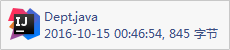
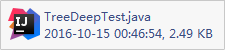
// 测试类
package test;
import java.util.*;
/**
* @Created with IntelliJ IDEA
* @User: boa
* @Date: 2016/10/15
* @Time: 0:10
* @Version: 0.0.1
* @Description: 计算多级集合/树/部门树的深度.
*/
public class TreeDeepTest {
public static void main(String[] args) {
List<Dept> depts1 = new ArrayList<Dept>();
depts1.add(new Dept());
List<Dept> depts2 = new ArrayList<Dept>();
depts2.add(new Dept());
depts2.add(new Dept());
List<Dept> depts3 = new ArrayList<Dept>();
depts3.add(new Dept());
depts3.add(new Dept());
depts3.add(new Dept());
Dept dept = new Dept(); //当前为第一层
//dept.setParent(new Dept());
dept.setSon(depts1); //第二层
dept.getSon().get(0).setSon(depts2); //第三层
dept.getSon().get(0).getSon().get(1).setSon(depts3); //第四层
TreeDeepTest test = new TreeDeepTest();
System.out.println(dept.hashCode());
System.out.println("深度: " + test.getDeep(dept));
}
/**
* 计算深度.
*
* @param dept
* @return
*/
public int getDeep(Dept dept) {
Set<Integer> pool = new HashSet<Integer>();
//默认当前所处深度为1
calcDeep(dept, 1, pool);
//返回池中记录的最深深度.
return pool.isEmpty() ? 1 : Collections.max(pool);
}
/**
* 遍历树的每个分支, 通过当前深度遍历延伸, 每次深度下潜一层都需要将深度+1,并存放深度记录池中.
*
* @param dept
* @param currentDeep
* @param pool
*/
public void calcDeep(Dept dept, int currentDeep, Set<Integer> pool) {
List<Dept> deptSon = dept.getSon();
if (deptSon != null && !deptSon.isEmpty()) {
currentDeep++; //如果当前的部门判断含有子部门,则深度+1
pool.add(currentDeep);
for (Dept d : deptSon) {
System.out.print(getLevel(currentDeep) + dept.hashCode());
System.out.println();
calcDeep(d, currentDeep, pool); //递归,传递当前的深度进去
}
}
}
/**
* 打印层级效果
*
* @param num
* @return
*/
public String getLevel(int num) {
String mark = "";
for (int i = 1; i < num; i++) {
mark += "|--";
}
return mark;
}
}
// 部门类
package test;
import java.util.List;
/**
* @Created with IntelliJ IDEA
* @User: boa
* @Date: 2016/10/15
* @Time: 0:10
* @Version: 0.0.1
* @Description: 模拟部门类
*/
public class Dept implements Cloneable {
private Long id;
private Dept parent;
private List<Dept> son;
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public Dept getParent() {
return parent;
}
public void setParent(Dept parent) {
this.parent = parent;
}
public List<Dept> getSon() {
return son;
}
public void setSon(List<Dept> son) {
this.son = son;
}
@Override
protected Object clone() throws CloneNotSupportedException {
return super.clone();
}
}