1.非对称加密
非对称加密就是加密和解密使用的不是相同的密钥
- 方法1:
* 加密:用自己的私钥加密,然后发送给对方:encrypt(privateKeyA, message)->s
* 解密:对方用自己的公钥解密:decrypt(publicKeyA, s)->message - 方法2:
* 加密:用对方的公钥加密,然后发送给对方:ecrypt(publicKeyB, message)->s
* 解密:对方用自己的私钥解密:decrypt(privateKeyB, s) -> message
非对称加密典型算法:RSA(3个发明人名字的缩写)
2.代码示例
package com.testList;
import org.bouncycastle.jcajce.provider.symmetric.ARC4;
import javax.crypto.Cipher;
import java.security.*;
import java.security.spec.PKCS8EncodedKeySpec;
import java.security.spec.X509EncodedKeySpec;
import java.util.Base64;
public class RSAKeyPair {
PrivateKey sk;//私钥
PublicKey pk;//公钥
//构造方法1:生成公钥/私钥对
public RSAKeyPair() throws GeneralSecurityException {
KeyPairGenerator kpGen = KeyPairGenerator.getInstance("RSA");
kpGen.initialize(1024);//初始化,密钥长度为1024位
KeyPair kp = kpGen.generateKeyPair();//生成KeyPair
this.sk = kp.getPrivate();//通过getPrivate()生成私钥
this.pk = kp.getPublic();//通过getPublic()生成公钥
}
//构造方法2:从已保存的字节中(例如,读取文件)恢复公钥/私钥
public RSAKeyPair(byte[] pk, byte[] sk) throws GeneralSecurityException{
KeyFactory kf = KeyFactory.getInstance("RSA");
//恢复公钥
X509EncodedKeySpec pkSpec = new X509EncodedKeySpec(pk);
this.pk = kf.generatePublic(pkSpec);
//恢复私钥
PKCS8EncodedKeySpec skSpec = new PKCS8EncodedKeySpec(sk);
this.sk = kf.generatePrivate(skSpec);
}
//把私钥导出为字节数组
public byte[] getPrivateKey(){
return this.sk.getEncoded();
}
//把公钥导出为字节数组
public byte[] getPublicKey(){
return this.pk.getEncoded();
}
//用公钥加密
public byte[] encrypt(byte[] message) throws GeneralSecurityException{
Cipher cipher = Cipher.getInstance("RSA");
cipher.init(Cipher.ENCRYPT_MODE,this.pk);
return cipher.doFinal(message);
}
//用公钥解密
public byte[] decrpt(byte[] input) throws GeneralSecurityException{
Cipher cipher = Cipher.getInstance("RSA");
cipher.init(Cipher.DECRYPT_MODE,this.sk);
return cipher.doFinal(input);
}
public static void main(String[] args) throws Exception{
byte[] plain = "Hello,使用RSA非对称加密算法对数据进行加密!".getBytes("utf-8");
//创建公钥和私钥对
RSAKeyPair rsa = new RSAKeyPair();
//加密
byte[] encrypted = rsa.encrypt(plain);
System.out.println("encrypted:"+ Base64.getEncoder().encodeToString(encrypted));
byte[] decrypted = rsa.decrpt(encrypted);
System.out.println("decryted:"+new String(decrypted));
//保存公钥和私钥
byte[] sk = rsa.getPrivateKey();
byte[] pk = rsa.getPublicKey();
System.out.println("pk:"+Base64.getEncoder().encodeToString(pk));
System.out.println("sk"+Base64.getEncoder().encodeToString(sk));
//重新恢复公钥和私钥
RSAKeyPair rsa2 = new RSAKeyPair(pk,sk);
//加密
byte[] encrypted2 = rsa2.encrypt(plain);
System.out.println("encrypted2:"+Base64.getEncoder().encodeToString(encrypted2));
//解密
byte[] decrypted2 = rsa2.decrpt(encrypted2);
System.out.println("decrypted2:"+new String(decrypted2));
}
}
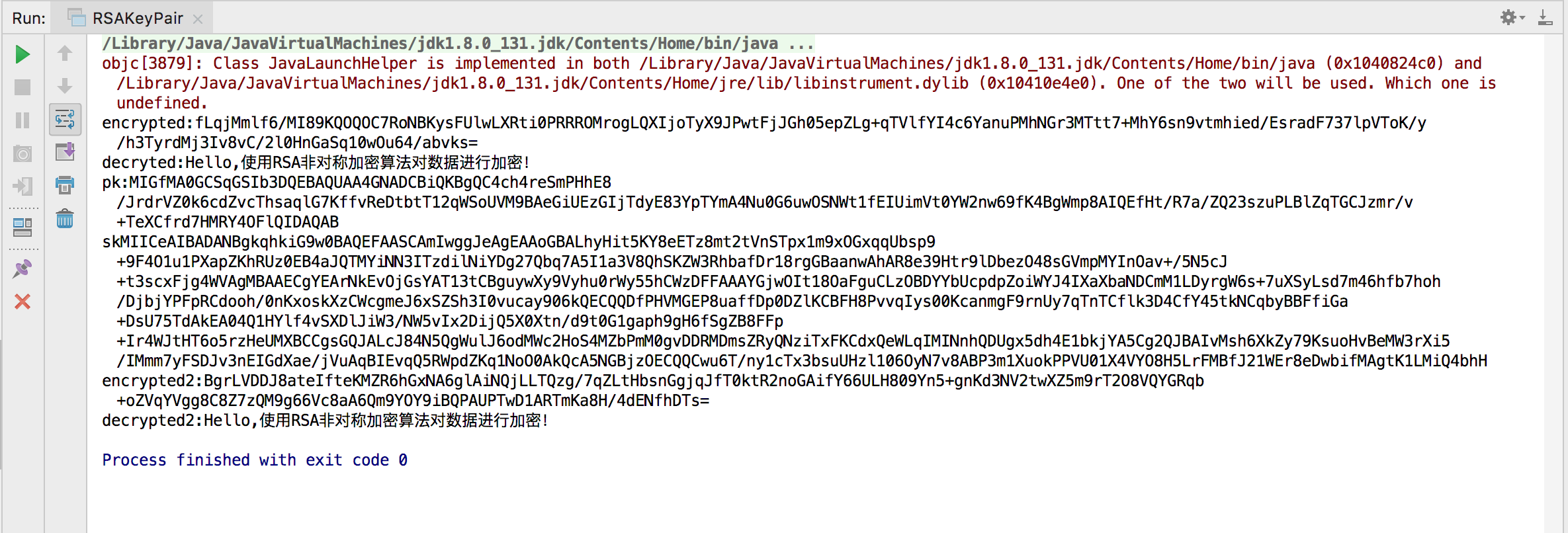
3.非对称加密算法优缺点:
- 优点
* 对称加密需要协商密钥,而非对称加密可以安全地公开各自的公钥
* N个人之间通信:- 使用非对称加密只需要N个密钥对。每个人值管理自己的密钥对
- 使用对称加密需要N*(N-1)/2个密钥。每个人需要管理N-1个密钥
- 缺点:速度慢
4.总结:
- 非对称加密就是加密和解密使用的不是相同的密钥
- 只有同一个公钥/私钥对才能正常加密/解密
- 只使用非对称加密算法不能防止中间人攻击