一、Ajax请求
1、jQuery Ajax请求
let ajaxTimeOut = $.ajax({ //将网络请求事件赋值给变量ajaxTimeOut url: "/api_v1.1/apiPackage/knowKeyWord", type: "GET", dataType: "json", data: { "knowcontent": this.state.title + this.state.description, }, timeout: 2000, //通过timeout属性,设置超时时间 success: function (data) { console.log("获取关键字成功"); console.log(data); }.bind(this), error: function (xhr, status, err) { }.bind(this), complete: function (XMLHttpRequest, status) { //当请求完成时调用函数 if (status == 'timeout') {//status == 'timeout'意为超时,status的可能取值:success,notmodified,nocontent,error,timeout,abort,parsererror ajaxTimeOut.abort(); //取消请求 Modal.warning({ //超时提示:网络不稳定 title: '友情提示', content: '网络不稳定', }); } } });
2、jQuery Ajax请求
$.ajax({ url:"https://heidongbuhei.github.io/test/pages/"+pagrUrl, type:"get", data:{}, async:true, dataType:"html", beforeSend:function(){ $(".loading-box").show(); }, complete:function(){ $(".loading-box").hide(); }, success:function (data) { console.log(data); $(".change-part").html(data); }, error:function (data) { console.log(data.status); } })
跨域支持:
dataType: 'jsonp', crossDomain: true,
代码如下:
$(document).ready(function(){ $("button").click(function(){ $.ajax({ url:"http://t.weather.sojson.com/api/weather/city/101220101", type:"get", data:{}, async:true, dataType: 'jsonp', crossDomain: true, beforeSend:function(){ $(".loading-box").show(); }, complete:function(){ $(".loading-box").hide(); }, success:function (data) { console.log(data); $(".change-part").html(data); }, error:function (data) { console.log(data.status); } }) }); });
按钮:
<button>切换</button>
二、jQuery.extend
1、jQuery.extend类方法:Query本身的扩展方法。
<script> $.extend({ print1:function(name){ //print1是自己定义的函数名字,括号中的name是参数 console.log(name) } }); $.print1("坤") ; //调用时直接$.函数名(参数); </script>
2、jQuery.fn.extent()对象方法: jQuery 所选对象扩展方法。
<body> <input type="text"> <script> $.fn.extend({ getMax:function(a,b){ var result=a>b?a:b; console.log(result); } }); $("input").getMax(1,2); //调用时要$(标签名).函数名(); </script> </body>
3、一般情况下,jQuery的扩展方法写在自执行匿名函数中(原因:在js中是以函数为作用域的,在函数中写可以避免自己定义的函数或者变量与外部冲突)。
<script> (function(){ $.extent({ print1:function(){ console.log(123); } }) })(); </script>
三、JavaScript prototype 属性
prototype 属性允许您向对象添加属性和方法
注意: Prototype 是全局属性,适用于所有的 Javascript 对象。
(function($){ Date.prototype.Format = function (fmt) { //author: meizz var o = { "M+": this.getMonth() + 1, //月份 "d+": this.getDate(), //日 "h+": this.getHours(), //小时 "m+": this.getMinutes(), //分 "s+": this.getSeconds(), //秒 }; if (/(y+)/.test(fmt)) fmt = fmt.replace(RegExp.$1, (this.getFullYear() + "").substr(4 - RegExp.$1.length)); for (var k in o) if (new RegExp("(" + k + ")").test(fmt)) fmt = fmt.replace(RegExp.$1, (RegExp.$1.length == 1) ? (o[k]) : (("00" + o[k]).substr(("" + o[k]).length))); return fmt; } })(jQuery);
使用方式:
(new Date()).Format("yyyy-M-d h:m:s")
输出结果:
2018-06-07 23:26:22
四、JQuery关于(function($){ })(jQuery)匿名函数的解析
(function($){ })(jQuery)
:执行(jQuery)函数,并把jQuery对象作为实参,然后就会自动调用(function ($) {...}()这个匿名函数,并把实参传递给匿名函数,作为匿名函数的形参。(function ($) { alert("我执行了"); })(jQuery);
等同于
function callfunc($) { alert("我执行了"); } callfunc(jQuery);
效果演示:
事例演示
相当于定义了一个参数为info的匿名函数,并且执行("CoderZB")的同时,将CoderZB作为参数来,就会自动调用这个(function (info) {})匿名函数.最后面的()是调用匿名函数并将参数传递给匿名函数
(function (info) { alert(info); })("CoderZB");
其实就是这种形式。
function infomationFunc(info) { alert(info); }; infomationFunc("CoderZB");
执行结果
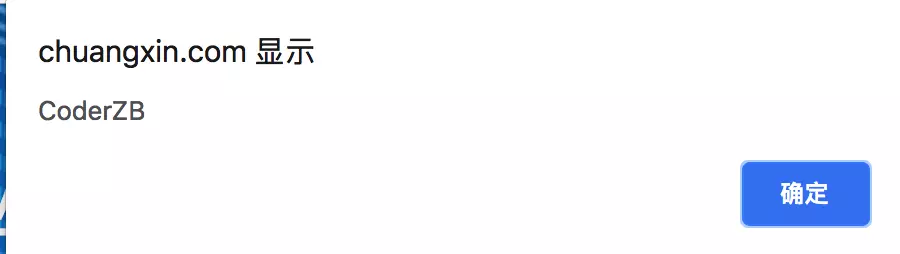