1.新建maven工程
2.导入依赖,并使用shade将所需的依赖打入jar包
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>ForJmeter</groupId>
<artifactId>com.csj2018</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<!-- https://mvnrepository.com/artifact/org.testng/testng -->
<dependency>
<groupId>org.testng</groupId>
<artifactId>testng</artifactId>
<version>6.14.3</version>
</dependency>
<!-- https://mvnrepository.com/artifact/junit/junit -->
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.hamcrest/hamcrest-all -->
<dependency>
<groupId>org.hamcrest</groupId>
<artifactId>hamcrest-all</artifactId>
<version>1.3</version>
</dependency>
<!-- https://mvnrepository.com/artifact/io.rest-assured/rest-assured -->
<dependency>
<groupId>io.rest-assured</groupId>
<artifactId>rest-assured</artifactId>
<version>4.0.0</version>
</dependency>
<dependency>
<groupId>io.rest-assured</groupId>
<artifactId>json-path</artifactId>
<version>4.0.0</version>
</dependency>
<dependency>
<groupId>io.rest-assured</groupId>
<artifactId>xml-path</artifactId>
<version>4.0.0</version>
</dependency>
<!-- JSON对象转换,把对象转换成JSON字符串或JSON Object -->
<dependency>
<groupId>io.rest-assured</groupId>
<artifactId>json-schema-validator</artifactId>
<version>4.1.2</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.fasterxml.jackson.core/jackson-databind -->
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.9.8</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.fasterxml.jackson.core/jackson-core -->
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-core</artifactId>
<version>2.9.8</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.fasterxml.jackson.core/jackson-annotations -->
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-annotations</artifactId>
<version>2.9.8</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.apache.poi/poi -->
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.0.1</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.apache.poi/poi-ooxml -->
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>4.0.1</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.apache.jmeter/ApacheJMeter_core -->
<dependency>
<groupId>org.apache.jmeter</groupId>
<artifactId>ApacheJMeter_core</artifactId>
<version>3.3</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.apache.jmeter/ApacheJMeter_java -->
<dependency>
<groupId>org.apache.jmeter</groupId>
<artifactId>ApacheJMeter_java</artifactId>
