文件上传(Servlet/Struts2/SpringMVC)的链接:http://www.cnblogs.com/ghq120/p/8312944.html
文件下载
Servlet实现
目录结构
DownLoadServlet.java
package com.ghq.controller;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.OutputStream;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class DownLoadServlet extends HttpServlet {
public void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
this.doPost(request, response);
}
public void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
String imageRP = this.getServletContext().getRealPath("/images");
//获取被下载的文件的名字
String oldfileName = request.getParameter("fn");
//代表要下载的文件
File sourceFile = new File(imageRP, oldfileName);
//被下载文件对应的输入流
FileInputStream fin = new FileInputStream(sourceFile);
//将文件名进行重新编码,解决下载中文文件名时,出现乱码的情况
oldfileName = new String(oldfileName.getBytes(),"ISO8859-1");
//设置响应头,表示是下载文件,不是给浏览器输出
response.addHeader("Content-Disposition", "attachment;filename="+oldfileName);
OutputStream out = response.getOutputStream();
byte[] bs = new byte[1024];
int count = 0;
while((count = fin.read(bs))!= -1){
out.write(bs, 0, count);
}
if (out != null) {
out.close();
}
if (fin != null) {
fin.close();
}
}
}
web.xml配置
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0">
<display-name>DownLoadFile</display-name>
<servlet>
<servlet-name>DownLoadServlet</servlet-name>
<servlet-class>com.ghq.controller.DownLoadServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>DownLoadServlet</servlet-name>
<url-pattern>/DownLoadServlet</url-pattern>
</servlet-mapping>
</web-app>
jsp页面
<body>
<a href="<%=path%>/DownLoadServlet?fn=测试.png">测试.png </a>
</body>
Struts2的文件下载
项目的目录结构
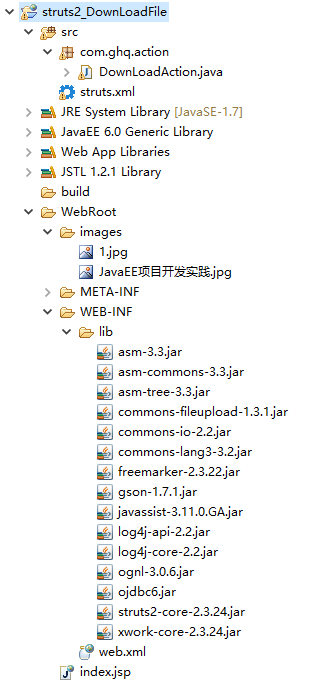
DownLoadAction.java
package com.ghq.action;
import java.io.InputStream;
import java.io.UnsupportedEncodingException;
import javax.servlet.ServletContext;
import org.apache.struts2.ServletActionContext;
import com.opensymphony.xwork2.ActionSupport;
public class DownLoadAction extends ActionSupport {
private String filename;
public String getFilename() {
//解决被下载文件的名字是中文时乱码的情况
try {
filename=new String(filename.getBytes(),"ISO8859-1");
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
}
return filename;
}
public void setFilename(String filename) {
this.filename = filename;
}
public InputStream getTargetFile() throws Exception{
//获取ServletContext的对象
ServletContext application = ServletActionContext.getServletContext();
InputStream fin = application.getResourceAsStream("/images/"+filename);
return fin;
}
}
struts.xml
tatgetFile和DownLoadAction.java中的下载文件的方法名getTargetFile()对应
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.3//EN"
"http://struts.apache.org/dtds/struts-2.3.dtd">
<struts>
<constant name="struts.enable.DynamicMethodInvocation" value="false" />
<constant name="struts.devMode" value="true" />
<constant name="struts.action.extension" value="action"/>
<package name="default" namespace="/" extends="struts-default">
<action name="DownLoadAction_*" class="com.ghq.action.DownLoadAction" method="{1}">
<result type="stream">
<param name="inputName">targetFile</param>
<!-- attachment用于指定是下载文件,而不是解析文件 -->
<param name="contentDisposition">attachment;filename=${filename}</param>
</result>
</action>
</package>
</struts>
web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0">
<display-name>struts2_DownLoadFile</display-name>
<welcome-file-list>
<welcome-file>index.jsp</welcome-file>
</welcome-file-list>
<filter>
<filter-name>struts2</filter-name>
<filter-class>org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>struts2</filter-name>
<url-pattern>*.action</url-pattern>
</filter-mapping>
</web-app>
jsp页面
<body>
JavaEE项目开发实践.jpg <a href="<%=path %>/DownLoadAction.action?filename=JavaEE项目开发实践.jpg">下载</a><br>
1.jpg <a href="<%=path %>/DownLoadAction.action?filename=1.jpg">下载</a>
</body>