You are given a map in form of a two-dimensional integer grid where 1 represents land and 0 represents water. Grid cells are connected horizontally/vertically (not diagonally). The grid is completely surrounded by water, and there is exactly one island (i.e., one or more connected land cells). The island doesn't have "lakes" (water inside that isn't connected to the water around the island). One cell is a square with side length 1. The grid is rectangular, width and height don't exceed 100. Determine the perimeter of the island.
Example:
[[0,1,0,0], [1,1,1,0], [0,1,0,0], [1,1,0,0]] Answer: 16
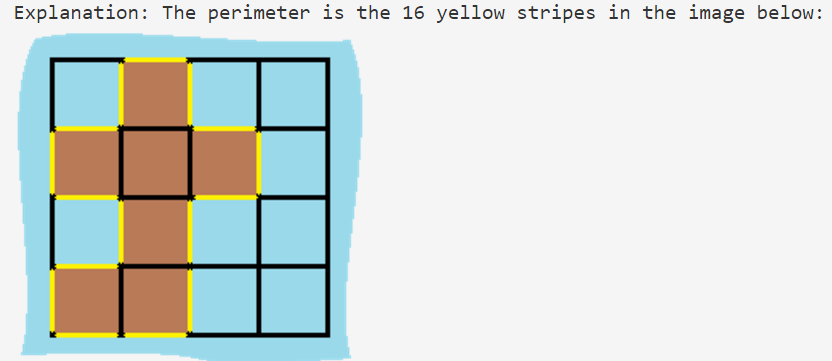
分析:
既然要求所有island的周长,那么一个island的周长是4,但是因为重叠部分不计算长度,所以我们要减去上下左右重叠部分的长度。代码如下:
function caculate(grids) { let total = 0; for(let outer = 0; outer < grids.length; outer++) { for(let inner = 0; inner < grids[outer].length; inner++) { if(grids[outer][inner] == 1) { let sum = 4; //left if((inner -1) >= 0 && grids[outer][inner-1] == 1) { sum--; } //right if((inner + 1) < grids[outer].length && grids[outer][inner+1] == 1 ){ sum--; } //top if(outer-1 >= 0 && grids[outer-1][inner] == 1){ sum--; } //bottom if(outer +1 <= grids.length-1 && grids[outer +1][inner] == 1){ sum--; } total+= sum; } } } return total; } console.log(caculate([[0,1,0,0],[1,1,1,0],[0,1,0,0],[1,1,0,0]]));