原题链接:http://acm.hdu.edu.cn/showproblem.php?pid=1022
Train Problem I
Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others)Total Submission(s): 13410 Accepted Submission(s): 4935
Problem Description
As the new term comes, the Ignatius Train Station is very busy nowadays. A lot of student want to get back to school by train(because the trains in the Ignatius Train Station is the fastest all over the world ^v^). But here comes a problem, there is only one
railway where all the trains stop. So all the trains come in from one side and get out from the other side. For this problem, if train A gets into the railway first, and then train B gets into the railway before train A leaves, train A can't leave until train
B leaves. The pictures below figure out the problem. Now the problem for you is, there are at most 9 trains in the station, all the trains has an ID(numbered from 1 to n), the trains get into the railway in an order O1, your task is to determine whether the
trains can get out in an order O2.
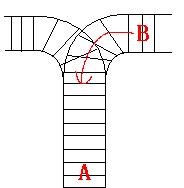

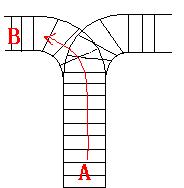
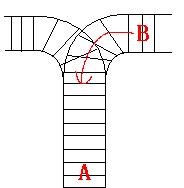

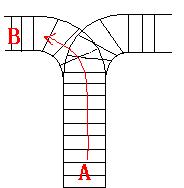
Input
The input contains several test cases. Each test case consists of an integer, the number of trains, and two strings, the order of the trains come in:O1, and the order of the trains leave:O2. The input is terminated by the end of file. More details in the Sample
Input.
Output
The output contains a string "No." if you can't exchange O2 to O1, or you should output a line contains "Yes.", and then output your way in exchanging the order(you should output "in" for a train getting into the railway, and "out" for a train getting out of
the railway). Print a line contains "FINISH" after each test case. More details in the Sample Output.
Sample Input
3 123 321 3 123 312
Sample Output
Yes. in in in out out out FINISH No. FINISHFor the first Sample Input, we let train 1 get in, then train 2 and train 3. So now train 3 is at the top of the railway, so train 3 can leave first, then train 2 and train 1. In the second Sample input, we should let train 3 leave first, so we have to let train 1 get in, then train 2 and train 3. Now we can let train 3 leave. But after that we can't let train 1 leave before train 2, because train 2 is at the top of the railway at the moment. So we output "No.".HintHint
Author
Ignatius.L
题意:先给一个数字n表示有几辆火车。
然后给出两串数字,第一串表示火车进栈顺序,第二串表示火车出栈顺序。
算法:栈 或数组模拟栈。
PS:栈:后进先出
此题类似模板:lrj 《算法竞赛入门经典》 P91 ~ P93 例 6.1.2 铁轨
去年校赛就开始接触这道题目,开始是昨天叶写了这个代码,由于没有注意一个小条件老是WA让我改一下,最后是KB改了,我就按照昨天他们写的说的,重新写了一下,添加了下模拟数组的版本和备注挂在这儿吧。。。这几天都没怎么AC刚刚好一点的状态又颓废了。
/*C++ STL 版本*/ //Accepted 284 KB 0 ms C++ 988 B 2013-03-11 20:40:50 #include<cstdio> #include<cstring> #include<stack> using namespace std; const int maxn = 15; char strIn[maxn],strOut[maxn]; int getIn[maxn],getOut[maxn]; /* *将结果存入result数组 *result[i] = 1 则入栈 输出 in *result[i] = 0 则出栈 输出 out */ int result[maxn*2]; int n; int main() { while(scanf("%d", &n) != EOF) { scanf("%s%s", strIn, strOut); for(int i = 0; i < n; i++) { getIn[i] = strIn[i] - '0'; getOut[i] = strOut[i] - '0'; } memset(result, 0, sizeof(result)); //初始化 int a = 0; int b = 0; int k = 0; bool ok = true; //标示符初始化为真 stack<int> s; //建立空栈 while(b < n) { /* 如果当前进入的正好等于当前应当出去的 *注意条件 a < n表示还未进入完,否则会WA的很惨 */ if(a < n && getIn[a] == getOut[b]) { a++; b++; /* a++进栈 b++出栈 */ result[k++] = 1; k++; /* 对应标记in out */ } /* 如果栈非空并且栈顶正好符合当前应当出来的火车 */ else if(!s.empty() && s.top() == getOut[b]) { s.pop(); /*弹出栈顶 */ b++; /*火车出栈 */ k++; /*标记 out */ } else if(a < n) /*否则 火车进栈*/ { s.push(getIn[a++]); result[k++] = 1; /* 对应标记 in */ } else /* 否则无法满足条件 跳出循环*/ { ok = false; break; } } if(ok) { printf("Yes.\n"); for(int i = 0; i < k; i++) printf("%s\n", result[i] ? "in" : "out"); } else printf("No.\n"); printf("FINISH\n"); } return 0; }
/*C语言数组模拟栈版本*/ //Accepted 268 KB 0 ms C++ 962 B 2013-03-11 20:54:49 #include<cstdio> #include<cstring> #define maxn 15 char strIn[maxn],strOut[maxn]; int getIn[maxn],getOut[maxn]; int result[maxn*2]; int n; int main() { while(scanf("%d", &n) != EOF) { scanf("%s%s", strIn, strOut); for(int i = 0; i < n; i++) { getIn[i] = strIn[i] - '0'; getOut[i] = strOut[i] - '0'; } memset(result, 0, sizeof(result)); int a = 0; int b = 0; int k = 0; bool ok = true; int stack[maxn], top = 0; while(b < n) { if(a < n && getIn[a] == getOut[b]) { a++; b++; result[k++] = 1; k++; } else if(top && stack[top] == getOut[b]) { top--; b++; k++; } else if(a < n) { stack[++top] = getIn[a++]; result[k++] = 1; } else { ok = false; break; } } if(ok) { printf("Yes.\n"); for(int i = 0; i < k; i++) printf("%s\n", result[i] ? "in" : "out"); } else printf("No.\n"); printf("FINISH\n"); } return 0; }