条件查询
Model.find(conditions, [fields], [options], [callback])
demo1
try.js
var User = require("./user.js"); function getByConditions(){ var wherestr = {'username' : 'xiaoming'}; User.find(wherestr, function(err, res){ if (err) { console.log("Error:" + err); } else { console.log("Res:" + res); } }) } getByConditions();
在robo查看数据库:
在webstorm 中查看输出结果:
demo2
try.js
var User = require("./user.js"); function getByConditions(){ var wherestr = {'username' : 'xiaoming'}; var opt = {"username": 1 ,"_id": 0}; User.find(wherestr,opt, function(err, res){ if (err) { console.log("Error:" + err); } else { console.log("Res:" + res); } }) } getByConditions();
输出结果:
年龄查询
在robo查看数据库:
try.js
var User = require("./user.js"); function getByConditions(){ User.find({userage: {$gte: 13, $lte: 30}}, function(err, res){ if (err) { console.log("Error:" + err); } else { console.log("Res:" + res); } }) } getByConditions();
$gte: 13, $lte: 30:表示大于等于13而且小于等于30岁
在webstorm中输出结果:
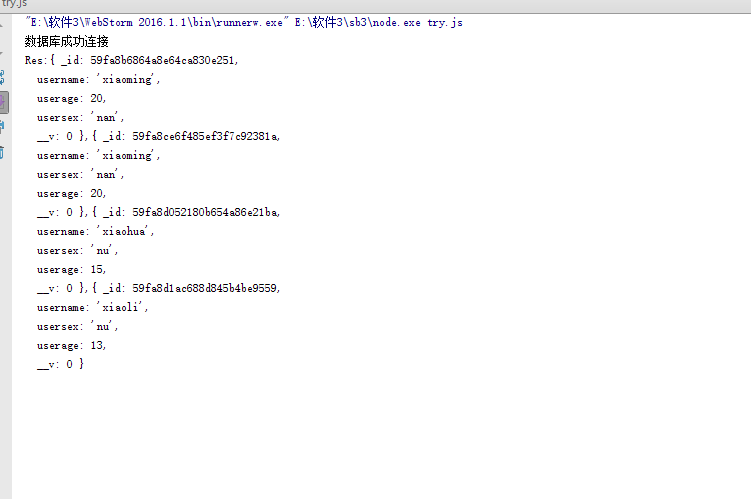
数量查询
Model.count(conditions, [callback])
try.js
var User = require("./user.js"); function getCountByConditions(){ var wherestr={}; User.count(wherestr, function(err, res){ if (err) { console.log("Error:" + err); } else { console.log("Res:" + res); } }) } getCountByConditions();
在webstorm中输出结果:
Model.findById(id, [fields], [options], [callback])
在robo中查看 id=59fa8b401061f8333095975a 的语句
try.js
var User = require("./user.js"); function getById(){ var id='59fa8b401061f8333095975a'; User.findById(id, function(err, res){ if (err) { console.log("Error:" + err); } else { console.log("Res:" + res); } }) } getById();
输出结果:
模糊查询
try.js
var User = require("./user.js"); function getByRegex(){ var whereStr={'username':{$regex:/z/i}}; User.find(whereStr, function(err, res){ if (err) { console.log("Error:" + err); } else { console.log("Res:" + res); } }) } getByRegex();
上面示例中查询出所有用户名中有'z'的名字,不区分大小写
输出结果:
2017-11-02 11:41:51