Flip Game
Time Limit: 1000MS | Memory Limit: 65536K | |
Total Submissions: 46805 | Accepted: 20034 |
Description
Flip game is played on a rectangular 4x4 field with two-sided pieces placed on each of its 16 squares. One side of each piece is white and the other one is black and each piece is lying either it's black or white side up. Each round you flip 3 to 5 pieces,
thus changing the color of their upper side from black to white and vice versa. The pieces to be flipped are chosen every round according to the following rules:
Consider the following position as an example:
bwbw
wwww
bbwb
bwwb
Here "b" denotes pieces lying their black side up and "w" denotes pieces lying their white side up. If we choose to flip the 1st piece from the 3rd row (this choice is shown at the picture), then the field will become:
bwbw
bwww
wwwb
wwwb
The goal of the game is to flip either all pieces white side up or all pieces black side up. You are to write a program that will search for the minimum number of rounds needed to achieve this goal.
- Choose any one of the 16 pieces.
- Flip the chosen piece and also all adjacent pieces to the left, to the right, to the top, and to the bottom of the chosen piece (if there are any).
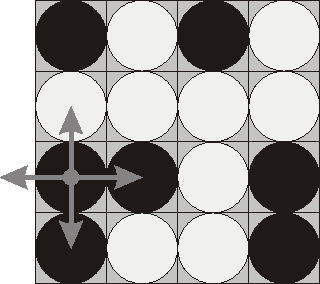
bwbw
wwww
bbwb
bwwb
Here "b" denotes pieces lying their black side up and "w" denotes pieces lying their white side up. If we choose to flip the 1st piece from the 3rd row (this choice is shown at the picture), then the field will become:
bwbw
bwww
wwwb
wwwb
The goal of the game is to flip either all pieces white side up or all pieces black side up. You are to write a program that will search for the minimum number of rounds needed to achieve this goal.
Input
The input consists of 4 lines with 4 characters "w" or "b" each that denote game field position.
Output
Write to the output file a single integer number - the minimum number of rounds needed to achieve the goal of the game from the given position. If the goal is initially achieved, then write 0. If it's impossible to achieve the goal, then write the word "Impossible"
(without quotes).
Sample Input
bwwb bbwb bwwb bwww
Sample Output
4
题意:给你一个4*4的棋盘,上面摆了16个非黑即白的棋子,每次可以翻一个棋子,而且每次翻的时候连着四周的棋子一起翻,问最少几次操作可以使棋盘全变为白棋或者黑棋
思路:
okokok
解法一:dfs+枚举 #include<stdio.h> #include<string.h> #include<string> #include<iostream> #include<cmath> #include<algorithm> #include<set> #include<map> using namespace std; bool m_map[6][6],flag; //这个m_map大小主要看棋盘是从(0,0)开始,还是(1,1)开始 int step; int isok()//判断棋子是否全为白色或者黑色 { int i,j; for(i=0; i<4; i++) { for(j=0; j<4; j++) if(m_map[i][j]!=m_map[0][0])return 0; } return 1; } void flip(int x,int y)//翻转棋子的操作 { m_map[x][y]=!m_map[x][y]; if(y<=2)m_map[x][y+1]=!m_map[x][y+1]; if(y!=0)m_map[x][y-1]=!m_map[x][y-1]; if(x<=2)m_map[x+1][y]=!m_map[x+1][y]; if(x!=0)m_map[x-1][y]=!m_map[x-1][y]; } void dfs(int x,int y,int dep) { if(step==dep) { if(isok())flag=true; return ; } if(flag||x==4) return; flip(x,y); if(y<3)dfs(x,y+1,dep+1); else dfs(x+1,0,dep+1); flip(x,y); if(y<3)dfs(x,y+1,dep); else dfs(x+1,0,dep); //return ;这个return可要可不要,要的话应该快一点 } //注意:这个题只有一个测试,不要求多次测试 int main() { int i,j,k; char ch; flag=false; //step=0; for(i=0; i<4; i++)//初始化 for(j=0; j<4; j++) { m_map[i][j]=false; } //初始化其实也可以bool m_map[6][6]={false}; //或者 memset(used,false,sizeof(used)); for(i=0; i<4; i++)//输入 { for(j=0; j<4; j++) { scanf("%c",&ch); if(ch=='b')m_map[i][j]=true; } getchar(); } for(step=0; step<=16; step++)//step是个全局变量,当完成步数等于当前step时,程序就推出; { dfs(0,0,0); if(flag)break; } if(flag)printf("%d ",step);//如果flag为true,那么打印步数,否则输出不可能 else printf("Impossible "); return 0; } /* bwbw wwww bbwb bwwb Impossible bwwb bbwb bwwb bwww 4 wwww wwww wwww wwww 0 bbbb bbbb bbbb bbbb 0 bbbb bwbb bbbb bbbb Impossible bwbb bwbb bwbb bbbb Impossible bwbb wwwb bwbb bbbb 1 wwww wwwb wwbb wwwb 1 wwww wwww wwwb wwbb 1 wbwb bwbw wbwb bwbw Impossible bbbb bwwb bwwb bbbb 4 bwwb wbbw wbbw bwwb 4 bbww bbww wwbb wwbb Impossible bbwb bbbw wwbb wwwb Impossible wwwb wwbw wbww wwbw Impossible bbbb wwww wwbb wbbb Impossible bwwb wbwb wbbb wbbb 4 bwbb bwbb bwbw bbbw 5 wbwb bbbb bbww wbbb 6 bbwb bbbb wbwb bbbb 5 */
解法2:bfs+状态压缩
这个会用到位运算,先复习下位运算的知识:
id &= ~(1<<x) :将id右起第x位置置为0。 //多加一个翻转
id |= (1<<x) :将id右起第x位置置为1。
id ^=(1<<x) :将id右起第x位置置翻转,1翻转为0,或0翻转为1
棋盘一共是有16方格,也就意味着有2^16(65535)种状态,
我们就假设黑色的棋子的状态为0,白色状态的棋子为1
每种方格只能被点击一次,比如我们在一个全为黑色点击了左上角的棋子,棋盘如下:
bbbb
bbbb
bbbb
bbbb
抽象为对应的状态棋盘就变为
0000
0000
0000
0000
点击左上角的棋子之后,状态棋盘就变为:
1100
1000
0000
0000
我们默认上面的和左边的高位,所以对应的二进制转换为十进制就变为51200
当点击其他的时候也是一样的原因。
有了这16中状态我们就可以通过异或来得到点击完相应的棋子之后的状态。
int change1[16]= { 51200,58368,29184,12544, 35968,20032,10016,4880, 2248,1252,626,305, 140,78,39,19 };//里面的数字与当前state进行异或运算后得到的状态就是翻转后的状态,例如state^=51200,那么运算后state视为对1号位的棋子进行了翻转后的状态(棋子是0-15号)
或者换一种方法对棋盘进行flip(翻转)操作:
x是翻转前的状态,(i,j)表示要操作的位置
int change(unsigned int x,int i,int j) { int k; k=(1<<(i*4+j));//i是行,j是列,那么i*4+j就是当前棋子的号数(0-15)号,k其实就是上面那种方法中的数字 x^=k;//自身翻转 if(i-1>=0) { k=(1<<(4*(i-1)+j));//对应棋盘进行理解,i-1就是上一行,故(i-1)*4+j就是该位置上面棋子的号数 x^=k; } if(i+1<4) { k=(1<<(4*(i+1)+j));//对该棋子下面的棋子进行翻转 x^=k; } if(j+1<4) { k=(1<<(4*i+j+1));//对该棋子右面的棋子进行翻转 x^=k; } if(j-1>=0) { k=(1<<(4*i+j-1));//对该棋子左面的棋子进行翻转 x^=k; } return x;//返回翻转后的状态 }
#include<stdio.h> #include<string.h> #include<string> #include<iostream> #include<algorithm> #include<cmath> #include<map> #include<set> #include<queue> using namespace std; int vis[65537]; queue<int>que; int change1[16]= { 51200,58368,29184,12544, 35968,20032,10016,4880, 2248,1252,626,305, 140,78,39,19 };//里面的数字与当前state进行异或运算后得到的状态就是翻转后的状态,例如state^=51200,那么运算后state视为对1号位的棋子进行了翻转后的状态(棋子是0-15号)int state;int bfs(){ while(!que.empty())que.pop(); int i,j,k,step=0,t; int cur,new_state,temp; memset(vis,0,sizeof(vis)); que.push(state); vis[state]=1; while(!que.empty()) { t=que.size(); for(j=0; j<t; j++)//为什么要进行t次循环呢//因为我们没有用结构体来储存数据,那么也就是说当前状态和当前已操作次数没有关联,这样就不好达到确定现在的状态究竟已经操作了多少次//为了解决这个问题,我们每次将que当前元素使用完,这些元素使用完后,步数+1,那么新加进来的状态的步数就已知了 { cur=que.front(); que.pop(); if((cur==0)||(cur==0xffff))//如果当前状态就是全黑或全白 { //printf("*%d* ",step); return step; } for(i=0; i<16; i++)//为什么是16次 ?现在你得到一个棋盘,那么就有16个操作可能对吧,对每个棋子都进行操作一下 { new_state=(cur^change1[i]);//这步就是翻转操作,状态压缩+位运算,是不是很简洁,直接将二维的平面操作换成了一步计算 if(!vis[new_state])//如果这个状态没有有其他操作到达 { que.push(new_state); vis[new_state]=1; } } } step++;//步数加一 } return -1;//如果没有从循环里的出口出来,那么说明没法使棋子全变成白色或者黑色}void init(){ int i,j,k; char s[6]; state=0; for(i=0; i<4; i++)//我们以左边和上边为二进制高位,右下角为二进制的低位 { scanf("%s",s); for(j=0; j<4; j++) { if(s[j]=='b')state=(state<<1)+1;//以黑为1,白为0 else state=(state<<1); } } // printf("*%d* ",state);}int main(){ int tmp; init(); tmp=bfs(); if(tmp==-1)printf("Impossible "); else printf("%d ",tmp);}/*bwbwwwwwbbwbbwwbbwwbbbwbbwwbbwwwwwwwwwwwwwwwwwwwbwbbwwwbbwbbbbbb*/