方式三、实现Callable接口,理解即可
- 实现Callable接口,需要返回值类型
- 重写call方法,需要抛出异常
- 创建目标对象
- 创建执行服务:
ExecutorService ser =Executors.newFixedThreadPool(3);
- 提交执行:
Future<Boolean> result1 = ser.submit(t1);
- 获取结果:
boolean r1 = result1.get();
- 关闭服务:
ser.shutdownNow();
Callable的好处:
- 可以定义返回值
- 可以抛出异常
- 执行比较麻烦,需要创建一个执行服务,通过服务提交,然后获取结果,最后得关闭服务
1 package com.thread.demo02; 2 3 import org.apache.commons.io.FileUtils; 4 5 import java.io.File; 6 import java.io.IOException; 7 import java.net.URL; 8 import java.util.concurrent.*; 9 10 //线程创建方式三:实现callable接口 11 public class TestCallable implements Callable<Boolean> { 12 13 private String url; //网络图片地址 14 private String name;//保存的文件名 15 16 public TestCallable(String url, String name) { 17 this.url = url; 18 this.name = name; 19 } 20 21 //下载图片线程的执行体 22 @Override 23 public Boolean call() { 24 WebDownloader webDownloader = new WebDownloader(); 25 webDownloader.downloader(url, name); 26 System.out.println("下载了文件名:" + name); 27 return true; 28 } 29 30 public static void main(String[] args) throws ExecutionException, InterruptedException { 31 TestCallable t1 = new TestCallable("http://a3.att.hudong.com/35/34/19300001295750130986345801104.jpg", "1.jpg"); 32 TestCallable t2 = new TestCallable("http://file02.16sucai.com/d/file/2014/1028/f445fe013d861d9e99fd70a0734efd8a.jpg", "2.jpg"); 33 TestCallable t3 = new TestCallable("http://img.tuzhaozhao.com/2017/10/25/1f33b154b2da3f51_300x300.jpg", "3.jpg"); 34 35 //创建执行服务 36 ExecutorService ser = Executors.newFixedThreadPool(3); 37 //提交执行 38 Future<Boolean> r1 = ser.submit(t1); 39 Future<Boolean> r2 = ser.submit(t2); 40 Future<Boolean> r3 = ser.submit(t3); 41 //获取结果 42 boolean rs1 = r1.get(); 43 boolean rs2 = r2.get(); 44 boolean rs3 = r3.get(); 45 //关闭服务 46 ser.shutdownNow(); 47 48 } 49 50 } 51 52 //下载器 53 class WebDownloader { 54 //下载方法 55 public void downloader(String url, String name) { 56 try { 57 FileUtils.copyURLToFile(new URL(url), new File(name)); 58 } catch (IOException e) { 59 e.printStackTrace(); 60 System.out.println("IO异常,downloader方法出现问题"); 61 } 62 } 63 } 64 65 结果: 66 下载了文件名:2.jpg 67 下载了文件名:1.jpg 68 下载了文件名:3.jpg
三张图片:
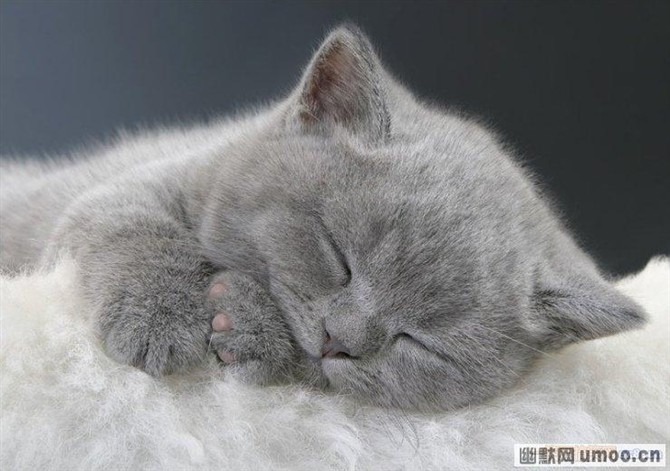