今天完成了一个日期类的小程序
在此附上代码和截图
以下是Date类代码
package a20200724;
public class Date {
String[] weekName= {"Sun","Mon","Tue","Wed","Thu","Fri","Sat"};
String[] monthName={"Jan","Feb","Mar","Api","May","Jun","Jul","Aug","Sep","Oct","Nov","Dec"};
private int year;
private int month;
private int day;
public int isLeapYear(){
if ((this.year % 4 == 0 && this.year % 100 != 0) || this.year % 400 == 0)return 1;
else return 0;
}
Date(int a,int b,int c){
if(b>12) {
this.month=12;
if(c>31) {
this.day=31;
}
else if(c<1) {
this.day=1;
}
else {
this.day=c;
}
}
else if(b<1) {
this.month=1;
if(c>31) {
this.day=31;
}
else if(c<1) {
this.day=1;
}
else {
this.day=c;
}
}
else {
if(b==2) {
if(a%4!=0&&c>28) {
this.day=28;
}
else if(a%4!=0&&c<1) {
this.day=1;
}
else if(a%4==0&&c>29) {
this.day=29;
}
else if(a%4==0&&c<1) {
this.day=1;
}else {
this.year=a;
this.month=2;
this.day=c;
}
}
else if(b==1||b==3||b==5||b==7||b==8||b==10||b==12) {
if(c<1) {
this.day=1;
this.year=a;
this.month=b;
}
else if(c>31) {
this.day=31;
this.year=a;
this.month=b;
}
else {
this.day=c;
this.month=b;
this.year=a;
}
}
else {
if(c<1) {
this.day=1;
this.year=a;
this.month=b;
}
else if(c>30) {
this.day=30;
this.year=a;
this.month=b;
}
else {
this.day=c;
this.month=b;
this.year=a;
}
}
}
System.out.println("Constructor run ");
}
Date(){
this.year=1;
this.day=1;
this.month=1;
System.out.println("Constructor run ");
}
Date(Date a){
this.year=a.year;
this.month=a.month;
this.day=a.day;
System.out.print("CopyConstructor run ");;
}
public void finalize() {
System.out.print("Destructor run ");
}
public void setYear(int a) {
this.year=a;
}
public void setMonth(int a) {
this.month=a;
if(this.month>12)
{
this.month=12;
}
if(this.month<1)
{
this.month=1;
}
}
public void setDay(int a) {
this.day=a;
if(this.day<1)
{
this.day=1;
}
if(this.day>31)
{
this.day=31;
}
if(this.month==2)
{
if(isLeapYear()==1)
{
if(this.day>30)
{
this.day=29;
}
}
else{
if(this.day>30)
{
this.day=28;
}
}
}
}
public int getYear() {
return this.year;
}
public int getMonth() {
return this.month;
}
public int getDay() {
return this.day;
}
public void tomorrow() {
if(this.day==31)
{
if(this.month==12){this.year++;this.month=1;this.day=1;}
else{this.month++;this.day=1;}
}
else if(this.day==30&&(this.month==4||this.month==6||this.month==9||this.month==11||this.month==8))
{
this.day=1;
this.month++;
}
else if(this.month==2)
{
if(isLeapYear()==1)
{
if(this.day==29)
{this.day=1;
this.month++;}
else{this.day++;}
}
else{
if(this.day==28)
{
this.day=1;
this.month++;
}
}
}
else{this.day++;}
}
public void yesterday() {
if(this.day==1){
if(this.month==1){
this.year--;
this.month=1;
this.day=31;
}
else if(this.month==2||this.month==4||this.month==6||this.month==9||this.month==11){
this.month--;
this.day=31;
}
else if(this.month==3)
{
this.month--;
if(isLeapYear()==1){this.day=29;}
else{this.day=28;}
}
else if(this.month==5||this.month==7||this.month==10||this.month==12){
this.month--;
this.day=30;
}
}
else{
this.day--;
}
}
public void chineseFormat() {
System.out.print(this.year+"年"+this.month+"月"+this.day+"日 ");
}
public void americaformat() {
System.out.print(monthName[this.month-1]+" "+this.day+","+this.year+" ");
}
public int nyear(int year) {
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0)
return 366;
else
return 365;
}
public int nmonth(int y,int m) {
if (m == 1 || m == 3 || m == 5 || m == 7 || m == 8 || m == 10 || m == 12)
return 31;
