目录
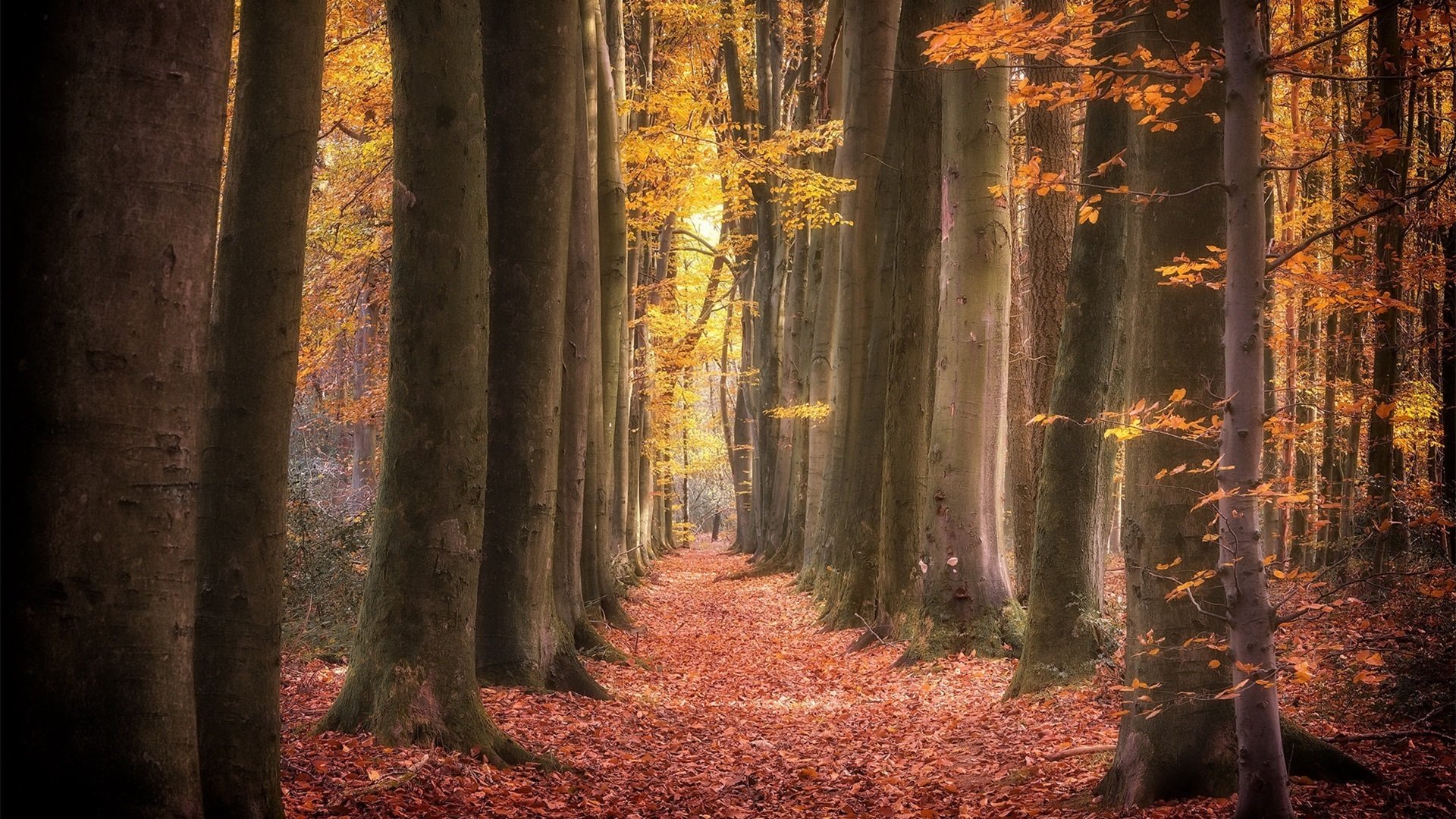
22、python生成随机数:uniform(), randint(), gauss(), expovariate()
22.1 模块:random
内建模块,伪随机数生成器
使用Mersenne Twister的伪随机数生成器PRNG进行生成,它以一个确定的数字作为属于,并为其生成一个随机数;为了安全起见,不要用PRNG生成随机数,要用secrets模块的真随机数TRNG生成;
22.2 播种随机数,即用随机数种子seed控制随机数
>>> import random
## 1、当不指定种子seed时,PRNG每次生成的数不一样
>>> print('Random Number 1=>',random.random())
Random Number 1=> 0.21008902332926982
>>> print('Random Number 2=>',random.random())
Random Number 2=> 0.434434837731393
## 2、当指定种子seed时,PRNG每次生成的数是一样的,所以称为伪随机数
>>> random.seed(42)
>>> print('Random Number 1=>',random.random())
Random Number 1=> 0.6394267984578837
>>> random.seed(42)
>>> print('Random Number 2=>',random.random())
Random Number 2=> 0.6394267984578837
22.3 在已知的范围内生成随机数,例如[2, 5],那就可以random.random()*3 + 2
, uniform(2,5), randint(2,5)
## 1、random.random()*3 + 2
>>> print('Random Number in range(2,8)=>', random.random()*6+2)
Random Number in range(2,8)=> 2.1500645313360014
## 2、uniform():获取开始值和结束值作为参数,返回一个浮点型的随机数
>>> print('Random Number in range(2,8)=>', random.uniform(2,8))
Random Number in range(2,8)=> 3.6501759102147155
## 3、randint():和uniform相似,不同的是返回值为一个整数
>>> print('Random Number in range(2,8)=>', random.randint(2,8))
Random Number in range(2,8)=> 3
22.4 从列表中随机选择一个值:choice(), choices()
## 1、choice会从这个列表中随机选择一个值
>>> a=[5,9,20,10,2,8]
>>> print('Randomly picked number=>',random.choice(a))
Randomly picked number=> 9
>>> print('Randomly picked number=>',random.choice(a))
Randomly picked number=> 8
>>> print('Randomly picked number=>',random.choice(a))
Randomly picked number=> 5
## 2、choices会从这个列表中随机选择多个值(随机数的数量可以超过列表程度)
>>> print('Randomly picked number=>',random.choices(a,k=3))
Randomly picked number=> [5, 20, 5]
>>> print('Randomly picked number=>',random.choices(a,k=3))
