1.计算机运行中的错误
在计算机程序运行的过程中,错误总会出现,不可避免的
- 用户输入错误
- 读写文件错误
- 网络错误、内存耗尽、无法连接打印机不可
String s = "abc";
int n = Integer.parseInt(s);
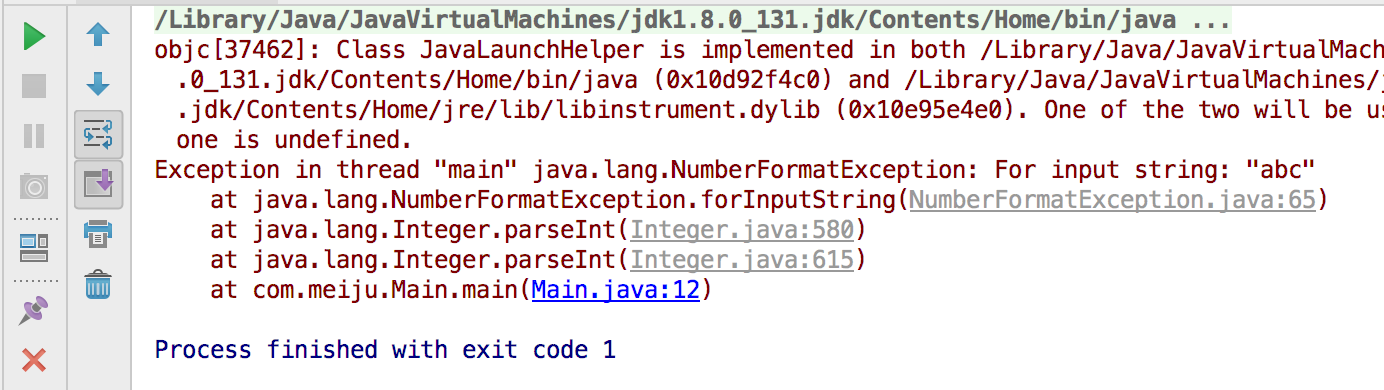
2.1Java的异常体系
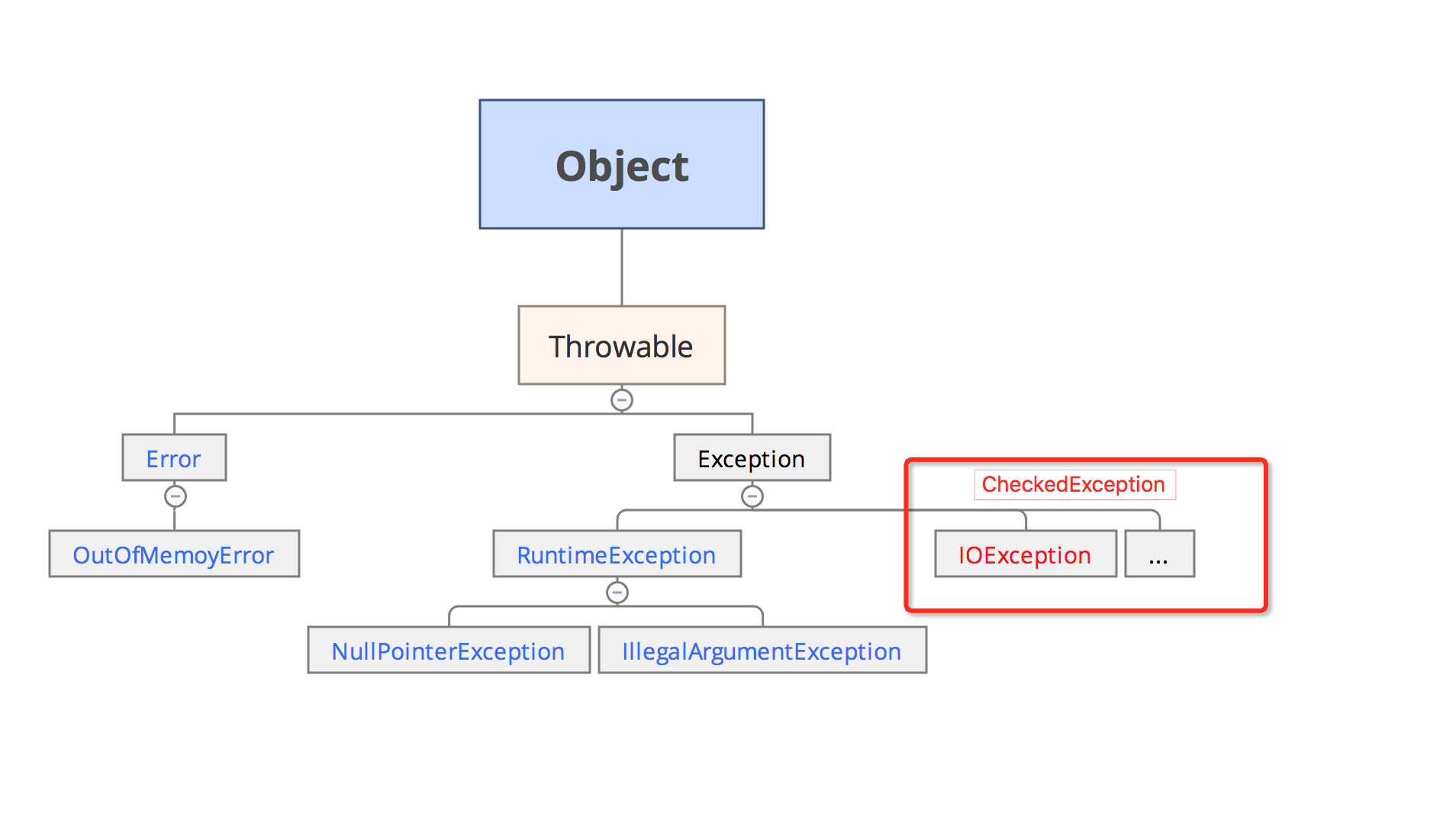
- Exception是发生了运行时逻辑错误,应该捕获异常并处理:
* 有些错误是可以预期可能出现的,如IOException,NumberFormatException...,此时应该捕获并处理这些错误
* 有些错误是程序存在bug导致的,如NullPointException,IndexOutOfBoundsException...,此时是程序本身有问题,应该修复程序
3捕获异常
Java程序中
- 用try{...}catch(){...}捕获异常
- 可能发生异常的语句放在try{...}中
- 使用catch捕获对应的Exception及其子类
import java.io.*;
import java.lang.String;
public class Main {
public static void main(String[] args) {
test("UTF-8");
test("ABC");
}
static void test(String encoding){
try{
"test".getBytes(encoding);
System.out.println("OK.");
