1. 创建接口ShopService.java
package com.csj2018.o2o.service;
import java.io.File;
import com.csj2018.o2o.entity.Shop;
import com.csj2018.o2o.dto.ShopExecution;
public interface ShopService {
ShopExecution addShop(Shop shop,File shopImg);
}
2. 创建店铺操作异常类
为什么会用RuntimeException,而不是Exception呢?
当我们的程序仅当跑出RuntimeException或者继承RuntimeException的异常类时,事物才得以终止并回滚。如果是Exception,事物就无法终止和回滚package com.csj2018.o2o.exceptions;
public class ShopOperationException extends RuntimeException{
/**
*
*/
private static final long serialVersionUID = -4379928435508967105L;
public ShopOperationException(String msg) {
super(msg);
}
}
3. 创建接口的实现类
package com.csj2018.o2o.service.impl;
import java.io.File;
import java.util.Date;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import com.csj2018.o2o.dao.ShopDao;
import com.csj2018.o2o.dto.ShopExecution;
import com.csj2018.o2o.entity.Shop;
import com.csj2018.o2o.enums.ShopStateEnum;
import com.csj2018.o2o.execptions.ShopOperationException;
import com.csj2018.o2o.service.ShopService;
import com.csj2018.o2o.util.ImageUtil;
import com.csj2018.o2o.util.PathUtil;
@Service
public class ShopServiceImpl implements ShopService {
@Autowired
private ShopDao shopDao;
@Override
@Transactional
public ShopExecution addShop(Shop shop,File shopImg) {
//控制判断,shop是不是包含必须的一些值
if(shop == null) {
return new ShopExecution(ShopStateEnum.NUll_SHOP);
}
//增加对Shop其他引入类非空的判断
try {
//给店铺信息赋初始值
shop.setEnableStatus(0);
shop.setCreateTime(new Date());
shop.setLastEditTime(new Date());
//添加店铺信息
int effectedNum = shopDao.insertShop(shop);
if(effectedNum <= 0) {
throw new ShopOperationException("店铺创建失败");
}else {
if(shopImg != null) {
//存储图片
try {
addShopImage(shop,shopImg);
}catch (Exception e) {
throw new ShopOperationException("addShopImg error:"+e.getMessage());
}
//更新店铺的图片信息
effectedNum = shopDao.updateShop(shop);
if(effectedNum <= 0) {
throw new ShopOperationException("更新图片地址失败");
}
}
}
}catch(Exception e) {
throw new ShopOperationException("addShop error:"+e.getMessage());
}
return new ShopExecution(ShopStateEnum.CHECK,shop);
}
private void addShopImage(Shop shop, File shopImg) {
// 获取shop图片目录的相对路径
String dest = PathUtil.getShopImagePath(shop.getShopId());
String shopImgAddr = ImageUtil.generateThumbnail(shopImg, dest);
shop.setShopImg(shopImgAddr);
}
}
4. 创建单元测试类
package com.csj2018.o2o.service;
import static org.junit.Assert.assertEquals;
import java.io.File;
import java.util.Date;
import org.junit.Test;
import org.springframework.beans.factory.annotation.Autowired;
import com.csj2018.o2o.BaseTest;
import com.csj2018.o2o.dto.ShopExecution;
import com.csj2018.o2o.entity.Area;
import com.csj2018.o2o.entity.PersonInfo;
import com.csj2018.o2o.entity.Shop;
import com.csj2018.o2o.entity.ShopCategory;
import com.csj2018.o2o.enums.ShopStateEnum;
public class ShopServiceTest extends BaseTest{
@Autowired
private ShopService shopService;
@Test
public void testAddShop() {
Shop shop = new Shop();
PersonInfo owner = new PersonInfo();
Area area = new Area();
ShopCategory shopCategory = new ShopCategory();
owner.setUserId(1L);
area.setAreaId(2);
shopCategory.setShopCategoryId(1L);
shop.setOwner(owner);
shop.setArea(area);
shop.setShopCategory(shopCategory);
shop.setShopName("测试de店铺1");
shop.setShopDesc("店铺描述1");
shop.setShopAddr("测试路1号1");
shop.setPhone("1234567891");
shop.setPriority(5);
shop.setCreateTime(new Date());
shop.setEnableStatus(ShopStateEnum.CHECK.getState());
shop.setAdvice("审核中");
File shopImg = new File("/Users/chenshanju/Downloads/cat.jpg");
ShopExecution se = shopService.addShop(shop, shopImg);
assertEquals(ShopStateEnum.CHECK.getState(), se.getState());
}
}
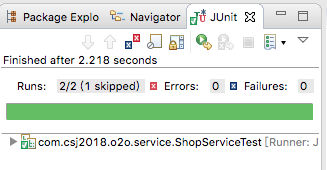