1捕获异常
1.1 finally语句保证有无错误都会执行
try{...}catch (){...}finally{...}
- 使用try...catch捕获异常
- 可能发生异常的语句放在try{...}中
- 使用catch捕获对应的Exception及其子类
1.2 捕获多个异常
try{...} catch() {...} catch(){...}finally{..}
使用多个catch子句:
- 每个catch捕获对应的Exception及其子类
- 从上到下匹配,匹配到某个catch后不再继续匹配
注意:catch的顺序非常重要,子类必须写在前面:
try{...} catch(IOException e){...} catch(UnsupportedEncodingException e){...}
UnsupportedEncodingException是IOExcetion的子类,这样UnsupportedEncodingException永远都不会被捕获到
import java.io.*;
import java.lang.String;
public class Main {
public static void main(String[] args) {
process("abc");
process("0");
}
static void process(String s) {
try{
int n = Integer.parseInt(s);
int m = 100 / n;
}catch(NumberFormatException e){
System.out.println(e);
System.out.println("Bad input");
}catch (ArithmeticException e){
System.out.println(e);
System.out.println("Bad input");
}finally {
System.out.println("end process");
}
}
}
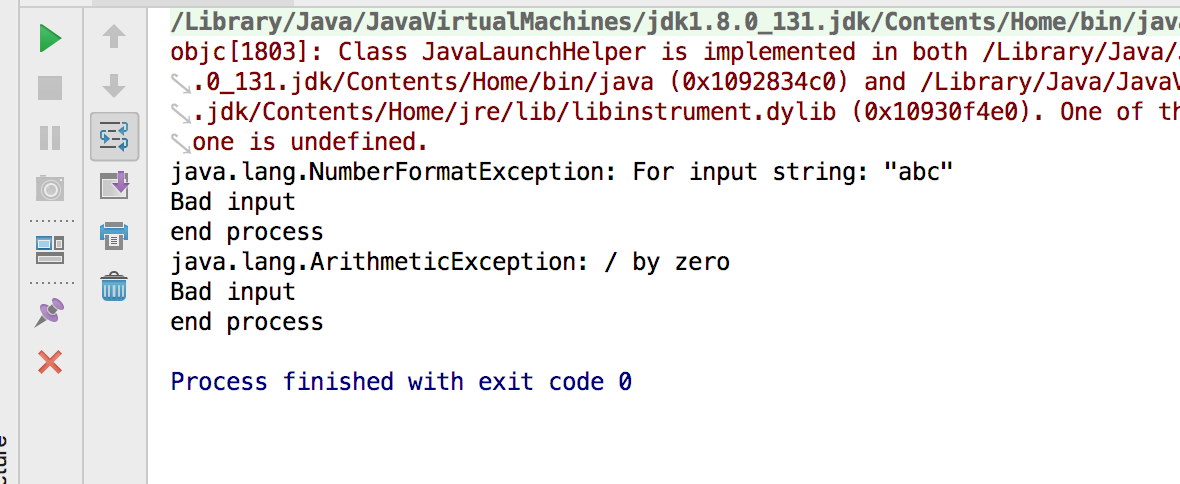
1.3 multi-catch
如果某些异常的处理逻辑相同:
- 不存在继承关系,必须编写多条catch子句,可以用 " | " 表示多种Exception
import java.lang.String;
public class Main {
public static void main(String[] args) {
process("abc");
process("0");
}
static void process(String s) {
try{
int n = Integer.parseInt(s);
int m = 100 / n;
}catch(NumberFormatException|ArithmeticException e){
System.out.println(e);
System.out.println("Bad input");
}finally {
System.out.println("end process");
}
}
}
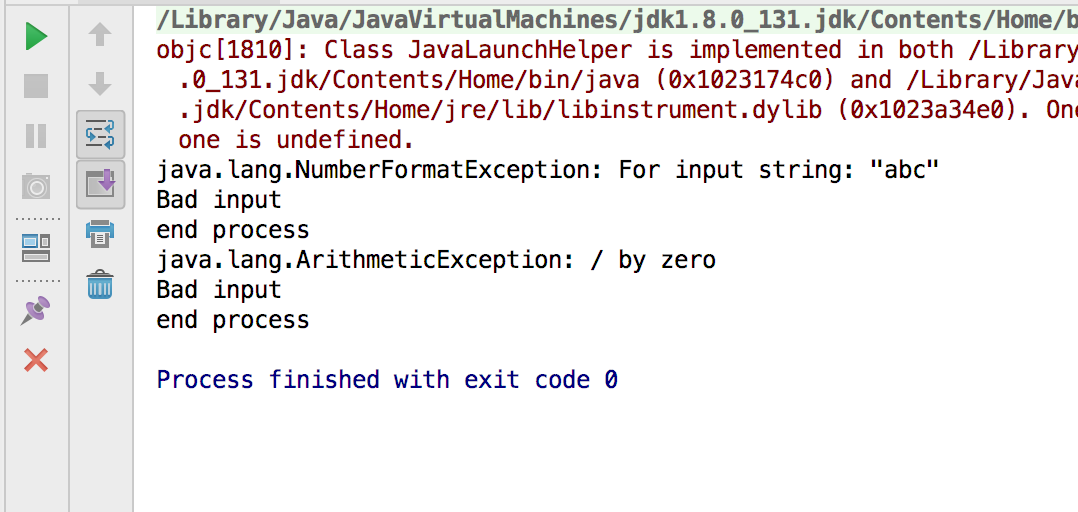
2.总结
- catch子句的匹配顺序非常重要,子类必须放在前面
- finally子句保证有无异常都会执行
- finally是可选的
- catch可以匹配多个非继承关系的异常(JDK >= 1.7)