<version>3.3</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-shade-plugin</artifactId>
<version>2.1</version>
<executions>
<execution>
<phase>package</phase>
<goals>
<goal>shade</goal>
</goals>
<configuration>
<artifactSet>
<includes>
<include>io.rest-assured</include>
</includes>
</artifactSet>
</configuration>
</execution>
</executions>
</plugin>
</plugins>
</build>
</project>
3.编写Java类
import io.restassured.response.Response;
import org.apache.jmeter.config.Arguments;
import org.apache.jmeter.protocol.java.sampler.AbstractJavaSamplerClient;
import org.apache.jmeter.protocol.java.sampler.JavaSamplerContext;
import org.apache.jmeter.samplers.SampleResult;
import static io.restassured.RestAssured.given;
public class TestJmeterDemo extends AbstractJavaSamplerClient {
String corpid;
String corpsecret;
@Override
public void setupTest(JavaSamplerContext context){
System.out.println("测试开始");
corpid = context.getParameter("corpid");
corpsecret = context.getParameter("corpsecret");
}
@Override
public SampleResult runTest(JavaSamplerContext javaSamplerContext) {
SampleResult result = new SampleResult();
result.sampleStart();
//同@Test
String url = "https://qyapi.weixin.qq.com/cgi-bin/gettoken";
Response res = given().param("corpid",corpid).param("corpsecret",corpsecret).get(url).prettyPeek();
String accessToken = res.getBody().jsonPath().getString("access_token");
if(res.statusCode() == 200){
result.setSuccessful(true);
result.setResponseData(res.getBody().prettyPrint(),"UTF-8");
}else{
result.setSuccessful(false);
}
result.sampleEnd();
return result;
}
@Override
public void teardownTest(JavaSamplerContext context){
System.out.println("测试结束");
}
/**
* 参数化
*/
@Override
public Arguments getDefaultParameters() {
Arguments arguments = new Arguments();
arguments.addArgument("corpid","corpid.value");//value可以为空
arguments.addArgument("corpsecret","");
return arguments;
}
}
4.打包
#打包
mvn clean package
#将打出的jar移到指定目录
mv com.csj2018-1.0-SNAPSHOT.jar ~/otherApp/apache-jmeter-3.3/lib/ext/
5.启动jmeter,添加java请求,运行
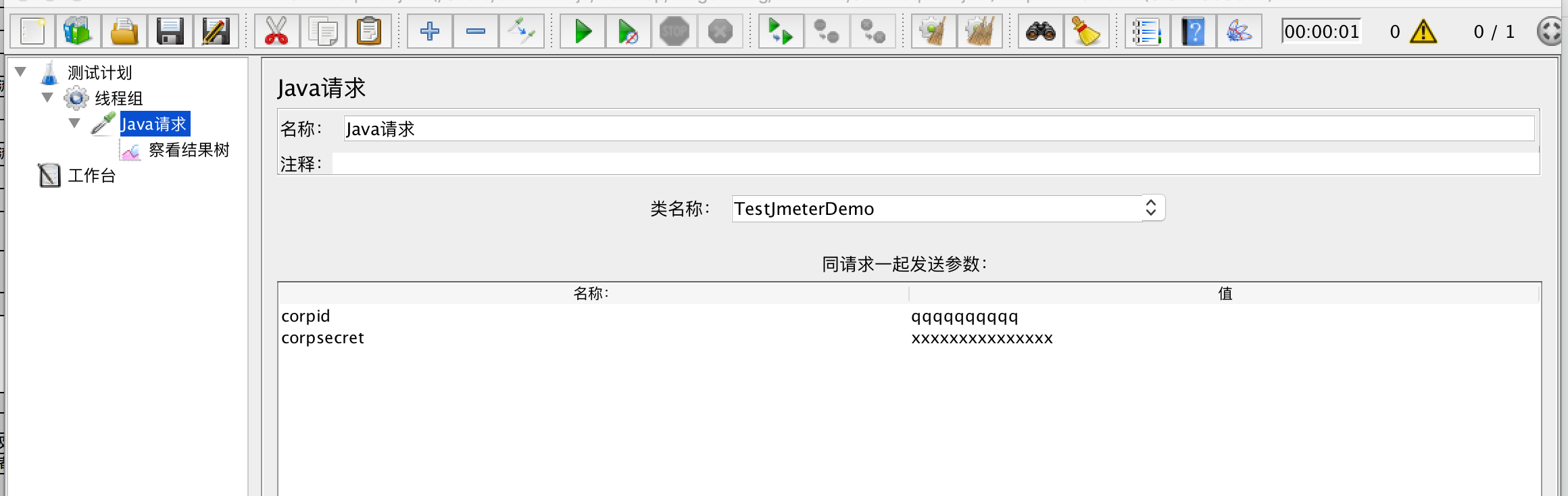
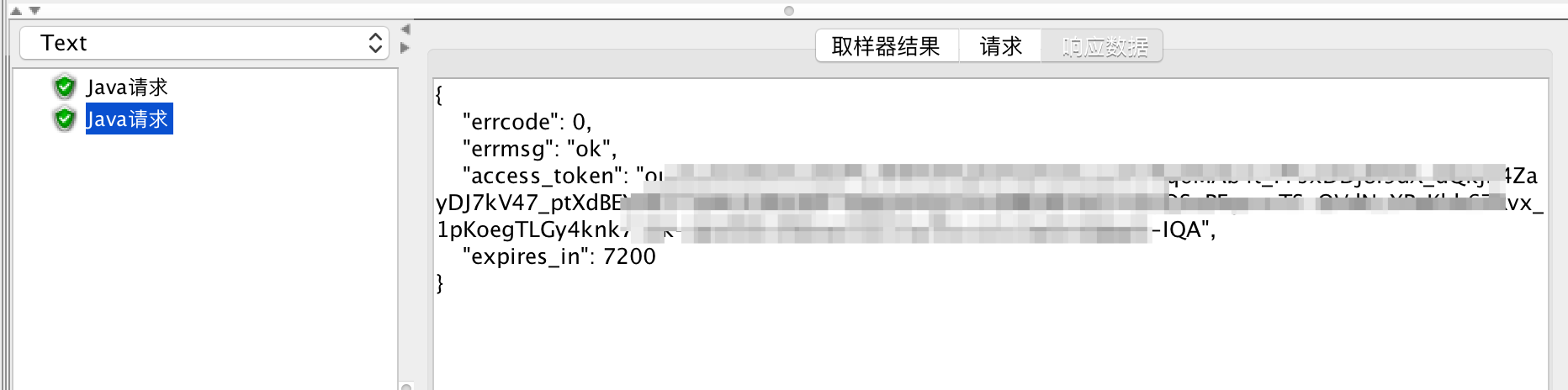
问题
1.打的jar包提示某些类不存在,如Uncaught Exception java.lang.NoClassDefFoundError: org/ccil/cowan/tagsoup/Parser. See log file for details.
原因:如果是三方依赖的包,那需要打在最后的jar里,百度搜索assembly的maven。只需要在inclueds里加入这个包即可
<configuration>
<artifactSet>
<includes>
<include>io.rest-assured</include>
<inclued>org.ccil.cowan.tagsoup</inclued><!--缺失的jar包-->
</includes>
</artifactSet>
</configuration>