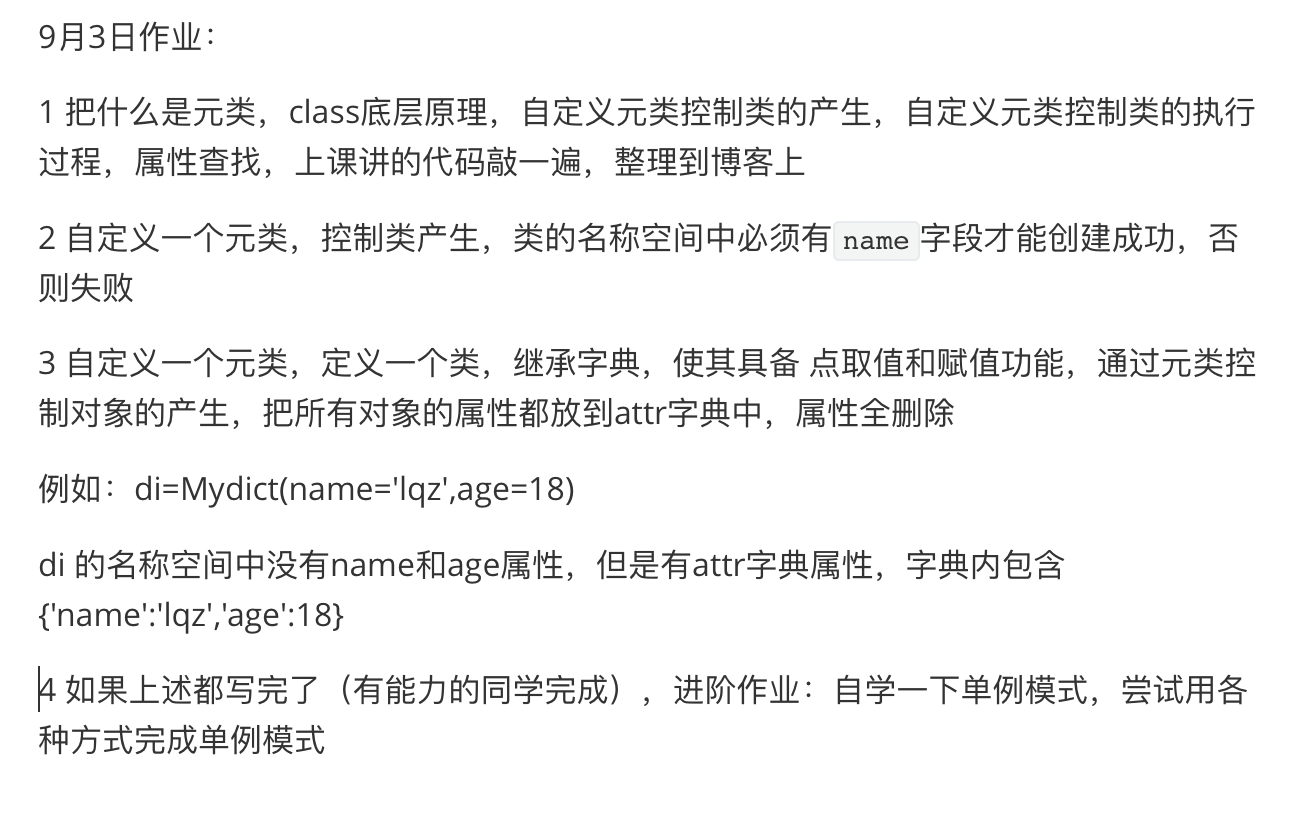
第一题
第二题
程序代码:
# 定义一个元类
class MyClass(type):
def __init__(self,name,bases,dic):
print(self.__dict__)
# 如果name 不在我们创建的类的名称空间中
if 'name' not in self.__dict__:
raise Exception('类名中没有name字段') # 报错
# 定义一个继承了元类的类其中包含name属性
class MyDemo(object,metaclass=MyClass):
name = 'forever'
def __init__(self,name):
self.name = name
# 定义一个继承元类的类其中不包含name属性
class Name(object,metaclass=MyClass):
def __init__(self,name):
self.name = name
运行结果:
继承了元类:
{'module': 'main', 'name': 'forever', 'init': <function MyDemo.init at 0x000001C319D408C8>, 'dict': <attribute 'dict' of 'MyDemo' objects>, 'weakref': <attribute 'weakref' of 'MyDemo' objects>, 'doc': None}
没有继承元类:
{'module': 'main', 'init': <function Name.init at 0x00000262B51908C8>, 'dict': <attribute 'dict' of 'Name' objects>, 'weakref': <attribute 'weakref' of 'Name' objects>, 'doc': None}
raise Exception('类名中没有name字段')
Exception: 类名中没有name字段
第三题
程序代码:
# 定义一个元类
class MyClass(type):
def __call__(self, *args, **kwargs):
obj = self.__new__(self,*args,**kwargs)
obj.__dict__['attr'] = kwargs
return obj
class MyDict(dict,metaclass=MyClass):
def __setattr__(self, key, value):
self[key] = value
def __getattr__(self, item):
kv = self[item]
return kv
di = MyDict(name='forever',age=18)
print(di.attr)
运行结果:
{'name': 'forever', 'age': 18}