Description
There are n children in Jzzhu's school. Jzzhu is going to give some candies to them. Let's number all the children from 1 to n. The i-th child wants to get at least ai candies.
Jzzhu asks children to line up. Initially, the i-th child stands at the i-th place of the line. Then Jzzhu start distribution of the candies. He follows the algorithm:
- Give m candies to the first child of the line.
- If this child still haven't got enough candies, then the child goes to the end of the line, else the child go home.
- Repeat the first two steps while the line is not empty.
Consider all the children in the order they go home. Jzzhu wants to know, which child will be the last in this order?
Input
The first line contains two integers n, m(1 ≤ n ≤ 100; 1 ≤ m ≤ 100). The second line contains n integers a1, a2, ..., an(1 ≤ ai ≤ 100).
Output
Output a single integer, representing the number of the last child.
Sample Input
5 2 1 3 1 4 2
4
6 4 1 1 2 2 3 3
6
Hint
Let's consider the first sample.
Firstly child 1 gets 2 candies and go home. Then child 2 gets 2 candies and go to the end of the line. Currently the line looks like [3, 4, 5, 2] (indices of the children in order of the line). Then child 3 gets 2 candies and go home, and then child 4 gets 2 candies and goes to the end of the line. Currently the line looks like [5, 2, 4]. Then child 5 gets 2 candies and goes home. Then child 2 gets two candies and goes home, and finally child 4 gets 2 candies and goes home.
Child 4 is the last one who goes home.
题意:给出n个人,每个人需要ai颗糖站成一列,每次给列首发m颗糖,如果够就回家,如果不够就回到队尾继续排队。
#include <iostream> #include <cstdio> #include <cstring> #include <queue> using namespace std; #define INF 0x3f3f3f3f #define N 110 struct Node { int id,need; }; int n,m; int main() { while(scanf("%d%d",&n,&m)!=EOF) { Node t; queue<Node> q; for(int i=1;i<=n;i++) { t.id=i; scanf("%d",&t.need); q.push(t); } while(q.size()>1) { t=q.front(); q.pop(); if(t.need>m) { t.need-=m; q.push(t); } } printf("%d ",q.front().id); } return 0; }
Description
Jzzhu has invented a kind of sequences, they meet the following property:

You are given x and y, please calculate fn modulo 1000000007(109 + 7).
Input
The first line contains two integers x and y(|x|, |y| ≤ 109). The second line contains a single integer n(1 ≤ n ≤ 2·109).
Output
Output a single integer representing fn modulo 1000000007(109 + 7).
Sample Input
2 3 3
1
0 -1 2
1000000006
Hint
In the first sample, f2 = f1 + f3, 3 = 2 + f3, f3 = 1.
In the second sample, f2 = - 1; - 1 modulo (109 + 7) equals (109 + 6).
题意:Fi=Fi-1+Fi-2,求Fn。
#include <iostream> #include <cstdio> #include <cstring> using namespace std; #define ll long long #define MOD 1000000007 #define N 2 struct Matric { ll size; ll a[N][N]; Matric(ll s=0) { size=s; memset(a,0,sizeof(a)); } Matric operator * (const Matric &t) { Matric res=Matric(size); for(ll i=0;i<size;i++) { for(ll k=0;k<size;k++) { if((*this).a[i][k]) for(ll j=0;j<size;j++) { res.a[i][j]=((res.a[i][j]+(*this).a[i][k]*t.a[k][j])%MOD+MOD)%MOD; //if(res.a[i][j]>=MOD) res.a[i][j]%=MOD; } } } return res; } Matric operator ^ (ll n) { Matric ans=Matric(size); for(ll i=0;i<size;i++) ans.a[i][i]=1; while(n) { if(n&1) ans=ans*(*this); (*this)=(*this)*(*this); n>>=1; } return ans; } void debug() { for(ll i=0;i<size;i++) { for(ll j=0;j<size;j++) { printf("%d ",a[i][j]); } printf(" "); } } }; int main() { ll n,x,y; while(scanf("%lld%lld%lld",&x,&y,&n)!=EOF) { n--; Matric a=Matric(2); Matric b=Matric(2); a.a[0][0]=x;a.a[0][1]=y; b.a[0][1]=-1;b.a[1][0]=1;b.a[1][1]=1; b=b^n; a=a*b; printf("%lld ",a.a[0][0]); } return 0; }
Description
Jzzhu has a big rectangular chocolate bar that consists of n × m unit squares. He wants to cut this bar exactly k times. Each cut must meet the following requirements:
- each cut should be straight (horizontal or vertical);
- each cut should go along edges of unit squares (it is prohibited to divide any unit chocolate square with cut);
- each cut should go inside the whole chocolate bar, and all cuts must be distinct.
