反射就是在运行的时候发现对象的相关信息。根据这些信息可以动态的执行对象的方法以及获取对象的属性所储存的值。
1,首先我们先建立一个类库工程MyDll,并新建一个类ReflectTest
代码如下:
using System;

namespace MyDll


{

/**//// <summary>
/// ReflectTest 的摘要说明。
/// </summary>

//接口
public interface ITest

{
int add();
}

public class ReflectTest : ITest

{
public String Write;
private String Writec;

public int add()

{
return 10;
}

public String Writea

{

get
{ return Write;}

set
{ Write = value;}
}

private String Writeb

{

get
{ return Writec;}

set
{ Writec = value;}
}

//构造函数
public ReflectTest()

{
this.Write = "Write";
this.Writec = "Writec";
}

//有参构造函数
public ReflectTest(string str1,string str2)

{
this.Write = str1;
this.Writec = str2;
}

public string PrintString(string s)

{
return "这是一个实例方法" + s;
}

private string PrintString2()

{
return "这是一个私有方法";
}

public static string Print(string s)

{
return "这是一个静态方法," + s;
}
public string PrintNoPara()

{
return "您使用的是无参数方法";
}

public static string PrintData(string s)

{
return s;
}

public static string PrintData(string s1, string s2)

{
return s1+s2;
}


}
}

编译后得到MyDll.dll文件
2,应用反射
using System;
using System.Reflection;

namespace cmdText


{

/**//// <summary>
/// 说明:反射学习
/// 程序:寻梦E.net
/// </summary>
public class Reflect2

{

delegate string TestDelegate(string value1);

public static void Main(string[] args)

{
Assembly ass = null ;
Type type = null ;
object obj = null;

string assemblyName = "MyDll";
string className = "MyDll.ReflectTest";
try

{
//以下三种方法都可以
//ass = Assembly.LoadFile(System.Environment.CurrentDirectory+"/MyDll.dll");
//ass = Assembly.LoadFrom(System.Environment.CurrentDirectory+"/MyDll.dll");
ass = Assembly.Load(assemblyName);
}
catch

{
Console.Write("无法找到程序集!");
return;
}
//获取类型
type = ass.GetType(className);
//type = Type.GetType(className);//效果同上
//生成一个类实例,无参构造函数
obj = ass.CreateInstance(className);
//obj = Activator.CreateInstance(type);//效果同上

//**************************方法调用*************************************
//反射实例方法

Console.WriteLine("*******方法调用**************************************");
MethodInfo method = type.GetMethod("PrintString");

string s = (string)method.Invoke(obj,new string[]
{"寻梦E.net"});
Console.WriteLine(s);

//反射私有方法
method = type.GetMethod("PrintString2",BindingFlags.Instance | BindingFlags.NonPublic | BindingFlags.Public);
s = (string)method.Invoke(obj,null);
Console.WriteLine(s);

//反射静态方法
method = type.GetMethod("Print");

s = (string)method.Invoke(null,new string[]
{"寻梦E.net"});
Console.WriteLine(s);

//反射无参方法
method = type.GetMethod("PrintNoPara");
s = (string)method.Invoke(obj,null);
Console.WriteLine(s);

//反射重载了的静态方法

Type[] objParam =
{typeof(string),typeof(string)};
method = type.GetMethod("PrintData",objParam );

s = (string)method.Invoke(null,new object[]
{"hello!"," 寻梦E.net"});
Console.WriteLine(s);

Console.WriteLine("*******属性,域的赋值与访问***********************");


//*****************属性,域的赋值与访问***************************************
//公有属性
PropertyInfo pi1 = type.GetProperty("Writea");
pi1.SetValue(obj, "公有属性:Writea", null);
Console.WriteLine(pi1.GetValue(obj,null));

