效果图:
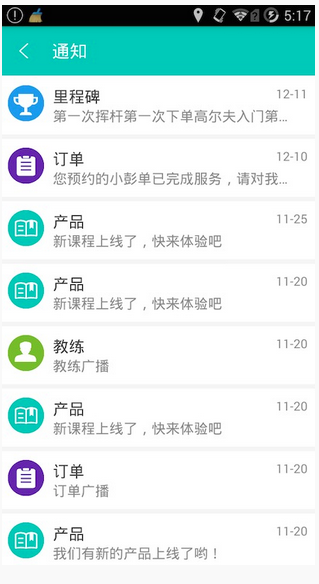
使用方法:
添加默认分割线:高度为2px,颜色为灰色
1 mRecyclerView.addItemDecoration(new RecyclerViewDivider(mContext, LinearLayoutManager.VERTICAL));
添加自定义分割线:可自定义分割线drawable
1 mRecyclerView.addItemDecoration(new RecyclerViewDivider(
2 mContext, LinearLayoutManager.VERTICAL, R.drawable.divider_mileage));
添加自定义分割线:可自定义分割线高度和颜色
1 mRecyclerView.addItemDecoration(new RecyclerViewDivider(
2 mContext, LinearLayoutManager.VERTICAL, 10, ContextCompat.getColor(mContext, R.color.divide_gray_color)));
万能分割线登场:
1 package utils;
2
3 import android.content.Context;
4 import android.content.res.TypedArray;
5 import android.graphics.Canvas;
6 import android.graphics.Paint;
7 import android.graphics.Rect;
8 import android.graphics.drawable.Drawable;
9 import android.support.v4.content.ContextCompat;
10 import android.support.v7.widget.LinearLayoutManager;
11 import android.support.v7.widget.RecyclerView;
12 import android.view.View;
13
14 /**
15 * Created by ${火龙裸先生} on 2016/10/29.
16 * 邮箱:791335000@qq.com
17 */
18 public class RecyclerViewDivider extends RecyclerView.ItemDecoration {
19 private Paint mPaint;
20 private Drawable mDivider;
21 private int mDividerHeight = 2;//分割线高度,默认为1px
22 private int mOrientation;//列表的方向:LinearLayoutManager.VERTICAL或LinearLayoutManager.HORIZONTAL
23 private static final int[] ATTRS = new int[]{android.R.attr.listDivider};
24
25 /**
26 * 默认分割线:高度为2px,颜色为灰色
27 *
28 * @param context
29 * @param orientation 列表方向
30 */
31 public RecyclerViewDivider(Context context, int orientation) {
32 if (orientation != LinearLayoutManager.VERTICAL && orientation != LinearLayoutManager.HORIZONTAL) {
33 throw new IllegalArgumentException("请输入正确的参数!");
34 }
35 mOrientation = orientation;
36
37 final TypedArray a = context.obtainStyledAttributes(ATTRS);
38 mDivider = a.getDrawable(0);
39 a.recycle();
40 }
41
42 /**
43 * 自定义分割线
44 *
45 * @param context
46 * @param orientation 列表方向
47 * @param drawableId 分割线图片
48 */
49 public RecyclerViewDivider(Context context, int orientation, int drawableId) {
50 this(context, orientation);
51 mDivider = ContextCompat.getDrawable(context, drawableId);
52 mDividerHeight = mDivider.getIntrinsicHeight();
53 }
54
55 /**
56 * 自定义分割线
57 *
58 * @param context
59 * @param orientation 列表方向
60 * @param dividerHeight 分割线高度
61 * @param dividerColor 分割线颜色
62 */
63 public RecyclerViewDivider(Context context, int orientation, int dividerHeight, int dividerColor) {
64 this(context, orientation);
65 mDividerHeight = dividerHeight;
66 mPaint = new Paint(Paint.ANTI_ALIAS_FLAG);
67 mPaint.setColor(dividerColor);
68 mPaint.setStyle(Paint.Style.FILL);
69 }
70
71
72 //获取分割线尺寸
73 @Override
74 public void getItemOffsets(Rect outRect, View view, RecyclerView parent, RecyclerView.State state) {
75 super.getItemOffsets(outRect, view, parent, state);
76 outRect.set(0, 0, 0, mDividerHeight);
77 }
78
79 //绘制分割线
80 @Override
81 public void onDraw(Canvas c, RecyclerView parent, RecyclerView.State state) {
82 super.onDraw(c, parent, state);
83 if (mOrientation == LinearLayoutManager.VERTICAL) {
84 drawVertical(c, parent);
85 } else {
86 drawHorizontal(c, parent);
87 }
88 }
89
90 //绘制横向 item 分割线
91 private void drawHorizontal(Canvas canvas, RecyclerView parent) {
92 final int left = parent.getPaddingLeft();
93 final int right = parent.getMeasuredWidth() - parent.getPaddingRight();
94 final int childSize = parent.getChildCount();
95 for (int i = 0; i < childSize; i++) {
96 final View child = parent.getChildAt(i);
97 RecyclerView.LayoutParams layoutParams = (RecyclerView.LayoutParams) child.getLayoutParams();
98 final int top = child.getBottom() + layoutParams.bottomMargin;
99 final int bottom = top + mDividerHeight;
100 if (mDivider != null) {
101 mDivider.setBounds(left, top, right, bottom);
102 mDivider.draw(canvas);
103 }
104 if (mPaint != null) {
105 canvas.drawRect(left, top, right, bottom, mPaint);
106 }
107 }
108 }
109
110 //绘制纵向 item 分割线
111 private void drawVertical(Canvas canvas, RecyclerView parent) {
112 final int top = parent.getPaddingTop();
113 final int bottom = parent.getMeasuredHeight() - parent.getPaddingBottom();
114 final int childSize = parent.getChildCount();
115 for (int i = 0; i < childSize; i++) {
116 final View child = parent.getChildAt(i);
117 RecyclerView.LayoutParams layoutParams = (RecyclerView.LayoutParams) child.getLayoutParams();
118 final int left = child.getRight() + layoutParams.rightMargin;
119 final int right = left + mDividerHeight;
120 if (mDivider != null) {
121 mDivider.setBounds(left, top, right, bottom);
122 mDivider.draw(canvas);
123 }
124 if (mPaint != null) {
125 canvas.drawRect(left, top, right, bottom, mPaint);
126 }
127 }
128 }
129
130 }