1.Math
Math提供了数学计算的静态方法
序号 | 方法 | 描述 |
---|---|---|
1 | abs() | 返回参数的绝对值。Math.abs(-9)//9 |
2 | ceil() | 返回大于等于( >= )给定参数的的最小整数。Math.ceil(4.5)//5.0 |
3 | floor() | 返回小于等于(<=)给定参数的最大整数 。ath.floor(4.5)//4.0 |
4 | rint() | 以0.5为界返回与参数最接近的整数。返回类型为double。Math.rint(4.5)//4.0 Math.rint(4.51)//5.0 |
5 | round() | 它表示四舍五入,算法为 Math.floor(x+0.5),即将原来的数字加上 0.5 后再向下取整,所以,Math.round(11.5) 的结果为12,Math.round(-11.5) 的结果为-11。 |
6 | min() | 返回两个参数中的最小值。Math.min(1,3)//1 |
7 | max() | 返回两个参数中的最大值。Math.max(1.3,6)//6 |
8 | exp() | 返回自然数底数e的参数次方。Math.exp(1)//2.718281828459045 |
9 | log() | 返回参数的自然数为底数的对数值。Math.log(2.718281828459045)//1.0; Math.log10(10) //1.0 返回参数以10为底数的对数值。 |
10 | pow() | 返回第一个参数的第二个参数次方。Math.pow(2,10)//1024 |
11 | sqrt() | 求参数的算术平方根。Math.sqrt(2)//1.4142135623730951 |
12 | sin() | 求指定double类型参数的正弦值。90度角的正弦值Math.sin(Math.PI/2)//1.0 |
13 | cos() | 求指定double类型参数的余弦值。0度角的余弦值:Math.cos(0)//1.0 |
14 | tan() | 求指定double类型参数的正切值。45度角的正弦值:Math.tan(Math.PI/4)//0.9999999999999999 |
15 | asin() | 求指定double类型参数的反正弦值。0.5的反正弦值是30度Math.toDegrees(Math.asin(0.5)) |
16 | acos() | 求指定double类型参数的反余弦值。0.5的反余弦值是60度Math.toDegrees(Math.acos(0.5)) |
17 | atan() | 求指定double类型参数的反正切值。1的反正切值是45度Math.toDegrees(Math.atan(1)) |
18 | atan2() | 将笛卡尔坐标转换为极坐标,并返回极坐标的角度值。 |
19 | toDegrees() | 将参数转化为角度。 |
