You are given an n x n 2D matrix representing an image.
Rotate the image by 90 degrees (clockwise).
Note:
You have to rotate the image in-place, which means you have to modify the input 2D matrix directly. DO NOT allocate another 2D matrix and do the rotation.
Example 1:
Given input matrix = [ [1,2,3], [4,5,6], [7,8,9] ], rotate the input matrix in-place such that it becomes: [ [7,4,1], [8,5,2], [9,6,3] ]
Example 2:
Given input matrix = [ [ 5, 1, 9,11], [ 2, 4, 8,10], [13, 3, 6, 7], [15,14,12,16] ], rotate the input matrix in-place such that it becomes: [ [15,13, 2, 5], [14, 3, 4, 1], [12, 6, 8, 9], [16, 7,10,11] ]
题意:题目就是说把一个n×n的矩阵旋转90°。
我的算法测试的时候都是正确的,但测试样本都是12345....这样的,而不是LeetCode给的那种测试数据:
{
{10,151,33,143,165,11,13,28,69,159,194,16,108,53},
{8,67,159,59,114,13,24,121,16,13,71,122,158,187},
{85,160,147,52,116,181,1,86,194,167,71,24,131,26},
{41,1,81,7,69,1,67,184,15,49,66,32,19,94},
{111,134,87,154,100,39,11,21,177,13,65,132,138,93},
{114,74,121,112,76,7,120,147,9,189,92,25,-1,116},
{15,19,15,127,154,59,42,59,55,55,38,190,25,60},
{83,164,154,2,0,80,116,77,44,41,181,10,187,35},
{189,187,108,161,163,80,49,123,141,93,139,1,149,134},
{148,131,-1,37,57,111,40,58,148,157,92,194,156,35},
{161,104,71,111,97,180,77,21,65,128,145,163,178,46},
{122,88,181,75,24,138,113,82,54,155,97,7,73,191},
{158,186,31,81,96,59,193,150,44,76,172,66,161,79},
{35,144,126,158,37,112,38,18,55,109,58,66,69,156}
};
这样运行时就发现出现了一些问题。。。
我先说说我的算法思路:就是按照旋转的意思,把正方形的矩阵中对应位置上的数进行交换来实现旋转,如图:
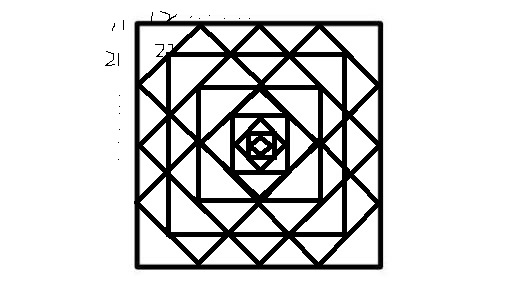
每个位置上的与根据计算得到的对应位置上的进行交换,并逐渐向内层递进。
代码:
#include <iostream> #include <vector> using namespace std; class Solution { public: void rotate(vector<vector<int> >& matrix) { int row = matrix.size(); for(int i = 0; (row%2 == 0) ? (row > 5 ? i < row/2 : i <= row/2 ) : i <= row/2; i++) { for(int j = 0; (row%2 == 0 && row > 5) ? j < row/2 : (row < 5 ? j <= i : j < row/2); j++) { int cont = (row%2 == 0) ? matrix[row/2 - 1][row/2] : matrix[j][j]; if((i != j && cont == matrix[j][i])) break; swap(matrix[i][j], matrix[j][row - 1 - i]); swap(matrix[i][j], matrix[row - 1 - j][i]); swap(matrix[row - 1 - j][i], matrix[row - 1 - i][row - 1 - j]); } } } }; #define N 14 int main() { int nums[][N] = { {10,151,33,143,165,11,13,28,69,159,194,16,108,53}, {8,67,159,59,114,13,24,121,16,13,71,122,158,187}, {85,160,147,52,116,181,1,86,194,167,71,24,131,26}, {41,1,81,7,69,1,67,184,15,49,66,32,19,94}, {111,134,87,154,100,39,11,21,177,13,65,132,138,93}, {114,74,121,112,76,7,120,147,9,189,92,25,-1,116}, {15,19,15,127,154,59,42,59,55,55,38,190,25,60}, {83,164,154,2,0,80,116,77,44,41,181,10,187,35}, {189,187,108,161,163,80,49,123,141,93,139,1,149,134}, {148,131,-1,37,57,111,40,58,148,157,92,194,156,35}, {161,104,71,111,97,180,77,21,65,128,145,163,178,46}, {122,88,181,75,24,138,113,82,54,155,97,7,73,191}, {158,186,31,81,96,59,193,150,44,76,172,66,161,79}, {35,144,126,158,37,112,38,18,55,109,58,66,69,156} }; vector<vector<int> > matrix(N, vector<int> (N)); Solution so; for(int i = 0; i < N; i++) { for(int j = 0; j < N; j++) { matrix[i][j] = nums[i][j]; } } for(int i = 0; i < N; i++) { for(int j = 0; j < N; j++) { cout << matrix[i][j] << ' '; } cout << endl; } cout << endl; so.rotate(matrix); for(int i = 0; i < N; i++) { for(int j = 0; j < N; j++) { cout << matrix[i][j] << ' '; } cout << endl; } return 0; }
但并没有通过...至少给的测试数据没通过的原因是,我的算法最多进行到层数的一半,也就是左上角的分块矩阵部分,所以无法进行到120,49,55,123这几个的位置...但对于12345...这样的14*14的矩阵却很正确的实现了。代码或许看着有些复杂,其实时间复杂度也不怎么样,O(n^2)。
题目来源:Rotate Image