给自己看的代码,没写注释
20201013-汉诺塔算法:
#include<iostream>
using namespace std;
int step=1;
void move(int disk,char M,char N)
{
cout << "第"<<(step++)<<"步"<<M<<"-->"<< N<< endl;
}
int hanoi(int disk,char A,char B,char C)
{
if(disk==1)
{
move(disk,A,C);
}
else {
hanoi(disk-1,A,C,B);
move(disk,A,C);
hanoi(disk-1,B,A,C);
}
return 0;
}
int main()
{
hanoi(3,'A','B','C');
return 0;
}
20201012-快速排序算法:
#include<iostream>
using namespace std;
int quicksort(int arr[],int i,int j) {
if(i<j)
{
int temp=arr[i];
int start=i;
int end=j;
while(start<end) {
while(start<end&&arr[end]>temp)
end--;
if(start<end)
{
arr[start]=arr[end];
start++;
}
while( start<end&&arr[start]<temp)
start++;
if(start<end)
{
arr[end]=arr[start];
end--;
}
}
arr[ start]=temp;
quicksort(arr,i,start-1);
quicksort(arr,start+1,j);
}
return 0;
}
int main()
{
int arr[]= {5,2,9,1,4,3,6,0};
quicksort(arr,0,7);
for( int i=0; i<8; i++)
cout << arr[i];
return 0;
}
二叉树实现:
#include<iostream>
using namespace std;
typedef struct BiTNode {
char data;
struct BiTNode *lchild,*rchild;
} BiTNode,*BiTree;
void Create(BiTree &T)
{
char ch;
cin >> ch;
if(ch!='#')
{
T=new BiTNode;
T->data=ch;
Create(T->lchild);
Create(T->rchild);
}
else {
T=NULL;
}
}
void midprint(BiTree T)
{
if(T==NULL)
return;
midprint(T->lchild);
cout << T->data;
midprint(T->rchild);
}
void preprint(BiTree T)
{
if(T==NULL)
return;
cout << T->data;
preprint(T->lchild);
preprint(T->rchild);
}
void postprint(BiTree T)
{
if(T==NULL)
return;
postprint(T->lchild);
postprint(T->rchild);
cout << T-> data;
}
int main()
{
BiTNode* head;
Create(head);
cout << "先序输出:" << endl;
preprint(head);
cout << "
中序输出:" << endl;
midprint(head);
cout << "
后序输出:" << endl;
postprint(head);
return 0;
}
20201014-动态规划最长子序列:
package test1;
public class test {
static int LCS(String s1,String s2){
int L1=s1.length()+1;
int L2=s2.length()+1;
int[][] map=new int[L1][L2]; //表示方向
int[][] steps=new int[L1][L2]; //表示相同序列长度
for(int i=1;i<L1;i++){
for(int j=1;j<L2;j++)
{
if(s1.charAt(i-1)==s2.charAt(j-1)){
steps[i][j]=steps[i-1][j-1]+1;
map[i][j]=1;
}
else if(s1.charAt(i-1)!=s2.charAt(j-1))
{
if(steps[i-1][j]>=steps[i][j-1])
{
steps[i][j]=steps[i-1][j];
map[i][j]=3;
}
else
{
steps[i][j]=steps[i][j-1];
map[i][j]=2;
}
}
}
}
int m=L1-1;
int n=L2-1;
int sign=steps[L1-1][L2-1];
while(sign>0)
{
//System.out.println(m+" "+n+" "+map[m][n]);
if(map[m][n]==1)
{
System.out.print(m+" "+n+" ");
System.out.println(s1.charAt(m-1));
n--;
m--;
sign--;
}
else if(map[m][n]==2)
n--;
else if(map[m][n]==3)
m--;
}
for(int i=1;i<L1;i++){
for(int j=1;j<L2;j++)
{
System.out.print(map[i][j]);
}
System.out.println();
}
return steps[L1-1][L2-1];
}
public static void main(String[] args) {
// TODO Auto-generated method stub
System.out.print("最长子序列长度为"+LCS("ABCPDSFJGODIHJOFDIUSHGD","OSDIHGKODGHBLKSJBHKAGHI"));
}
}
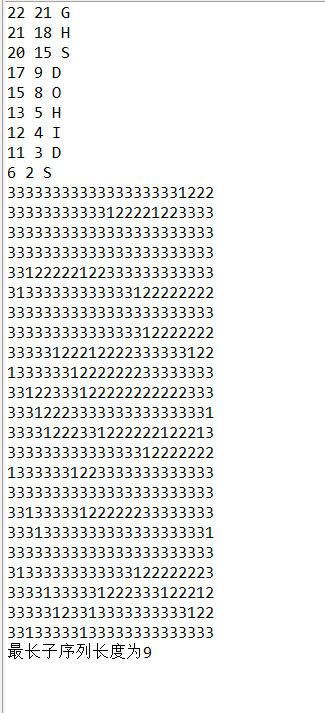
2020101x-递归反转栈: