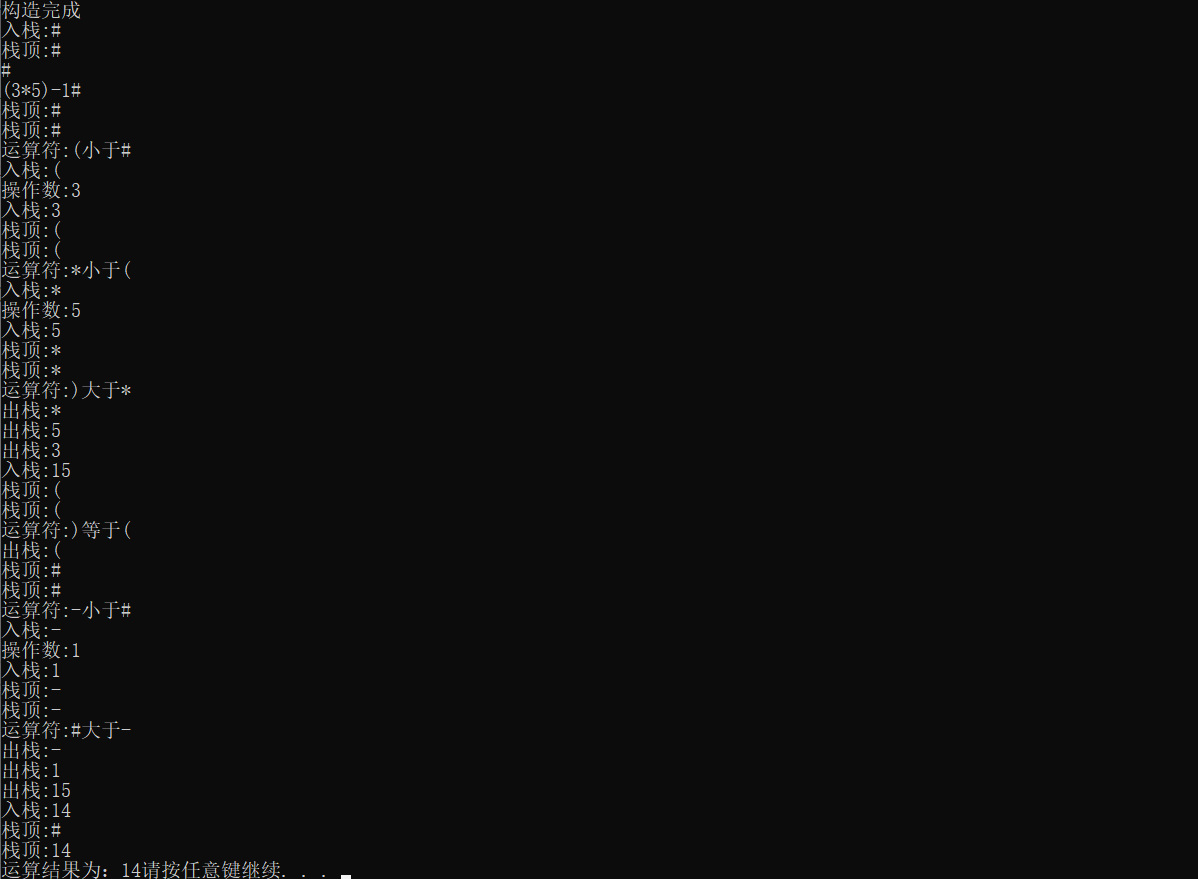
#include<stdio.h> #include<stdlib.h> #include<iostream> using namespace std; #define STACK_SIZE 100 #define STACKINCREMENT 10 template<class T> class MyStack { private: T* top; T* bottom; int stacksize; public: MyStack Init_Stack() { this->bottom = (T*)malloc(STACK_SIZE * sizeof(T)); //this->bottom = new T[stacksize * sizeof(T)]; if (!this->bottom) return *this; this->top = this->bottom; this->stacksize = STACK_SIZE; cout << "构造完成" << endl; } MyStack push(T e) { if (this->top - this->bottom >= this->stacksize) { this->bottom = (T*)realloc(this->bottom,(this->stacksize + STACKINCREMENT) * sizeof(T)); if (!this->bottom) return *this; this->top = this->bottom + this->stacksize; this->stacksize += STACKINCREMENT; } *this->top = e; this->top++; cout << "入栈:" << e << endl; } T pop() { if (this->top == this->bottom) return 0; this->top--; T e; e = *this->top; cout << "出栈:"<< e << endl; return e; } T GetTop() { if (this->top == this->bottom) return 0; T e; e = *(this->top - 1); cout << "栈顶:"<< e << endl; return e; } }; //bool isNumber(char x) { // char a[10] = { 1,2,3,4,5,6,7,8,9,0 }; // for (int i = 0; i <= 9; i++) { // if (x == 'a[i]') { // cout << a[i]; // return true; // } // } // return false; //} bool isNumber(char x) { if (x <= '9' && x >= '0') { return true; } else return false; } char Precede(char a,char b) { if (a == '+' || a == '-') { if (b == '+' || b == '-' || b == ')' || b == '#') return '>'; else return '<'; } else if (a == '*' || a == '/') { if (b == '+' || b == '-' || b == ')' || b == '#' || b == '*' || b == '/') return '>'; else return '<'; } else if (a == '(') { if (b == ')') return '='; else return '<'; } else if (a == ')') { return '>'; } else if (a == '.') { return '>'; } else { if (b == '#') return '='; else return '<'; } } double Operate(double a,char s,double b) { if (s == '+') { return a + b; } else if (s == '-') { return a - b; } else if (s == '*') { return