else if (nyear(y) == 366 && m == 2)
return 29;
else if (nyear(y) == 365 && m == 2)
return 28;
else
return 30;
}
public int GetDays(int year,int month) {
int i = 0;
int sum = 0;
if (year>1990)
for (i = 1990; i < year; i++)
sum += nyear(i);
if (month>1)
for (i = 1; i < month; i++)
{
sum += nmonth(year, i);
}
public class Date {
String[] weekName= {"Sun","Mon","Tue","Wed","Thu","Fri","Sat"};
String[] monthName={"Jan","Feb","Mar","Api","May","Jun","Jul","Aug","Sep","Oct","Nov","Dec"};
private int year;
private int month;
private int day;
public int isLeapYear(){
if ((this.year % 4 == 0 && this.year % 100 != 0) || this.year % 400 == 0)return 1;
else return 0;
}
Date(int a,int b,int c){
if(b>12) {
this.month=12;
if(c>31) {
this.day=31;
}
else if(c<1) {
this.day=1;
}
else {
this.day=c;
}
}
else if(b<1) {
this.month=1;
if(c>31) {
this.day=31;
}
else if(c<1) {
this.day=1;
}
else {
this.day=c;
}
}
else {
if(b==2) {
if(a%4!=0&&c>28) {
this.day=28;
}
else if(a%4!=0&&c<1) {
this.day=1;
}
else if(a%4==0&&c>29) {
this.day=29;
}
else if(a%4==0&&c<1) {
this.day=1;
}else {
this.year=a;
this.month=2;
this.day=c;
}
}
else if(b==1||b==3||b==5||b==7||b==8||b==10||b==12) {
if(c<1) {
this.day=1;
this.year=a;
this.month=b;
}
else if(c>31) {
this.day=31;
this.year=a;
this.month=b;
}
else {
this.day=c;
this.month=b;
this.year=a;
}
}
else {
if(c<1) {
this.day=1;
this.year=a;
this.month=b;
}
else if(c>30) {
this.day=30;
this.year=a;
this.month=b;
}
else {
this.day=c;
this.month=b;
this.year=a;
}
}
}
System.out.println("Constructor run ");
}
Date(){
this.year=1;
this.day=1;
this.month=1;
System.out.println("Constructor run ");
}
Date(Date a){
this.year=a.year;
this.month=a.month;
this.day=a.day;
System.out.print("CopyConstructor run ");;
}
public void finalize() {
System.out.print("Destructor run ");
}
public void setYear(int a) {
this.year=a;
}
public void setMonth(int a) {
this.month=a;
if(this.month>12)
{
this.month=12;
}
if(this.month<1)
{
this.month=1;
}
}
public void setDay(int a) {
this.day=a;
if(this.day<1)
{
this.day=1;
}
if(this.day>31)
{
this.day=31;
}
if(this.month==2)
{
if(isLeapYear()==1)
{
if(this.day>30)
{
this.day=29;
}
}
else{
if(this.day>30)
{
this.day=28;
}
}
}
}
public int getYear() {
return this.year;
}
public int getMonth() {
return this.month;
}
public int getDay() {
return this.day;
}
public void tomorrow() {
if(this.day==31)
{
if(this.month==12){this.year++;this.month=1;this.day=1;}
else{this.month++;this.day=1;}
}
else if(this.day==30&&(this.month==4||this.month==6||this.month==9||this.month==11||this.month==8))
{
this.day=1;
this.month++;
}
else if(this.month==2)
{
if(isLeapYear()==1)
{
if(this.day==29)
{this.day=1;
this.month++;}
else{this.day++;}
}
else{
if(this.day==28)
{
this.day=1;
this.month++;
}
}
}
else{this.day++;}
}
public void yesterday() {
if(this.day==1){
if(this.month==1){
this.year--;
this.month=1;
this.day=31;
}
else if(this.month==2||this.month==4||this.month==6||this.month==9||this.month==11){
this.month--;
this.day=31;
}
else if(this.month==3)
{
this.month--;
if(isLeapYear()==1){this.day=29;}
else{this.day=28;}
}
else if(this.month==5||this.month==7||this.month==10||this.month==12){
this.month--;
this.day=30;
}
}
else{
this.day--;
}
}
public void chineseFormat() {
System.out.print(this.year+"年"+this.month+"月"+this.day+"日 ");
}
public void americaformat() {
System.out.print(monthName[this.month-1]+" "+this.day+","+this.year+" ");
