A. Vanya and Cubes
time limit per test
1 secondmemory limit per test
256 megabytesinput
standard inputoutput
standard outputVanya got n cubes. He decided to build a pyramid from them. Vanya wants to build the pyramid as follows: the top level of the pyramid must consist of 1 cube, the second level must consist of 1 + 2 = 3 cubes, the third level must have 1 + 2 + 3 = 6 cubes, and so on. Thus, the i-th level of the pyramid must have 1 + 2 + ... + (i - 1) + i cubes.
Vanya wants to know what is the maximum height of the pyramid that he can make using the given cubes.
Input
The first line contains integer n (1 ≤ n ≤ 104) — the number of cubes given to Vanya.
Output
Print the maximum possible height of the pyramid in the single line.
Examples
input
1
output
1
input
25
output
4
Note
Illustration to the second sample:
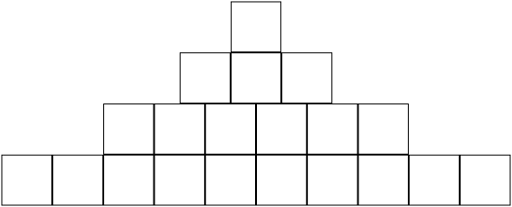
题意:给你n个方块 按照如图的方式摆放 问最多能摆放多少层?
题解:暴力层数 判断需要多少方块
1 /****************************** 2 code by drizzle 3 blog: www.cnblogs.com/hsd-/ 4 ^ ^ ^ ^ 5 O O 6 ******************************/ 7 #include<bits/stdc++.h> 8 #include<iostream> 9 #include<cstring> 10 #include<cstdio> 11 #include<map> 12 #include<algorithm> 13 #include<queue> 14 #define ll __int64 15 using namespace std; 16 int n; 17 int a[10004]; 18 int main() 19 { 20 scanf("%d",&n); 21 int sum=0; 22 for(int i=1;i<=50;i++) 23 { 24 sum=sum+i*(i+1)/2; 25 if(sum==n) 26 { 27 cout<<i<<endl; 28 return 0; 29 } 30 if(sum>n) 31 { 32 cout<<i-1<<endl; 33 return 0; 34 } 35 } 36 return 0; 37 }