FFF at Valentine
Time Limit: 6000/3000 MS (Java/Others) Memory Limit: 65536/65536 K (Java/Others)
Total Submission(s): 730 Accepted Submission(s): 359
Problem Description
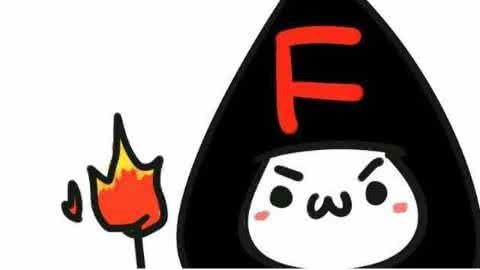
At Valentine's eve, Shylock and Lucar were enjoying their time as any other couples. Suddenly, LSH, Boss of FFF Group caught both of them, and locked them into two separate cells of the jail randomly. But as the saying goes: There is always a way out , the lovers made a bet with LSH: if either of them can reach the cell of the other one, then LSH has to let them go.
The jail is formed of several cells and each cell has some special portals connect to a specific cell. One can be transported to the connected cell by the portal, but be transported back is impossible. There will not be a portal connecting a cell and itself, and since the cost of a portal is pretty expensive, LSH would not tolerate the fact that two portals connect exactly the same two cells.
As an enthusiastic person of the FFF group, YOU are quit curious about whether the lovers can survive or not. So you get a map of the jail and decide to figure it out.
Input
∙Input starts with an integer T (T≤120), denoting the number of test cases.
∙For each case,
First line is two number n and m, the total number of cells and portals in the jail.(2≤n≤1000,m≤6000)
Then next m lines each contains two integer u and v, which indicates a portal from u to v.
Output
If the couple can survive, print “I love you my love and our love save us!”
Otherwise, print “Light my fire!”
Sample Input
Sample Output
Source
2017 Multi-University Training Contest - Team 9
//题意:给出一个有向图,问是否任意两点都可以有,至少从其中一点到另一点可行的路径
//题解:首先想到的是好像是问是否是强连通图,然后看清题后发现并不是,求出连通分量缩点后变为有向无环图后,只需要确定,有唯一的拓扑排序的结果即可

1 # include <cstring> 2 # include <cstdio> 3 # include <cstdlib> 4 # include <iostream> 5 # include <vector> 6 # include <queue> 7 # include <stack> 8 # include <map> 9 # include <bitset> 10 # include <sstream> 11 # include <set> 12 # include <cmath> 13 # include <algorithm> 14 # pragma comment(linker,"/STACK:102400000,102400000") 15 using namespace std; 16 # define LL long long 17 # define pr pair 18 # define mkp make_pair 19 # define lowbit(x) ((x)&(-x)) 20 # define PI acos(-1.0) 21 # define INF 0x3f3f3f3f3f3f3f3f 22 # define eps 1e-8 23 # define MOD 1000000007 24 25 inline int scan() { 26 int x=0,f=1; char ch=getchar(); 27 while(ch<'0'||ch>'9'){if(ch=='-') f=-1; ch=getchar();} 28 while(ch>='0'&&ch<='9'){x=x*10+ch-'0'; ch=getchar();} 29 return x*f; 30 } 31 inline void Out(int a) { 32 if(a<0) {putchar('-'); a=-a;} 33 if(a>=10) Out(a/10); 34 putchar(a%10+'0'); 35 } 36 const int N = 1005; 37 const int M = 6005; 38 /**************************/ 39 struct Edge 40 { 41 int to; 42 int nex; 43 }edge[M*2]; 44 int n,m,realm,scc,Ddex; 45 int hlist[N],hlist2[N]; 46 int dfn[N],low[N],belong[N]; 47 bool instk[N]; 48 stack<int> stk; 49 int indu[N]; 50 51 void addedge(int u,int v) 52 { 53 edge[realm] = (Edge){v,hlist[u]}; 54 hlist[u]=realm++; 55 } 56 void addedge2(int u,int v) 57 { 58 edge[realm] = (Edge){v,hlist2[u]}; 59 hlist2[u]=realm++; 60 } 61 62 void Init_tarjan() 63 { 64 Ddex=0;scc=0; 65 memset(dfn,0,sizeof(dfn)); 66 memset(instk,0,sizeof(instk)); 67 } 68 69 void tarjan(int u) 70 { 71 dfn[u]=low[u]=++Ddex; 72 stk.push(u); instk[u]=1; 73 for (int i=hlist[u];i!=-1;i=edge[i].nex) 74 { 75 int v = edge[i].to; 76 if (!dfn[v]) 77 { 78 tarjan(v); 79 low[u] = min(low[u],low[v]); 80 } 81 else if (instk[v]) 82 low[u] = min(low[u],dfn[v]); 83 } 84 if (dfn[u]==low[u]) 85 { 86 scc++; 87 while(1){ 88 int p = stk.top(); stk.pop(); 89 instk[p]=0; 90 belong[p]=scc; 91 if (u==p) break; 92 } 93 } 94 } 95 96 void build() 97 { 98 memset(hlist2,-1,sizeof(hlist2)); 99 memset(indu,0,sizeof(indu)); 100 for (int i=1;i<=n;i++) 101 { 102 for (int j=hlist[i];j!=-1;j=edge[j].nex) 103 { 104 int x = belong[i]; 105 int y = belong[edge[j].to]; 106 if (x!=y) 107 { 108 addedge2(x,y); 109 indu[y]++; 110 } 111 } 112 } 113 } 114 115 int topo() 116 { 117 queue<int> Q; 118 for (int i=1;i<=scc;i++) 119 if (indu[i]==0) Q.push(i); 120 if (Q.size()!=1) return 0; 121 while (!Q.empty()) 122 { 123 int u = Q.front(); Q.pop(); 124 for (int i=hlist2[u];i!=-1;i=edge[i].nex) 125 { 126 int v = edge[i].to; 127 indu[v]--; 128 if (indu[v]==0) 129 Q.push(v); 130 } 131 if (Q.size()>1) return 0; 132 } 133 return 1; 134 } 135 136 int main() 137 { 138 int T = scan(); 139 while (T--) 140 { 141 memset(hlist,-1,sizeof(hlist)); 142 realm=0; 143 scanf("%d%d",&n,&m); 144 for (int i=0;i<m;i++) 145 { 146 int u,v; 147 scanf("%d%d",&u,&v); 148 addedge(u,v); 149 } 150 Init_tarjan(); 151 for (int i=1;i<=n;i++) 152 if (!dfn[i]) 153 tarjan(i); 154 build();//建新图 155 if (topo())//拓扑 156 printf("I love you my love and our love save us! "); 157 else 158 printf("Light my fire! "); 159 } 160 return 0; 161 }