//私有属性
PropertyInfo pi2 = type.GetProperty("Writeb", BindingFlags.Instance | BindingFlags.NonPublic | BindingFlags.Public);
pi2.SetValue(obj, "私有属性:Writeb", null);
Console.WriteLine(pi2.GetValue(obj, null));
//域
FieldInfo fi1 = type.GetField("Write");
Console.WriteLine(fi1.GetValue(obj));


Console.WriteLine("*******类型,模块,方法,构造函数等信息的获取*****");



//******************类型,模块,方法,构造函数等信息的获取******************************************
//有参构造函数实例化
//string[] classParams = {"lsm","sky"};
//object obj2 = Activator.CreateInstance(type,classParams);

foreach(Type t in ass.GetTypes())

{
Console.WriteLine("类型:"+t.Name );
}
foreach(Module m in ass.GetModules())

{
Console.WriteLine("模块:"+m.Name);
}

MethodInfo[] methods = type.GetMethods();
foreach (MethodInfo m in methods)

{
Console.WriteLine("方法"+m.Name); //显示所有的共有方法
}

FieldInfo[] fields = type.GetFields();
foreach (FieldInfo f in fields)

{
Console.WriteLine("字段信息:"+f.Name);
}

PropertyInfo[] propertys = type.GetProperties();
foreach( PropertyInfo p in propertys)

{
Console.WriteLine("属性:"+p.Name);
}

ConstructorInfo[] ci1 = type.GetConstructors();
foreach (ConstructorInfo ci in ci1)

{
Console.WriteLine("构造函数:"+ci.ToString()); //获得构造函数的形式
}

//*******************接口***************************************
Console.WriteLine("*******接口*****************************************");

MyDll.ITest obj1 = (MyDll.ITest)ass.CreateInstance(className);//这里必须是实现接口的类名,因为接口不能实例化
//MyDll.ReflectTest obj2= (MyDll.ReflectTest)ass.CreateInstance(className);
//典型的工厂模式
int i = obj1.add();
Console.WriteLine(i);


//*****************委托*******************************************

TestDelegate myDelegate = (TestDelegate)Delegate.CreateDelegate(typeof(TestDelegate), obj, "PrintString");
//动态创建委托的简单例子
Console.WriteLine(myDelegate(" 动态调用委托"));


//*****************获得解决方案的所有Assembly********************

Assembly[] assemblys = AppDomain.CurrentDomain.GetAssemblies();
//遍历显示每个Assembly的名字
foreach (object var in assemblys)

{
Console.WriteLine("所有的Assembly的名字:"+var.ToString());
}
Console.Read();



}


}
}
运行效果图如下:
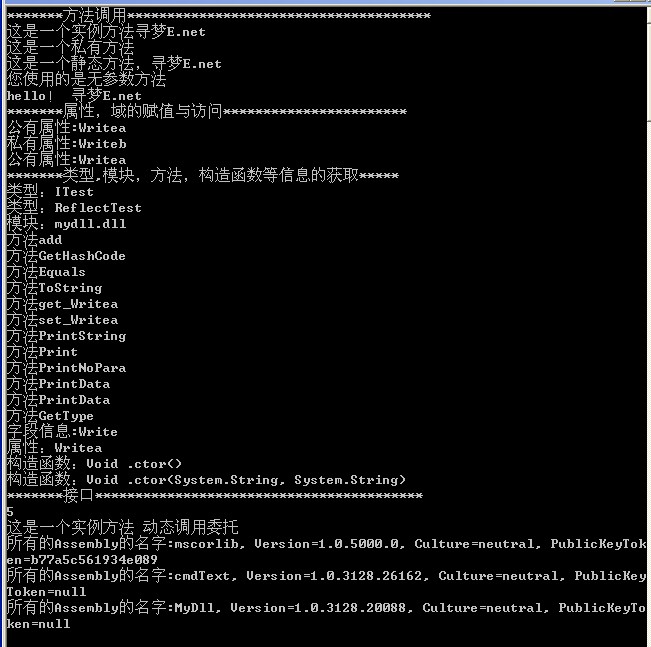