The picture below shows a possible way to cut a 5 × 6 chocolate for 5 times.
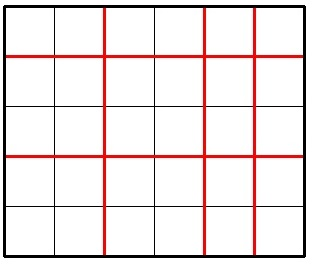
Imagine Jzzhu have made k cuts and the big chocolate is splitted into several pieces. Consider the smallest (by area) piece of the chocolate, Jzzhu wants this piece to be as large as possible. What is the maximum possible area of smallest piece he can get with exactlyk cuts? The area of a chocolate piece is the number of unit squares in it.
Input
A single line contains three integers n, m, k(1 ≤ n, m ≤ 109; 1 ≤ k ≤ 2·109).
Output
Output a single integer representing the answer. If it is impossible to cut the big chocolate k times, print -1.
Sample Input
3 4 1
6
6 4 2
8
2 3 4
-1
Hint
In the first sample, Jzzhu can cut the chocolate following the picture below:
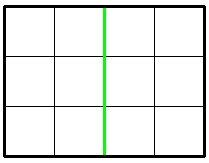
In the second sample the optimal division looks like this:
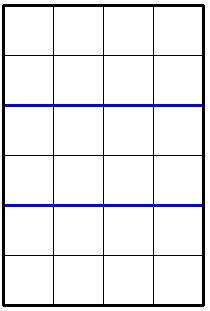
In the third sample, it's impossible to cut a 2 × 3 chocolate 4 times.
题意:一个n*m的巧克力需要切k刀,设最小面积为s,求最大s。如果不能切,输出-1
#include <iostream> #include <cstdio> #include <cstring> #include <queue> using namespace std; #define ll long long #define INF 0x3f3f3f3f #define N 110 ll ans; ll n,m,k; int main() { while(scanf("%lld%lld%lld",&n,&m,&k)!=EOF) { if(k>n+m-2) { printf("-1 "); continue; } if(k<n || k<m) { ll k1=0,k2=0; if(k<n) k1=n/(k+1)*m; if(k<m) k2=m/(k+1)*n; ans=max(k1,k2); } else { ll k1=n/(k-(m-1)+1); ll k2=m/(k-(n-1)+1); ans=max(k1,k2); } printf("%lld ",ans); } return 0; }
Description
Jzzhu is the president of country A. There are n cities numbered from 1 to n in his country. City 1 is the capital of A. Also there are mroads connecting the cities. One can go from city ui to vi (and vise versa) using the i-th road, the length of this road is xi. Finally, there are k train routes in the country. One can use the i-th train route to go from capital of the country to city si (and vise versa), the length of this route is yi.
Jzzhu doesn't want to waste the money of the country, so he is going to close some of the train routes. Please tell Jzzhu the maximum number of the train routes which can be closed under the following condition: the length of the shortest path from every city to the capital mustn't change.
Input
The first line contains three integers n, m, k(2 ≤ n ≤ 105; 1 ≤ m ≤ 3·105; 1 ≤ k ≤ 105).
Each of the next m lines contains three integers ui, vi, xi(1 ≤ ui, vi ≤ n; ui ≠ vi; 1 ≤ xi ≤ 109).
Each of the next k lines contains two integers si and yi(2 ≤ si ≤ n; 1 ≤ yi ≤ 109).
It is guaranteed that there is at least one way from every city to the capital. Note, that there can be multiple roads between two cities. Also, there can be multiple routes going to the same city from the capital.
Output
Output a single integer representing the maximum number of the train routes which can be closed.