}
public int nyear(int year) {
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0)
return 366;
else
return 365;
}
public int nmonth(int y,int m) {
if (m == 1 || m == 3 || m == 5 || m == 7 || m == 8 || m == 10 || m == 12)
return 31;
else if (nyear(y) == 366 && m == 2)
return 29;
else if (nyear(y) == 365 && m == 2)
return 28;
else
return 30;
}
public int GetDays(int year,int month) {
int i = 0;
int sum = 0;
if (year>1990)
for (i = 1990; i < year; i++)
sum += nyear(i);
if (month>1)
for (i = 1; i < month; i++)
{
sum += nmonth(year, i);
}
return sum;
}
public void printMonthCalendar() {
int i, j, sum, daycount;
while (this.year+this.month!=0)
{
sum = GetDays(this.year, this.month);
day = (sum + 1) % 7; //得出一个月第一行前要空几格
daycount = nmonth(this.year, this.month); //算出这一个月的天数
System.out.print(weekName[0]+weekName[1]+weekName[2]+weekName[3]+weekName[4]+weekName[5]+weekName[6]+" ");
for (i = 0; i < day; i++)
{
System.out.print(" ");
}
for (i = 1, j = day + i; i <= daycount; i++, j++)
{
System.out.printf("%3d",i);
if (j % 7 == 0)
System.out.print(" ");
}
System.out.print(" ");
year=0;
month=0;
}
}
int weekDay(){
int a,b,c;
a=GetDays(this.year,this.month);
b=a+this.day-1;
c=b%7;
return c;
}
}
}
public void printMonthCalendar() {
int i, j, sum, daycount;
while (this.year+this.month!=0)
{
sum = GetDays(this.year, this.month);
day = (sum + 1) % 7; //得出一个月第一行前要空几格
daycount = nmonth(this.year, this.month); //算出这一个月的天数
System.out.print(weekName[0]+weekName[1]+weekName[2]+weekName[3]+weekName[4]+weekName[5]+weekName[6]+" ");
for (i = 0; i < day; i++)
{
System.out.print(" ");
}
for (i = 1, j = day + i; i <= daycount; i++, j++)
{
System.out.printf("%3d",i);
if (j % 7 == 0)
System.out.print(" ");
}
System.out.print(" ");
year=0;
month=0;
}
}
int weekDay(){
int a,b,c;
a=GetDays(this.year,this.month);
b=a+this.day-1;
c=b%7;
return c;
}
}
以下是主函数代码
package a20200724;
import java.util.Scanner;
public class main {
public static void main(String[] arg) {
int year,month,day;
Date d1=new Date();
Date d2=new Date(d1);
Scanner y = new Scanner(System.in);
Scanner m = new Scanner(System.in);
Scanner d = new Scanner(System.in);
year = y.nextInt();
month = m.nextInt();
day = d.nextInt();
d1.setYear(year);
d1.setMonth(month);
d1.setDay(day);
d1.yesterday();
d1.chineseFormat();
Scanner y1 = new Scanner(System.in);
Scanner m1 = new Scanner(System.in);
Scanner D1 = new Scanner(System.in);
year = y1.nextInt();
month = m1.nextInt();
day = D1.nextInt();
d2.setYear(year);
d2.setMonth(month);
d2.setDay(day);
d2.tomorrow();
d2.americaformat();
d2.printMonthCalendar();
d1=null;
d2=null;
System.gc();
}
}
import java.util.Scanner;
public class main {
public static void main(String[] arg) {
int year,month,day;
Date d1=new Date();
Date d2=new Date(d1);
Scanner y = new Scanner(System.in);
Scanner m = new Scanner(System.in);
Scanner d = new Scanner(System.in);
year = y.nextInt();
month = m.nextInt();
day = d.nextInt();
d1.setYear(year);
d1.setMonth(month);
d1.setDay(day);
d1.yesterday();
d1.chineseFormat();
Scanner y1 = new Scanner(System.in);
Scanner m1 = new Scanner(System.in);
Scanner D1 = new Scanner(System.in);
year = y1.nextInt();
month = m1.nextInt();
day = D1.nextInt();
d2.setYear(year);
d2.setMonth(month);
d2.setDay(day);
d2.tomorrow();
d2.americaformat();
d2.printMonthCalendar();
d1=null;
d2=null;
System.gc();
}
}
以下是运行结果截图
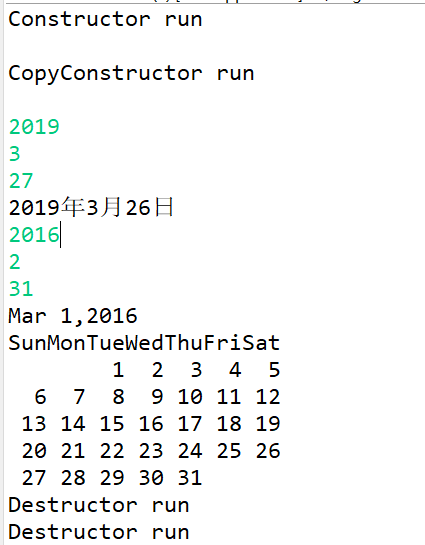