Hongcow is learning to spell! One day, his teacher gives him a word that he needs to learn to spell. Being a dutiful student, he immediately learns how to spell the word.
Hongcow has decided to try to make new words from this one. He starts by taking the word he just learned how to spell, and moves the last character of the word to the beginning of the word. He calls this a cyclic shift. He can apply cyclic shift many times. For example, consecutively applying cyclic shift operation to the word "abracadabra" Hongcow will get words "aabracadabr", "raabracadab" and so on.
Hongcow is now wondering how many distinct words he can generate by doing the cyclic shift arbitrarily many times. The initial string is also counted.
The first line of input will be a single string s (1 ≤ |s| ≤ 50), the word Hongcow initially learns how to spell. The string s consists only of lowercase English letters ('a'–'z').
Output a single integer equal to the number of distinct strings that Hongcow can obtain by applying the cyclic shift arbitrarily many times to the given string.
abcd
4
bbb
1
yzyz
2
For the first sample, the strings Hongcow can generate are "abcd", "dabc", "cdab", and "bcda".
For the second sample, no matter how many times Hongcow does the cyclic shift, Hongcow can only generate "bbb".
For the third sample, the two strings Hongcow can generate are "yzyz" and "zyzy".
题意:给你一个字符串 每次可以把最尾部的一个字符放到最前面 问形成的字符串有多少种?
题解:模拟
1 #include <iostream> 2 #include <cstdio> 3 #include <cstdlib> 4 #include <cstring> 5 #include <algorithm> 6 #include <stack> 7 #include <queue> 8 #include <cmath> 9 #include <map> 10 #define ll __int64 11 #define mod 1000000007 12 #define dazhi 2147483647 13 #define bug() printf("!!!!!!!") 14 #define M 100005 15 using namespace std; 16 char s[55]; 17 map<string,int>mp; 18 int main() 19 { 20 scanf("%s",s); 21 string str; 22 str.assign(s); 23 mp[s]=1; 24 int ans=1; 25 int len=strlen(s); 26 int last=len-1; 27 len--; 28 while(len) 29 { 30 len--; 31 char exm=s[last]; 32 for(int i=last;i>=1;i--) 33 { 34 s[i]=s[i-1]; 35 } 36 s[0]=exm; 37 str.assign(s); 38 if(mp[str]==0) 39 { 40 mp[str]++; 41 ans++; 42 } 43 } 44 cout<<ans<<endl; 45 return 0; 46 }
Hongcow likes solving puzzles.
One day, Hongcow finds two identical puzzle pieces, with the instructions "make a rectangle" next to them. The pieces can be described by an n by m grid of characters, where the character 'X' denotes a part of the puzzle and '.' denotes an empty part of the grid. It is guaranteed that the puzzle pieces are one 4-connected piece. See the input format and samples for the exact details on how a jigsaw piece will be specified.
The puzzle pieces are very heavy, so Hongcow cannot rotate or flip the puzzle pieces. However, he is allowed to move them in any directions. The puzzle pieces also cannot overlap.
You are given as input the description of one of the pieces. Determine if it is possible to make a rectangle from two identical copies of the given input. The rectangle should be solid, i.e. there should be no empty holes inside it or on its border. Keep in mind that Hongcow is not allowed to flip or rotate pieces and they cannot overlap, i.e. no two 'X' from different pieces can share the same position.
The first line of input will contain two integers n and m (1 ≤ n, m ≤ 500), the dimensions of the puzzle piece.
The next n lines will describe the jigsaw piece. Each line will have length m and will consist of characters '.' and 'X' only. 'X' corresponds to a part of the puzzle piece, '.' is an empty space.
It is guaranteed there is at least one 'X' character in the input and that the 'X' characters form a 4-connected region.
Output "YES" if it is possible for Hongcow to make a rectangle. Output "NO" otherwise.
2 3
XXX
XXX
YES
2 2
.X
XX
NO
5 5
.....
..X..
.....
.....
.....
YES
For the first sample, one example of a rectangle we can form is as follows
111222
111222
For the second sample, it is impossible to put two of those pieces without rotating or flipping to form a rectangle.
In the third sample, we can shift the first tile by one to the right, and then compose the following rectangle:
.....
..XX.
.....
.....
.....
