array是固定大小的数组,和我们用的 [ ] 形式数组差不多。
特点:
1. 只保存里面元素,连元素大小都不保存 Internally, an array does not keep any data other than the elements it contains (not even its size, which is a template parameter, fixed on compile time)
2. 大小为0是合法的,但是不要解引用。Zero-sized arrays are valid, but they should not be dereferenced (members front, back, and data).
常见操作
array<int, 6> arr {1,2,3,4,5,6};
arr.begin() // 第一个元素地址
Return iterator to beginning (public member function )
arr.end() // 最后一个元素的下一个元素地址 ,和begin组合起来是数学中的[a,b) ,左闭右开区间
Return iterator to end (public member function )
arr.rbegin() // 翻转begin
Return reverse iterator to reverse beginning (public member function )
arr.rend() // 翻转end
Return reverse iterator to reverse end (public member function )
arr.cbegin() // const begin的意思
Return const_iterator to beginning (public member function )
arr.cend() // const end的意思
Return const_iterator to end (public member function )
arr.crbegin() // const rbegin 的意思
Return const_reverse_iterator to reverse beginning (public member function )
arr.crend() // const rbegin 的意思
Return const_reverse_iterator to reverse end (public member function )
arr.size()
Return size (public member function )
arr.max_size() // 永远和arr.size()一样是初始化的那个数字即 array <int ,6> 中的6
Return maximum size (public member function )
arr.empty()
Test whether array is empty (public member function )
arr[i] //不检查下标是否越界
Access element (public member function )
arr.at(i) //会检查下标是否越界
Access element (public member function )
arr.front() // 返回引用,可以当左值,第一个元素
Access first element (public member function )
arr.back() // 返回引用,可以当左值,最后一个元素
Access last element (public member function )
arr.data() //返回指向数组首元素的指针
Get pointer to data (public member function )
Modifiers
arr.fill(num) // 把数组中所有元素都填为num
Fill array with value (public member function )
arr.swap(arr2) // 同另外一个数组交换元素,同类型,同大小
Swap content (public member function )
当反向遍历时,迭代器++ 其实是迭代器 --
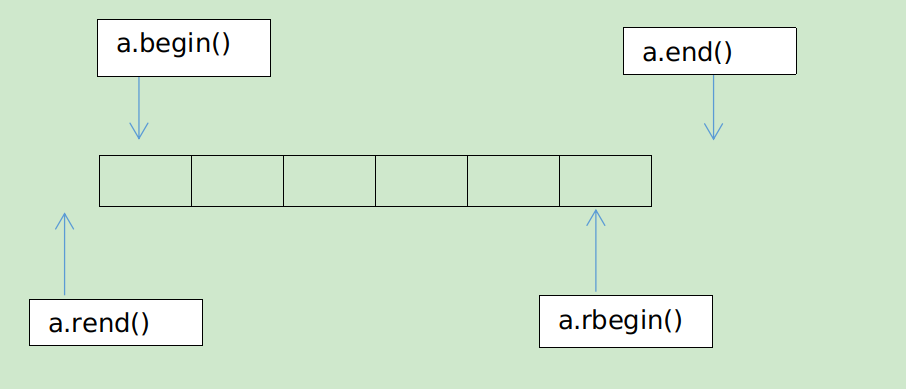
#include <iostream> #include <array> using namespace std; int main(void) { array<int,0> test; cout << test.size() << endl; // 0 cout << test.empty() << endl; //是空的,返回真,1 array<int, 6> a = {0, 1, 2, 3, 4, 5}; for (auto i : a) { cout << a[i] << endl; } cout << a.size() << endl; // 等于6 for (auto it = a.data(); it != a.end(); it++) { cout << *it << endl; } cout << a[7] << endl; // 不会检查是否越界,返回一个随机值 //cout << a.at(7); // 会检查越界,编译不过 for (auto it = a.rbegin(); it != a.rend(); it++) { cout << *it << endl; // 5 4 3 2 1 0 } }