="5242880"></property> 14 15 </bean> 16 |
5 富文本编辑器
5.1 使用方法
第一步:在jsp中加入富文本编辑器js的引用。
第二步:在富文本编辑器出现的位置添加一个input 类型为textarea
第三步:调用js方法初始化富文本编辑器。
第四步:提交表单时,调用富文本编辑器的同步方法sync,把富文本编辑器中的内容同步到textarea中。
6 商品添加功能实现
6.1 功能分析
1、请求的url
/item/save
2、返回结果,自定义。
TaotaoResult,参见参考资料。
6.2 Dao层
6.2.1 数据库
商品表、商品描述表。
分开的目的是为了提高查询效率。
6.2.2 Mapper文件
逆向工程生成的mapper可以使用。
6.3 Service层
功能分析:接收controller传递过来的对象一个是item一个是itemDesc对象。需要生成商品的id。把不为空的字段都补全。分别向两个表中插入数据。
参数:TbItem,TbItemDesc
返回值:TaotaoResult
1 @Override 2 3 public TaotaoResult addItem(TbItem item, TbItemDesc itemDesc) { 4 5 try { 6 7 //生成商品id 8 9 //可以使用redis的自增长key,在没有redis之前使用时间+随机数策略生成 10 11 Long itemId = IDUtils.genItemId(); 12 13 //补全不完整的字段 14 15 item.setId(itemId); 16 17 item.setStatus((byte) 1); 18 19 Date date = new Date(); 20 21 item.setCreated(date); 22 23 item.setUpdated(date); 24 25 //把数据插入到商品表 26 27 itemMapper.insert(item); 28 29 //添加商品描述 30 31 itemDesc.setItemId(itemId); 32 33 itemDesc.setCreated(date); 34 35 itemDesc.setUpdated(date); 36 37 //把数据插入到商品描述表 38 39 itemDescMapper.insert(itemDesc); 40 41 } catch (Exception e) { 42 43 e.printStackTrace(); 44 45 return TaotaoResult.build(500, ExceptionUtil.getStackTrace(e)); 46 47 } 48 49 return TaotaoResult.ok(); 50 51 } 52 |
6.4 Controller
功能分析:接收页面传递过来的数据包括商品和商品描述。
参数:TbItem、TbItemDesc。
返回值:TaotaoResult
1 @RequestMapping("/save") 2 3 @ResponseBody 4 5 public TaotaoResult addItem(TbItem item, String desc) { 6 7 TbItemDesc itemDesc = new TbItemDesc(); 8 9 itemDesc.setItemDesc(desc); 10 11 TaotaoResult result = itemService.addItem(item, itemDesc); 12 13 return result; 14 15 } 16 |