Sample Input
5 5 3 1 2 1 2 3 2 1 3 3 3 4 4 1 5 5 3 5 4 5 5 5
2
2 2 3 1 2 2 2 1 3 2 1 2 2 2 3
2
题意:给出N个点,M条边的图,现在有L条铁路(铁路的起始点都为1)问最多可以去掉多少条铁路使得点1到其他的点的最短距离不变。
#include <iostream> #include <cstdio> #include <queue> #include <cstring> using namespace std; #define INF 0x3f3f3f3f #define N 100010 #define M 10*N struct Edge { int to,next,val; int flag; }edge[M]; int ans; int tot; int n,m,k; int dis[N]; int vis[N]; int flag[N]; int head[N]; int mp[N]; void init() { tot=0; ans=0; memset(mp,INF,sizeof(mp)); memset(flag,0,sizeof(flag)); memset(head,-1,sizeof(head)); } void add(int u,int v,int w,int flag=0) { edge[tot].to=v; edge[tot].val=w; edge[tot].flag=flag; edge[tot].next=head[u]; head[u]=tot++; } void spfa(int s) { priority_queue<int> q; //不明白为啥改成优先队列就280ms ac for(int i=1;i<=n;i++) { dis[i]=INF; vis[i]=0; } dis[s]=0; vis[s]=1; q.push(s); while(!q.empty()) { int u=q.top(); q.pop(); vis[u]=0; for(int i=head[u];i!=-1;i=edge[i].next) { int v=edge[i].to; int w=edge[i].val; if(dis[u]+w<=dis[v]) { if(!flag[v] && edge[i].flag) { ans++; flag[v]=1; } else if(flag[v] && !edge[i].flag) { ans--; flag[v]=0; } if(dis[u]+w<dis[v] && !vis[v]) { vis[v]=1; q.push(v); } dis[v]=dis[u]+w; } } } ans=k-ans; } int main() { //freopen("C:\Users\Administrator\Desktop\1.txt","r",stdin); while(scanf("%d%d%d",&n,&m,&k)!=EOF) { init(); for(int i=1;i<=m;i++) { int u,v,w; scanf("%d%d%d",&u,&v,&w); add(u,v,w); add(v,u,w); } for(int i=1;i<=k;i++) { int v,w; scanf("%d%d",&v,&w); mp[v]=min(w,mp[v]); //小优化,取最小 } for(int i=1;i<=n;i++) { if(mp[i]!=INF) { add(1,i,mp[i],1); add(i,1,mp[i],1); } } spfa(1); printf("%d ",ans); } return 0; }
Description
Jzzhu has picked n apples from his big apple tree. All the apples are numbered from 1 to n. Now he wants to sell them to an apple store.
Jzzhu will pack his apples into groups and then sell them. Each group must contain two apples, and the greatest common divisor of numbers of the apples in each group must be greater than 1. Of course, each apple can be part of at most one group.
Jzzhu wonders how to get the maximum possible number of groups. Can you help him?
Input
A single integer n(1 ≤ n ≤ 105), the number of the apples.
Output
The first line must contain a single integer m, representing the maximum number of groups he can get. Each of the next m lines must contain two integers — the numbers of apples in the current group.
If there are several optimal answers you can print any of them.
Sample Input
6
2 6 3 2 4
9
3 9 3 2 4 6 8
2
0
题意:给出n,表示数1到n,从中选出尽可能多的对数,两个数为1对,使得任意一对中两个数互质,并输出任意一种答案。
#include <iostream> #include <cstdio> #include <queue> #include <cstring> using namespace std; #define INF 0x3f3f3f3f #define N 100010 int n; int len; int vis[N]; pair<int,int> p[N]; void init() { len=0; memset(vis,0,sizeof(vis)); } void solve() { int i,j,k,t,flag; for(i=3;i<=n;i++) { if(!vis[i]) { t=0,flag=0; for(j=i;j<=n;j+=i) if(!vis[j]) t++; if((t&1) && i+i<=n && !vis[i+i]) flag=1,vis[i+i]=1; for(j=i;j<=n;j+=i) { if(!vis[j]) { for(k=j+i;k<=n;k+=i) { if(!vis[k]) { p[++len]=make_pair(j,k); vis[j]=1; vis[k]=1; j=k; break; } } } } if(flag) vis[i+i]=0; } } for(i=2;i<=n;i+=2) { if(!vis[i]) { for(j=i+2;j<=n;j+=2) { if(!vis[j]) { p[++len]=make_pair(i,j); vis[i]=1; vis[j]=1; i=j; break; } } } } } void print() { printf("%d ",len); for(int i=1;i<=len;i++) { printf("%d %d ",p[i].first,p[i].second); } } int main() { while(scanf("%d",&n)!=EOF) { init(); solve(); print(); } return 0; }