分页功能 封装代码
分页代码 逻辑和样式
from django.utils.safestring import mark_safe
from django.http.request import QueryDict
class Pagination:
"""
需要参数
page: 当前的页码数(必填)
all_count: 总的数据量(可填)
per_num : 每页显示的数据量(选填)
max_show: 最多显示的页码数(选填)
"""
def __init__(self, page, all_count, per_num=10, max_show=11):
try:
self.page = int(page)
if self.page <= 0:
self.page = 1
except Exception:
self.page = 1
# 总的数据量
all_count = all_count
# 每页显示的数据量 10
# 总的页码数
total_num, more = divmod(all_count, per_num)
if more:
total_num += 1
# 最大显示的页码数
half_show = max_show // 2
if total_num <= max_show:
page_start = 1
page_end = total_num
else:
if self.page - half_show <= 0:
# 页码的起始值
page_start = 1
# 页码的终止值
page_end = max_show
elif self.page + half_show > total_num:
page_end = total_num
page_start = total_num - max_show + 1
else:
# 页码的起始值
page_start = self.page - half_show
# 页码的终止值
page_end = self.page + half_show
self.page_start = page_start
self.page_end = page_end
self.total_num = total_num
self.start = (self.page - 1) * per_num
self.end = self.page * per_num
@property
def page_html(self):
li_list = []
if self.page == 1:
li_list.append(
'<li class="disabled"><a aria-label="Previous"> <span aria-hidden="true">«</span></a></li>')
else:
li_list.append(
'<li><a href="?page={}" aria-label="Previous"> <span aria-hidden="true">«</span></a></li>'.format(
self.page - 1))
for i in range(self.page_start, self.page_end + 1):
if i == self.page:
li_list.append('<li class="active"><a href="?page={}">{}</a></li>'.format(i, i))
else:
li_list.append('<li><a href="?page={}">{}</a></li>'.format(i, i))
if self.page == self.total_num:
li_list.append(
'<li class="disabled"><a aria-label="Next"> <span aria-hidden="true">»</span></a></li>')
else:
li_list.append(
'<li><a href="?page={}" aria-label="Next"> <span aria-hidden="true">»</span></a></li>'.format(
self.page + 1))
return ''.join(li_list)
View 使用示列
# 公私户,批量操作
class CustomerList(View):
def get(self, request, *args, **kwargs):
q = self.search(["qq", "name", "phone"])
if request.path_info == reverse('customer_list'):
title = '公共客户'
all_customer = models.Customer.objects.filter(q, consultant__isnull=True)
else:
title = '我的客户'
all_customer = models.Customer.objects.filter(q, consultant=request.user_obj)
page = Pagination(request.GET.get('page', 1), all_customer.count(), request.GET.copy(), 3)
return render(request, 'consultant/customer_list.html',
{"all_customer": all_customer[page.start:page.end], 'page_html': page.page_html, "title": title}) #传入参数
html 页面引用 bootstrap样式
<script src="bootstrap-3.3.7/css/bootstrap.css"></script>
<nav aria-label="Page navigation">
<ul class="pagination">
{{ page_html }}
</ul>
</nav>
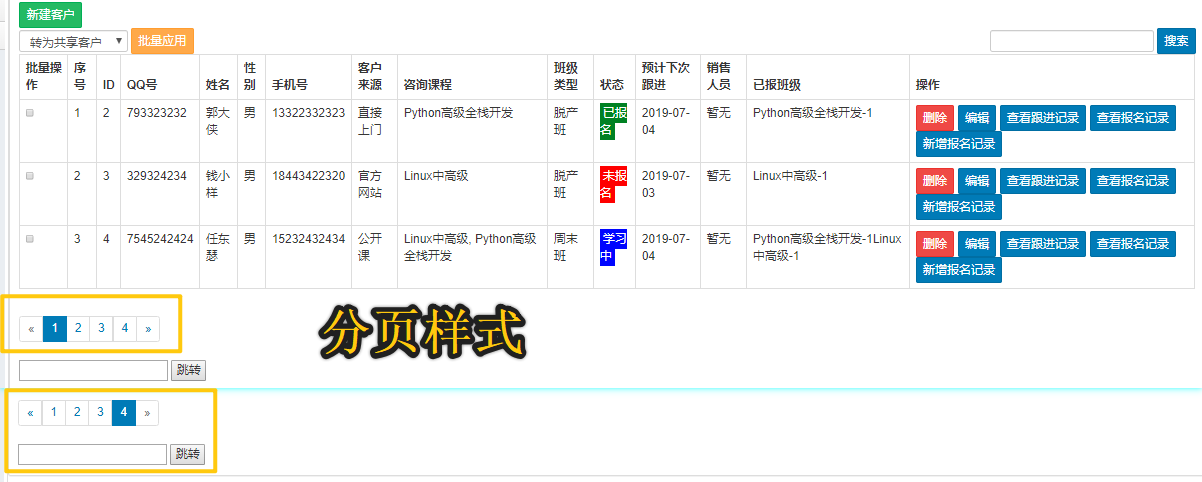