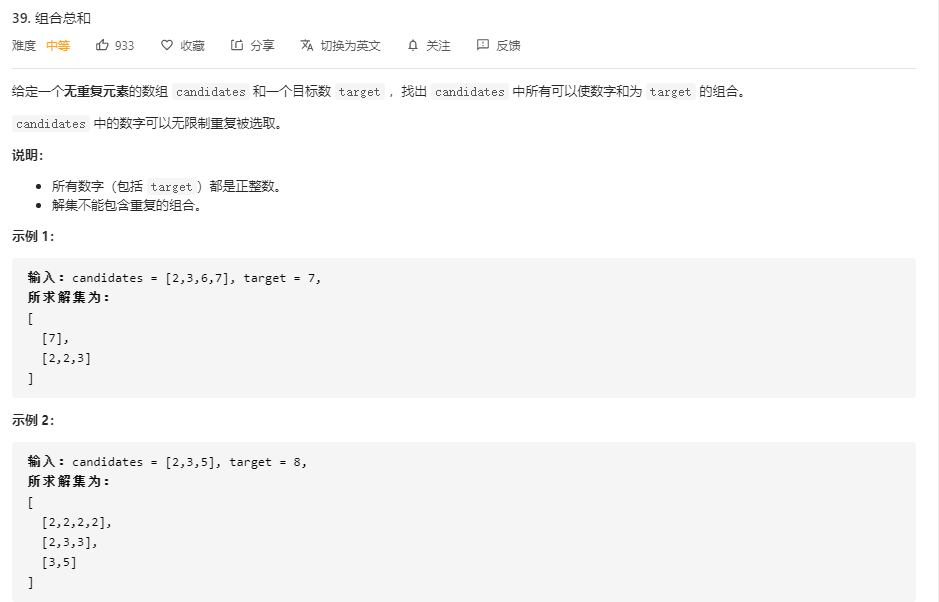
package com.example.lettcode.dailyexercises;
import java.util.ArrayList;
import java.util.List;
/**
* @Class CombinationSum
* @Description 39 组合总和
* 给定一个无重复元素的数组 candidates 和一个目标数 target ,找出 candidates 中所有可以使数字和为 target 的组合。
* candidates 中的数字可以无限制重复被选取。
* 说明:
* 所有数字(包括 target)都是正整数。
* 解集不能包含重复的组合。
* <p>
* 示例 1:
* 输入:candidates = [2,3,6,7], target = 7,
* 所求解集为:
* [
* [7],
* [2,2,3]
* ]
* <p>
* 示例 2:
* 输入:candidates = [2,3,5], target = 8,
* 所求解集为:
* [
* [2,2,2,2],
* [2,3,3],
* [3,5]
* ]
* 提示:
* 1 <= candidates.length <= 30
* 1 <= candidates[i] <= 200
* candidate 中的每个元素都是独一无二的。
* 1 <= target <= 500
* @Author
* @Date 2020/9/9
**/
public class CombinationSum {
public static List<List<Integer>> combinationSum(int[] candidates, int target) {
List<List<Integer>> ans = new ArrayList<List<Integer>>();
List<Integer> combine = new ArrayList<Integer>();
dfs(candidates, target, ans, combine, 0);
return ans;
}
/**
* 回溯算法
* @param candidates
* @param target 目标数
* @param ans 最终结果
* @param combine 用于保存每一组的数据
* @param idx 遍历到的位置
*/
public static void dfs(int[] candidates, int target, List<List<Integer>> ans, List<Integer> combine, int idx) {
if (idx == candidates.length) {
return;
}
if (target == 0) {
ans.add(new ArrayList<Integer>(combine));
return;
}
// 选择当前数
if (target - candidates[idx] >= 0) {
combine.add(candidates[idx]);
dfs(candidates, target - candidates[idx], ans, combine, idx);
combine.remove(combine.size() - 1);
}
// 直接跳过
dfs(candidates, target, ans, combine, idx + 1);
}
public static void main(String[] args) {
int[] candidates = new int[]{2, 3, 6, 7};
int target = 7;
List<List<Integer>> res = combinationSum(candidates, target);
System.out.println("CombinationSum demo01 result:");
for (List<Integer> integerList : res) {
for (Integer integer : integerList) {
System.out.print("," + integer);
}
System.out.println();
}
candidates = new int[]{2,3,5};
target = 8;
res = combinationSum(candidates, target);
System.out.println("CombinationSum demo02 result:");
for (List<Integer> integerList : res) {
for (Integer integer : integerList) {
System.out.print("," + integer);
}
System.out.println();
}
}
}