package array;
import java.util.Arrays;
/*
冒泡排序
*/
public class Demo07 {
final static int[] result={4,564,54,54,5,4,54,54,54,54,54,65,46,58,7,54,67,8,4,564,899,2,2,46,4,0};
public static void main(String[] args) {
int[] a = {1, 2, 15, 4, 12, 45, 2, 55, 54, 12, 22, 45, 99, 231, 4, 2, 55};
int[] sort = sort(a);
System.out.println("冒泡排序:" + Arrays.toString(sort));//[231, 99, 55, 55, 54, 45, 45, 22, 15, 12, 12, 4, 4, 2, 2, 2, 1]
int[] ints = a_sort(a);
System.out.println("冒泡排序:" + Arrays.toString(ints));//[1, 2, 2, 2, 4, 4, 12, 12, 15, 22, 45, 45, 54, 55, 55, 99, 231]
int[] res = sort2(result);
System.out.println("冒泡排序:"+Arrays.toString(res));//[0, 2, 2, 4, 4, 4, 4, 5, 7, 8, 46, 46, 54, 54, 54, 54, 54, 54, 54, 54, 58, 65, 67, 564, 564, 899]
}
public static int[] sort2(int[] array){
int temp =0;
for (int i = 0; i < array.length-1; i++) {
for (int j = 0; j < array.length-1-i; j++) {
if (array[j+1]<array[j]){
temp=array[j];
array[j]=array[j+1];
array[j+1]=temp;
}
}
}
return array;
}
// 从大到小
public static int[] sort(int[] array) {
int temp = 0;
for (int i = 0; i < array.length - 1; i++) {
for (int j = 0; j < array.length - 1 - i; j++) {
// 后一个数大于当前数据 ,需交换;
if (array[j + 1] > array[j]) {
temp = array[j];
array[j] = array[j + 1];
array[j + 1] = temp;
}
}
}
return array;
}
// 从小到大
public static int[] a_sort(int[] array) {
int temp = 0;
for (int i = 0; i < array.length - 1; i++) {
boolean flag = false;//优化;最后一轮不用走;
for (int j = 0; j < array.length - 1 - i; j++) {
// 后一个数大于当前数据 ,需交换;
if (array[j + 1] < array[j]) {
temp = array[j];
array[j] = array[j + 1];
array[j + 1] = temp;
flag = true;
}
}
if (flag == false) {
break;
}
}
return array;
}
}
运行结果
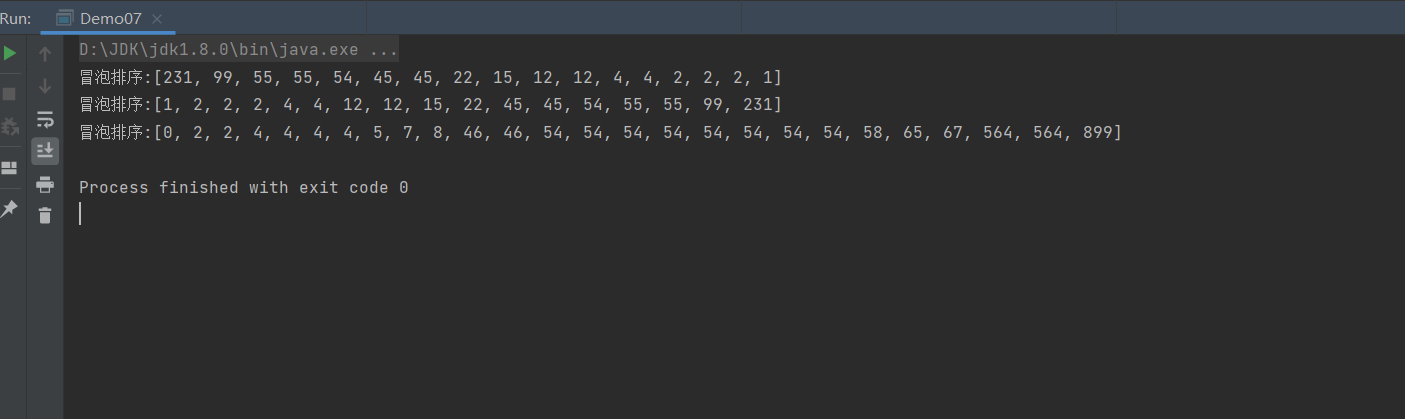