20 | toRadians() | 将角度转换为弧度。Math.toRadians(45)/Math.PI//0.25PI |
21 | PI | Math.PI |
22 | E | Math.E 自然数2.718281828459045 |
2.random() 随机数
Math.random()生成一个随机数:
- 0 <= 随机数< 1
- 可用于生成某个区间的随机数
double x1 = Math.random();
long MIN = 1000;
long MAX = 9000;
double x2 = Math.random() * (MAX - MIN) + MIN;
System.out.println(x1+" "+(long) x2);
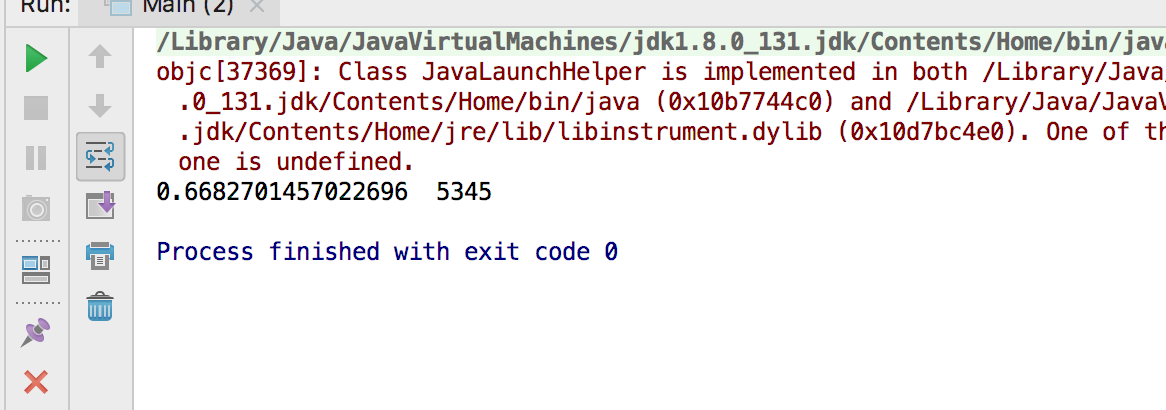
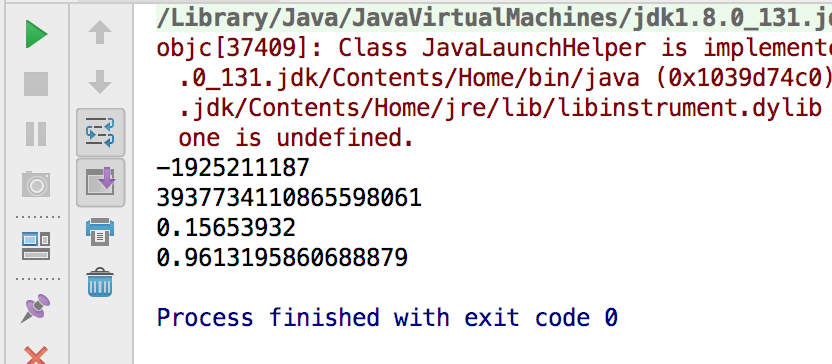
//根据种子号码,生成固定序列的随机数
System.out.println("Hello java");//Hello world
Random r = new Random(12345);
for(int i = 0;i<10;i++){
System.out.print(r.nextInt(100)+" ");
}
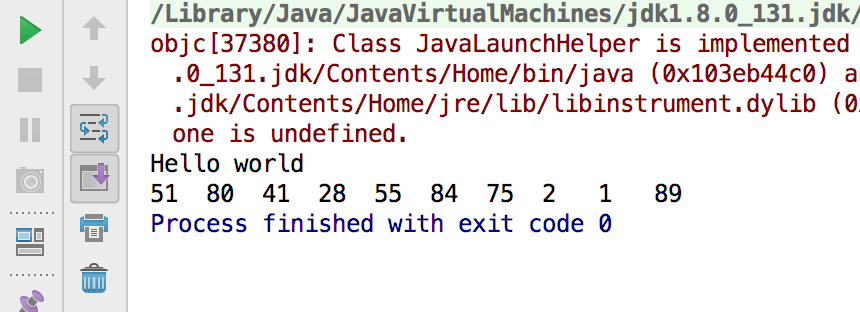
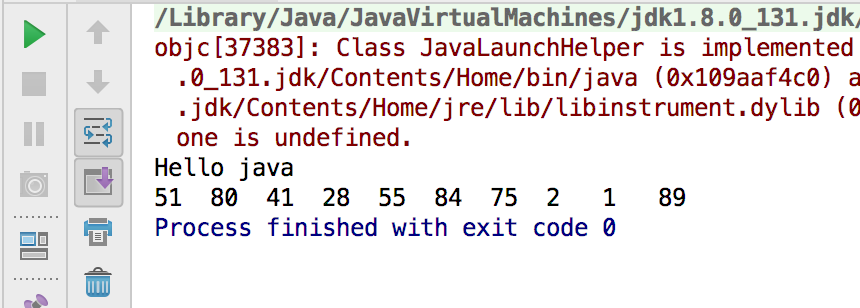
3.安全随机数
SecureRandom用来创建安全的随机数
SecureRandom sr = new SecureRandom();
for(int i = 0;i < 10;i++){
System.out.print(sr.nextInt(100)+" ");
}
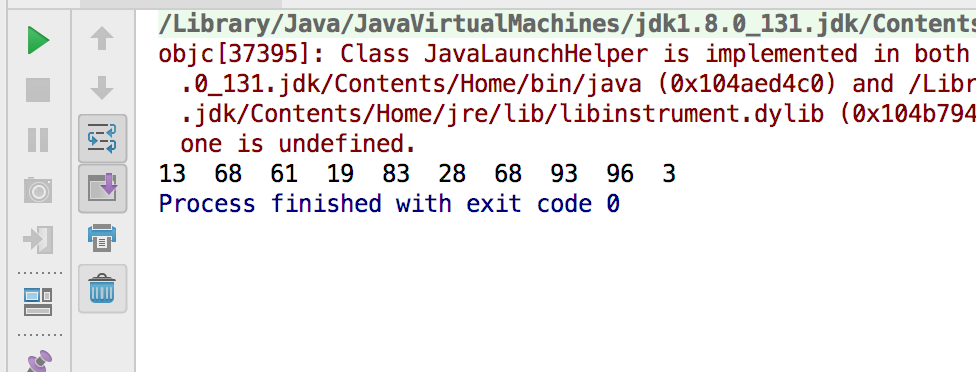
4.BigInteger
用任意多个int[] 来表示非常大的整数
BigInteger bi = new BigInteger("1234567890");
System.out.println(bi.pow(5));
5.Bigdecimal表示任意精度的浮点数
BigDecimal bd = new BigDecimal("123.10");
System.out.println(bd.multiply(bd));
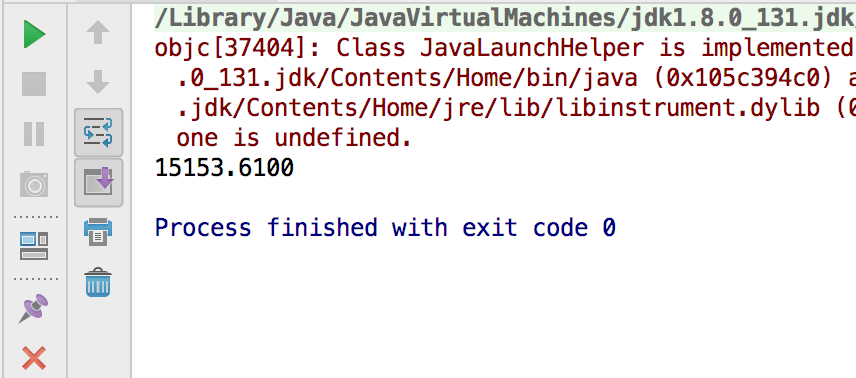
总结
- Math:数学运算
- Random:生成伪随机数
- SecureRandom:生成安全的随机数
- BigInteger:表示任意大小的证书
- BigDecimal:表示任意精度的浮点数
- BigInteger和BigDecimal都继承自Number
更多参考菜鸟(http://www.runoob.com/java/java-number.html){http://www.runoob.com/java/java-number.html}