Randomly picked number=> [9, 10, 5]
>>> print('Randomly picked number=>',random.choices(a,k=3))
Randomly picked number=> [9, 10, 10]
## 3、choices利用weights将数组作为权重传递,增加每个值被选取的可能性
>>> print('Randomly picked number=>',random.choices(a,weights=[1,1,1,3,1,1],k=3))
Randomly picked number=> [5, 5, 2]
>>> print('Randomly picked number=>',random.choices(a,weights=[1,1,1,3,1,1],k=3))
Randomly picked number=> [10, 2, 10]
>>> print('Randomly picked number=>',random.choices(a,weights=[1,1,1,3,1,1],k=3))
Randomly picked number=> [10, 8, 10]
22.5 shuffling改组列表,对列表随机重排
>>> print('Original list=>',a)
Original list=> [5, 9, 20, 10, 2, 8]
>>> random.shuffle(a)
>>> print('Shuffled list=>',a)
Shuffled list=> [10, 5, 8, 9, 2, 20]
22.6 根据概率分布生成随机数:gauss(), expovariate()
(1)高斯分布gauss()
>>> import random
>>> import matplotlib.pyplot as plt
>>> temp = []
>>> for i in range(1000):
... temp.append(random.gauss(0,1))
...
>>> plt.hist(temp, bins=30)
>>> plt.show()
(2)变数分布expovariate():以lambda的值作为参数,lambda为正,则返回从0到正无穷的值;如果lambda为负,则返回从负无穷到0的值
>>> print('Random number from exponential distribution=>',random.expovariate(10))
Random number from exponential distribution=> 0.012164560954097013
>>> print('Random number from exponential distribution=>',random.expovariate(-1))
Random number from exponential distribution=> -0.6461397037921695
(3)伯努利分布
(4)均匀分布
(5)二项分布
(6)正太分布
(7)泊松分布
参考:https://www.analyticsvidhya.com/blog/2020/04/how-to-generate-random-numbers-in-python/?utm_source=feedburner&utm_medium=email&utm_campaign=Feed%3A+AnalyticsVidhya+(Analytics+Vidhya)
其他六种不同的概率分布:https://www.analyticsvidhya.com/blog/2017/09/6-probability-distributions-data-science/?utm_source=blog&utm_medium=how-to-generate-random-numbers-in-python