a * b; } else if (s == '/') { if (b == 0) { cout << "除数不能为0" << endl; exit(3); system("pause"); } else return a / b*1.0; } else if (s == '.') { double x = b; if (b == 0) { return 0.0 + a; } else while(x>=1) { x = (x / 10)*1.0; cout << x << endl; } return a + x; } } double run() { int a = 0, b = 0; bool isNumber(char x); double Operate(double a, char s, double b); char Precede(char a, char b); MyStack<double> opnd; opnd.Init_Stack(); MyStack<char> opter; opter.Init_Stack(); opter.push('#'); cout << opter.GetTop() << endl; char c; c = getchar(); //cout << "是否数字" << isNumber(c) << endl; while (c != '#' || opter.GetTop() != '#') { double y = 0; int t = 0; while (isNumber(c)) { { //opnd.pop(); y = y * 10 + double (c - '0'); cout << "操作数:" << y << endl; t++; c = getchar(); } //cout << "是否数字" << isNumber(c) << endl; //cout << c<<endl; //double x; //x=c-'0'; //cout << x << endl; //opnd.push(x); //c = getchar(); //cout << "Number:" << opnd.GetTop() << endl; //cout << "Number:" << opter.GetTop() << endl; } if (t) { opnd.push(y); } switch (Precede(opter.GetTop(), c)) { case '<': cout << "运算符:" << c << "小于" << opter.GetTop() << endl; opter.push(c); if (c == ')') { a = 1; } c = getchar(); if (a == 0 && !isNumber(c) && c != '(' && c!= '#') { cout << "算式格式不正确" << endl; exit(3); system("pause"); } //cout << "小于:" << opnd.GetTop() << endl; break; case '=': cout << "运算符:" << c << "等于" << opter.GetTop() << endl; opter.pop(); if (c == ')') { b = 1; } c = getchar(); if (b == 0 && !isNumber(c) && c != '(' && c != '#') { cout << "算式格式不正确" << endl; exit(3); system("pause"); } //cout << "等于:" << opnd.GetTop(); break; case '>': cout << "运算符:" << c << "大于" << opter.GetTop() << endl; double a, b; char theta; theta = opter.pop(); a = opnd.pop(); b = opnd.pop(); opnd.push(Operate(b, theta, a)); break; } // . 应该小于# } return opnd.GetTop(); } int main() { cout << "运算结果为:" << run(); system("pause"); return 0; }
此程序包含小数运算(.运算的实现) 以及格式是否正确 比较全面