}catch (UnsupportedEncodingException e){
System.out.println("failed");
System.out.println(e);
}
}
}
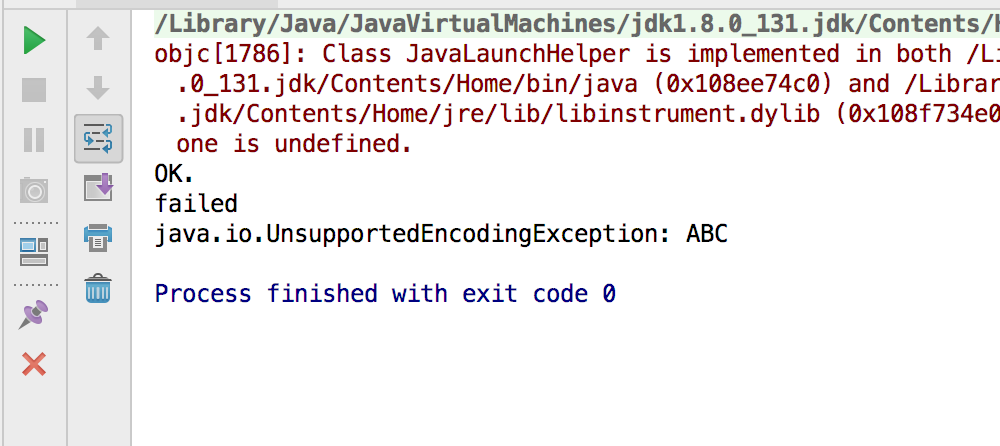
public class Main {
public static void main(String[] args) throws UnsupportedEncodingException{
test("UTF-8");
test("ABC");
}
static void test(String encoding) throws UnsupportedEncodingException{
"test".getBytes(encoding);
System.out.println("OK.");
}
}
<img src="https://img2018.cnblogs.com/blog/1418970/201901/1418970-20190128215638621-598124900.png" width="500" />
# 5.总结:
* Java使用异常来表示错误,并通过try{...} catch {...}捕获异常
* Java的异常是class,并且从Throwable继承
* Error是无需捕获的严重错误
* Exception是应该捕获的可处理的错误
* RuntimeException无需强制捕获,非RuntimeException(CheckedException)需强制捕获,或者用throws声明。