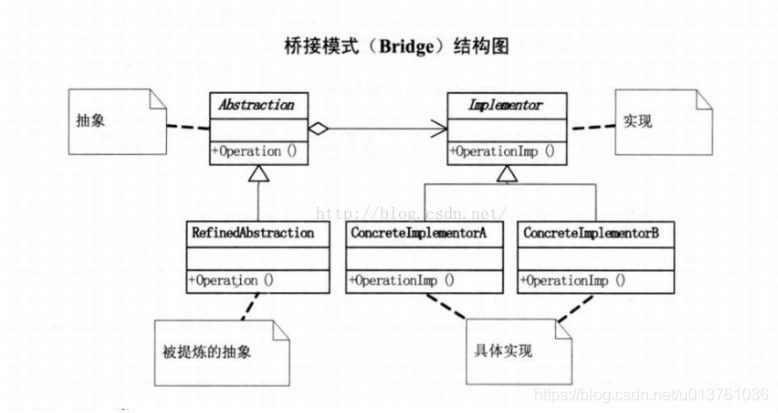
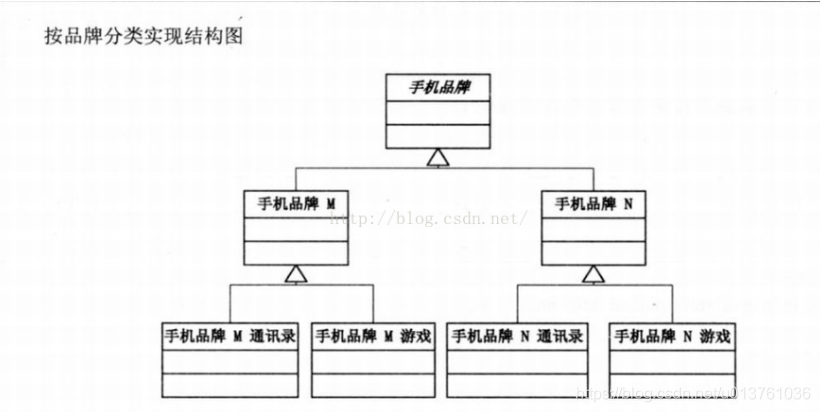
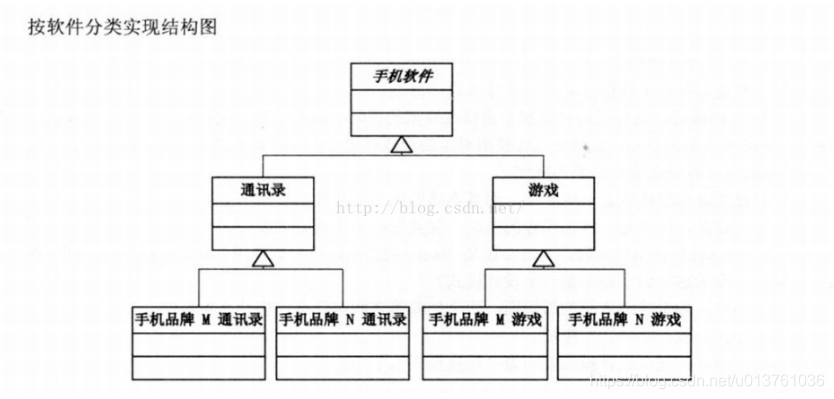
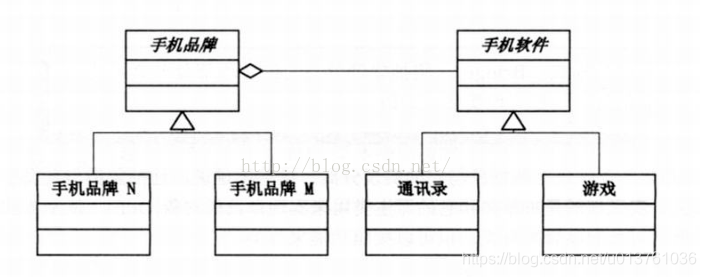
#pragma once
#include "stdafx.h"
#include<set>
#include<string>
#include<iostream>
using namespace std;
/*
设计模式-桥接模式(Bridge)
将抽象部分与它的实现部分分离,使他们都可以独立地变化。
*/
class CImplementor {
public:
virtual void Operation() = 0;
};
class CConcreteImplementorA :public CImplementor {
public:
void Operation() {
cout << "Execution method A" << endl;
}
};
class CConcreteImplementorB :public CImplementor {
public:
void Operation() {
cout << "Execution method B" << endl;
}
};
class CAbstraction {
protected:
CImplementor * m_pImplementor;
public:
CAbstraction() {
m_pImplementor = NULL;
}
void SetImplementor(CImplementor *pImplementor) {
m_pImplementor = pImplementor;
}
virtual void Operation() = 0;
};
class CRefinedAbstraction :public CAbstraction {
public:
void Operation() {
if (m_pImplementor != NULL) {
m_pImplementor->Operation();
}
}
};
int main() {
CAbstraction *pAb = new CRefinedAbstraction();
CConcreteImplementorA *pcA = new CConcreteImplementorA();
CConcreteImplementorB *pcB = new CConcreteImplementorB();
pAb->SetImplementor(pcA);
pAb->Operation();
pAb->SetImplementor(pcB);
pAb->Operation();
getchar();
return 0;
}
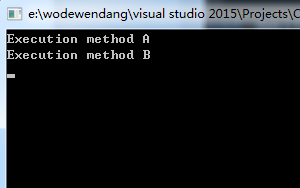