一、Spring 框架
什么是框架[framework]?
在某个问题域中的一整套解决方案,在javaee体系下,不同的问题域[场景]下面有不同的框架,比如:在持久层中有 mybatis, Hibernate, Jdo等框架,还有MVC的框架,如:struts2, spring mvc、JSF、等。
Spring 是什么?
直译过来就是“春天”,它是由作者Rod Johnson 为改变早期企业级项目开发过程中使用EJB所遇到的问题而开发的一套轻量级的企业应用开发解决方案,取名 Spring, 【意味着程序员的“春天”】
现在, Spring Framework 是整个JAVAEE开发生态中必不可少的一个环节,大量的JAVAEE项目都是基于Spring框架来展开的,Spring Framework也构建了一个很好的技术生态,基本上可以提供给程序员一整套的技术链来完成企业级项目的开发。
Spring Framework 目前最新版本是 5.x, 它的技术生态所含盖的技术和框架也很多,主要有:
- Spring MVC 框架
- Spring Boot
- Spring Cloud
- Spring Security
- Spring cache
- …
Spring Framework 核心概念
- IOC 容器, 也叫 Inverse of Control, 控制反转, 换成另外一个词更好理解,叫 依赖注入 Dependency Injection, 简称 DI。
- AOP, 面向切面编程
- 数据访问支持层,包含 JDBC的技术, mybatis的支持,hibernate的支持, JPA 的支持.
- 事务的支持
- 测试的支持
- …
了解Spring 框架的架构图
从上图可以看出,Spring core Container 是支撑所有上面技术组件的基础,其中beans是负责创建由spring管理的各种对象,context是容器应用上下文, SPEL是表达式语言,core 就是框架的核心代码码,一般无需程序员参与。
test 是测试组件,也是所有上面技术的组件所共用的。
左上角是数据持久层的支持,包含 JDBC、ORM[hibernate框架和mybatis框架]、OXM[java object和xml之间的转换]、以及JMS的支持、当然还有事务的支持。
右上角是 针对MVC的实现,由 spring mvc负责。
二、Spring Framework 之 IOC
开发的步骤流程
- 准备一个maven项目
- 导入相关的依赖 [spring-context]和[spring-test]
- 编写相关的代码
- 测试
Spring IOC的特性
- 采用单例模型,也就是说在IOC容器中,默认只创建配置Bean的唯一实例
- 通过 scope 属性来控制容器是否采用单例,默认是 单例 [singleton]
- 设置 scope=“prototype” ,表示IOC容器采用 原型模式,也就是不是单例。
- 采用“懒加载“方式来创建目标Bean对象
- 同一个接口类型,有多个实现的情况下,就会产生“二义性”。怎么解决?
- 再加一个 名字 进行限定。
所以,默认情况下, Spring IOC中,Bean的搜索是根据 类型 来搜索的[by Type], 如果类型存在“二义性”时,则需要进一步通过bean的名称[id] 来搜索,这个叫 by Name。
IOC容器核心API
- ApplicationContext 接口,这就是IOC的核心容器接口,通过它可以获取被IOC容器管理的Bean
- 支持两种不同的配置:
-
XML 配置,传统的配置,一直很强大,很好用,如下: [ applicationContext.xml ]
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd"> <!-- 定义我们的Bean, 在此定义的Bean,就会由spring容器负责创建和管理 --> <bean id="studentService" scope="singleton" init-method="xxx" class="com.hc.service.impl.StudentServiceImpl"/> </beans>
-
注解的配置,JDK5.0之后才支持的,现在很流行。 如下: [ AppConfig.java ]
@Configuration //表示这是一个spring 注解配置类 @ComponentScan(value = {"com.hc.service.impl"}) public class AppConfig { //nothing! }
-
ApplicationContext 的实现类
\- ClassPathXmlApplicationContext 如果采用xml配置,使用这个类
\- AnnotationConfigApplicationContext [简称 ACAC], 如果使用注解配置,使用这个类
三、Spring-test 环境
-
如果你想手动编写代码来获取Spring IOC 的上下文,这种情况下,是无需Spring-test 组件的,如下:
/****** * 测试[非 Spring-test 环境] */ public class StudentServiceTest { @Test public void testAdd_use_spring_annotation() { //手工写代码得到 ac [也就是 spring ioc 上下文] ApplicationContext ac = new AnnotationConfigApplicationContext(AppConfig.class); //通过 ac 来获取被IOC容器管理的bean IStudentService iss = ac.getBean(IStudentService.class); // iss.add(null); } }
- 当然,建议是使用 spring-test 组件来做单元测试,它需要使用如下注解
- @ContextConfiguration(classes=xxxx.class)
- @RunWith(SpringJUnit4ClassRunner.class)
```java
@ContextConfiguration(classes = AppConfig.class) //读取spring的注解配置类
@RunWith(SpringJUnit4ClassRunner.class) //把上面读取到的IOC上下文由测试环境来使用
public class StudentServiceTestWithSpring {
@Autowired
private IStudentService studentService;
@Test
public void testAdd_use_spring_annotation_test() {
System.out.println(studentService);
studentService.add(null);
}
}
```
-
这种使用 spring-test 组件进行测试的方式,是官方推荐的。它还可以这么写:
@ContextConfiguration(classes = AppConfig.class) //读取spring的注解配置类 public class StudentServiceTestWithSpring extends AbstractJUnit4SpringContextTests { @Autowired private IStudentService studentService; @Test public void testAdd_use_spring_annotation_test() { System.out.println(studentService); studentService.add(null); } }
IOC相关的注解
- @Component 组件,修饰类的,需要被容器管理的bean类型,现在使用很少,因为有下面三个来代替它:
* @Service 从语义上扩展了@Component, 代表 服务层的bean
* @Repository 从语义上扩展了@Component, 代表 DAO层的bean
* @Controller 从语义上扩展了@Component, 代表 控制层的bean
- @Autowired 自动注入,它是 byType 进行搜索的。
- @Qualifier 进一步限定搜索的Bean,按姓名搜索,也就是 byName
- @Resource 它是JAVAEE规范中的注解,spring IOC 实现了这个注解,它即支持byType,也支持byName
- @Configuration 表示此类是一个Spring的注解配置类
- @ComponentScan 用来指定要扫描的包,可以指定多个
- @ContextConfiguration 它是spring-test的注解,用在测试类上面,来读取目标配置类
- @RunWith 也是spring-test的注解,用在测试类上面,让测试类拥有spring 框架的上下文环境。
有关数据源配置
数据源是用来配置数据库的连接的,它是自动参与到连接池管理之中,一般都无需我们去实现数据源,都是由第三方的组件提供的,目前最主流的数据源技术组件【连接池实现】有:
- commons-dbcp 组件, 由 apache 提供的
- druid 组件,由 alibaba 提供
- hikari 组件,一家日本公司
- …
我们选用 druid 数据源,所以,在pom.xml中要加入 druid的依赖.
配置数据源相关的属性
- url
- driver
- username
- password
- …
Note:
欢迎点赞,留言,转载请在文章页面明显位置给出原文链接
知者,感谢您在茫茫人海中阅读了我的文章
没有个性 哪来的签名!
详情请关注点我
持续更新中
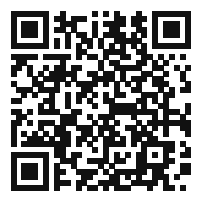
© 2020 09 - Guyu.com | 【版权所有 侵权必究】