题目给出一个图, 问图中所有的“#”能否恰好独立的组成十字架(一个#只能在一个十字架中)一开始用dfs写的好混乱。。 后面发现从左上到右下,如果一个#满足正好在中间且四周可以消去,那就一定要消去,否则就NO。这样枚举一遍就好了。
题目:
Fox Ciel has a board with n rows and n columns. So, the board consists of n × n cells. Each cell contains either a symbol '.', or a symbol '#'.
A cross on the board is a connected set of exactly five cells of the board that looks like a cross. The picture below shows how it looks.
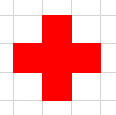
Ciel wants to draw several (may be zero) crosses on the board. Each cross must cover exactly five cells with symbols '#', and any cell with symbol '#' must belong to some cross. No two crosses can share a cell.
Please, tell Ciel if she can draw the crosses in the described way.
The first line contains an integer n (3 ≤ n ≤ 100) — the size of the board.
Each of the next n lines describes one row of the board. The i-th line describes the i-th row of the board and consists of n characters. Each character is either a symbol '.', or a symbol '#'.
Output a single line with "YES" if Ciel can draw the crosses in the described way. Otherwise output a single line with "NO".
5
.#...
####.
.####
...#.
.....
YES
4
####
####
####
####
NO
6
.#....
####..
.####.
.#.##.
######
.#..#.
YES
6
.#..#.
######
.####.
.####.
######
.#..#.
NO
3
...
...
...
YES
In example 1, you can draw two crosses. The picture below shows what they look like.
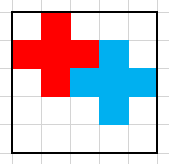
In example 2, the board contains 16 cells with '#', but each cross contains 5. Since 16 is not a multiple of 5, so it's impossible to cover all.
代码:
1 #include <iostream> 2 #include <algorithm> 3 #include <cstdio> 4 #include <vector> 5 #include <queue> 6 #include <stack> 7 #include <cmath> 8 #include <cstring> 9 #include <string> 10 #include <cstdlib> 11 using namespace std; 12 #define LL lolng long 13 14 int n; 15 char G[105][105]; 16 void init() 17 { 18 scanf("%d",&n); 19 getchar(); 20 21 for(int i=0;i<n;i++) 22 { 23 for(int j=0;j<n;j++) 24 { 25 scanf("%c",&G[i][j]); 26 } 27 getchar(); 28 } 29 } 30 31 bool inmap(int x,int y) 32 { 33 if(x>=0&&x<n &&y>=0&&y<n) 34 return true; 35 return false; 36 } 37 38 int dx[]= {1,-1,0,0}; 39 int dy[] ={0,0,1,-1}; 40 41 42 int main() 43 { 44 init(); 45 int cnt=0; 46 int mm=0; 47 for(int i=0;i<n;i++) 48 { 49 for(int j=0;j<n;j++) 50 { 51 if( G[i][j] =='#') 52 { 53 mm++; 54 cnt =1; 55 for(int p=0;p<4;p++) 56 { 57 if(inmap(i+dx[p],j+dy[p])&&G[i+dx[p]][j+dy[p]]=='#') 58 cnt++; 59 } 60 if(cnt==5) 61 { 62 G[i][j]='.'; 63 for(int p=0;p<4;p++) 64 { 65 G[i+dx[p]][j+dy[p]]='.'; 66 } 67 } 68 //flag = dfs(i,j); 69 } 70 } 71 72 } 73 cnt=0; 74 for(int i=0;i<n;i++) 75 { 76 for(int j=0;j<n;j++) 77 { 78 if(G[i][j] =='#') 79 { 80 cnt=1; 81 break; 82 } 83 } 84 } 85 if(!cnt||mm==0)cout<<"YES"<<endl; 86 else 87 cout<<"NO"<<endl; 88 return 0; 89 }