题意:给你一个n*m的矩阵 由‘X’形成一个图形 问你是否可以用两个相同的图形拼成一个矩形(不可以旋转和翻折)
题解:确定给你的图形是否为一个矩形即可
1 #include <iostream> 2 #include <cstdio> 3 #include <cstdlib> 4 #include <cstring> 5 #include <algorithm> 6 #include <stack> 7 #include <queue> 8 #include <cmath> 9 #include <map> 10 #define ll __int64 11 #define mod 1000000007 12 #define dazhi 2147483647 13 #define bug() printf("!!!!!!!") 14 #define M 100005 15 using namespace std; 16 int n,m; 17 char a[505][505]; 18 int main() 19 { 20 scanf("%d %d",&n,&m); 21 getchar(); 22 for(int i=0;i<n;i++) 23 scanf("%s",a[i]); 24 int l=m-1,r=0,u=n-1,d=0; 25 for(int i=0;i<n;i++) 26 { 27 for(int j=0;j<m;j++) 28 { 29 if(a[i][j]=='X') 30 { 31 l=min(l,j); 32 r=max(r,j); 33 u=min(u,i); 34 d=max(d,i); 35 } 36 } 37 } 38 for(int i=u;i<=d;i++) 39 { 40 for(int j=l;j<=r;j++) 41 { 42 if(a[i][j]=='.') 43 { 44 printf("NO "); 45 return 0; 46 } 47 } 48 } 49 printf("YES "); 50 return 0; 51 }
Hongcow is ruler of the world. As ruler of the world, he wants to make it easier for people to travel by road within their own countries.
The world can be modeled as an undirected graph with n nodes and m edges. k of the nodes are home to the governments of the k countries that make up the world.
There is at most one edge connecting any two nodes and no edge connects a node to itself. Furthermore, for any two nodes corresponding to governments, there is no path between those two nodes. Any graph that satisfies all of these conditions is stable.
Hongcow wants to add as many edges as possible to the graph while keeping it stable. Determine the maximum number of edges Hongcow can add.
The first line of input will contain three integers n, m and k (1 ≤ n ≤ 1 000, 0 ≤ m ≤ 100 000, 1 ≤ k ≤ n) — the number of vertices and edges in the graph, and the number of vertices that are homes of the government.
The next line of input will contain k integers c1, c2, ..., ck (1 ≤ ci ≤ n). These integers will be pairwise distinct and denote the nodes that are home to the governments in this world.
The following m lines of input will contain two integers ui and vi (1 ≤ ui, vi ≤ n). This denotes an undirected edge between nodes ui and vi.
It is guaranteed that the graph described by the input is stable.
Output a single integer, the maximum number of edges Hongcow can add to the graph while keeping it stable.
4 1 2
1 3
1 2
2
3 3 1
2
1 2
1 3
2 3
0
For the first sample test, the graph looks like this:
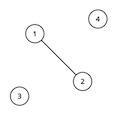
For the second sample test, the graph looks like this:
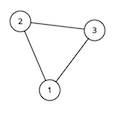
1 #include <iostream> 2 #include <cstdio> 3 #include <cstdlib> 4 #include <cstring> 5 #include <algorithm> 6 #include <stack> 7 #include <queue> 8 #include <cmath> 9 #include <map> 10 #define ll __int64 11 #define mod 1000000007 12 #define dazhi 2147483647 13 #define bug() printf("!!!!!!!") 14 #define M 100005 15 using namespace std; 16 int n,m,k; 17 int a[1005]; 18 int fa[1005]; 19 int l,r; 20 map<int,int>mp; 21 map<int,int>mpp; 22 int find(int root) 23 { 24 if(root!=fa[root]) 25 return fa[root]=find(fa[root]); 26 else 27 return root; 28 } 29 void unio(int a,int b) 30 { 31 int aa=find(a); 32 int bb=find(b); 33 if(aa!=bb) 34 { 35 fa[aa]=bb; 36 } 37 } 38 int main() 39 { 40 scanf("%d %d %d",&n,&m,&k); 41 for(int i=1;i<=k;i++) 42 scanf("%d",&a[i]); 43 for(int i=1;i<=n;i++) 44 fa[i]=i; 45 for(int i=1;i<=m;i++) 46 { 47 scanf("%d %d",&l,&r); 48 unio(l,r); 49 } 50 for(int i=1;i<=n;i++) 51 { 52 int x=find(i); 53 mp[fa[i]]++; 54 } 55 int maxn=0; 56 int sum=0; 57 int re=0; 58 for(int i=1;i<=k;i++) 59 { 60 maxn=max(maxn,mp[fa[a[i]]]); 61 sum+=mp[fa[a[i]]]; 62 mpp[fa[a[i]]]=1; 63 re=re+ mp[fa[a[i]]]*(mp[fa[a[i]]]-1)/2; 64 } 65 int b[1005]; 66 int jishu=0; 67 int ans=0; 68 for(int i=1;i<=n;i++) 69 { 70 if(mpp[fa[i]]==0) 71 { 72 b[jishu++]=mp[fa[i]]; 73 re=re+mp[fa[i]]*(mp[fa[i]]-1)/2; 74 mpp[fa[i]]=1; 75 } 76 } 77 for(int i=0;i<jishu;i++) 78 { 79 for(int j=i+1;j<jishu;j++) 80 { 81 ans+=(b[i]*b[j]); 82 } 83 } 84 printf("%d ",re-m+(n-sum)*maxn+ans); 85 return 0; 86 }