Flip Game
Time Limit: 1000MS | Memory Limit: 65536K | |
Total Submissions: 40424 | Accepted: 17556 |
Description
Flip game is played on a rectangular 4x4 field with two-sided pieces placed on each of its 16 squares. One side of each piece is white and the other one is black and each piece is lying either it's black or white side up. Each round you flip 3 to 5 pieces, thus changing the color of their upper side from black to white and vice versa. The pieces to be flipped are chosen every round according to the following rules:
Consider the following position as an example:
bwbw
wwww
bbwb
bwwb
Here "b" denotes pieces lying their black side up and "w" denotes pieces lying their white side up. If we choose to flip the 1st piece from the 3rd row (this choice is shown at the picture), then the field will become:
bwbw
bwww
wwwb
wwwb
The goal of the game is to flip either all pieces white side up or all pieces black side up. You are to write a program that will search for the minimum number of rounds needed to achieve this goal.
- Choose any one of the 16 pieces.
- Flip the chosen piece and also all adjacent pieces to the left, to the right, to the top, and to the bottom of the chosen piece (if there are any).
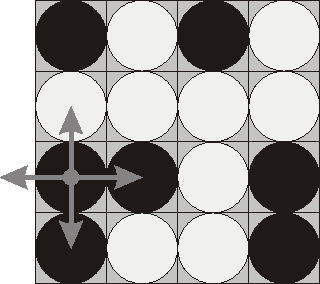
bwbw
wwww
bbwb
bwwb
Here "b" denotes pieces lying their black side up and "w" denotes pieces lying their white side up. If we choose to flip the 1st piece from the 3rd row (this choice is shown at the picture), then the field will become:
bwbw
bwww
wwwb
wwwb
The goal of the game is to flip either all pieces white side up or all pieces black side up. You are to write a program that will search for the minimum number of rounds needed to achieve this goal.
Input
The input consists of 4 lines with 4 characters "w" or "b" each that denote game field position.
Output
Write
to the output file a single integer number - the minimum number of
rounds needed to achieve the goal of the game from the given position.
If the goal is initially achieved, then write 0. If it's impossible to
achieve the goal, then write the word "Impossible" (without quotes).
Sample Input
bwwb bbwb bwwb bwww
Sample Output
4
Source
解析:状态压缩。因为棋子只有黑白两种颜色,对于某一个棋子来说,翻转奇数次等价于翻转1次,翻转偶数次等价于翻转0次。因此,可以等效的认为,每个棋子有翻转和不翻转2种状态,对于16个棋子而言,则有216种状态。我们可以把这个棋盘压缩成16位的空间,每一位可以有0、1两种状态。0代表白色,1代表黑色,通过异或操作进行翻转。枚举这216种状态,如果16位全变为0(等效的数为0)或全变为1(等效的数为65535)时,则表明棋子全为白色或黑色,在这个过程中,不断更新结果即可。如果这216种状态均不能使16位全变成0或全变成1,按照题意则输出"Impossible"。
//从上到下,从左到右,每个棋子翻转应该异或的值 void init() { int dir[4][2] = {{-1, 0}, {1, 0}, {0, -1}, {0, 1}}; for(int i = 0; i < 4; ++i){ for(int j = 0; j < 4; ++j){ int tmp = 0; tmp ^= 1<<(4*i+j); for(int k = 0; k < 4; ++k){ int x = i+dir[k][0]; int y = j+dir[k][1]; if(x<0 || x>3 || y<0 || y>3) continue; tmp ^= 1<<(4*x+y); } printf("%d ", tmp); } printf(" "); } }
预处理得到change[]。
#include <cstdio> #include <algorithm> using namespace std; const int INF = 0x7fffffff; char s[20]; int change[16] = {19,39,78,140,305,626,1252,2248,4880,8992,20032,35968,12544,29184,58368,51200}; int pos[16] = {1,2,4,8,16,32,64,128,256,512,1024,2048,4096,8192,16384,32768}; void solve() { int init_state = 0; for(int i = 0; i < 16; ++i){ if(s[i] == 'b') init_state += pos[i]; } int res = INF; for(int i = 0; i < 65536; ++i){ int cnt = 0; int state = init_state; for(int j = 0; j < 16; ++j){ if(i&pos[j]){ ++cnt; state ^= change[j]; } } if(state == 0 || state == 65535) res = min(res, cnt); } if(res == INF) printf("Impossible "); else printf("%d ", res); } int main() { for(int i = 0; i < 4; ++i) scanf("%s", &s[i*4]); solve(); return 0; }