进程:计算机程序是磁盘中可执行的二进制或其他类型数据。他们只有在被读取到内存中、被操作系统调用的时候才开始它们的生命周期。进程是程序的一次执行,每个进程都有自己的地址空间、内存、数据栈,以及其他记录其运行轨迹的辅助数据。操作系统管理在其上面运行的所有进程,并为这些进程公平的分配时间。
线程:所有的线程都运行在同一个进程中,共享相同的运行环境。
1.单线程与多进程
避免使用thread模块,原因是thread不支持守护进程。当主进程退出时,所有的子线程不管他们是否在工作,都会被强行退出。threading模块支持守护线程。
from time import ctime,sleep,time
import threading
def music(list):
for i in list:
print("I was listening to %s! %s"%(i,ctime()))
sleep(2)
def movie(m,loop):
for i in range(loop):
print("I was at the %s! %s" % (m, ctime()))
sleep(5)
if __name__=="__main__":
start=time()
music(["寂静之声","一生何求"])
movie("阿甘正传",2)
end=time()
used_time = int(end-start)
print("Time consuming: %s seconds" % used_time)
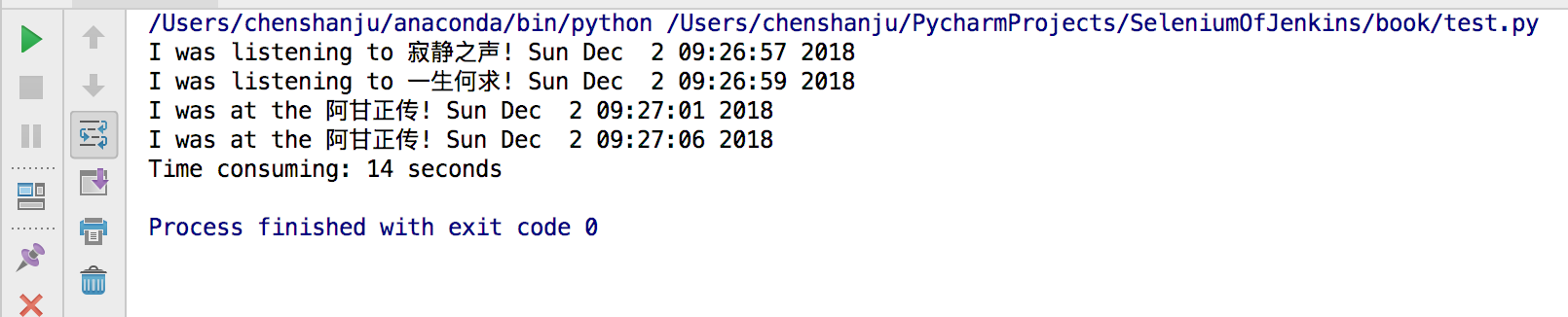
from time import ctime,sleep,time
import threading
def music(list):
for i in list:
print("I was listening to %s! %s"%(i,ctime()))
sleep(2)
def movie(m,loop):
for i in range(loop):
print("I was at the %s! %s" % (m, ctime()))
sleep(5)
theads=[]
t1=threading.Thread(target=music,args=(["寂静之声","一生何求"],))
theads.append(t1)
t2=threading.Thread(target=movie,args=("阿甘正传",2))
theads.append(t2)
if __name__=="__main__":
start=time()
for i in theads:
i.start()
for i in theads:
i.join()
end=time()
used_time = int(end-start)
print("Time consuming: %s seconds" % used_time)
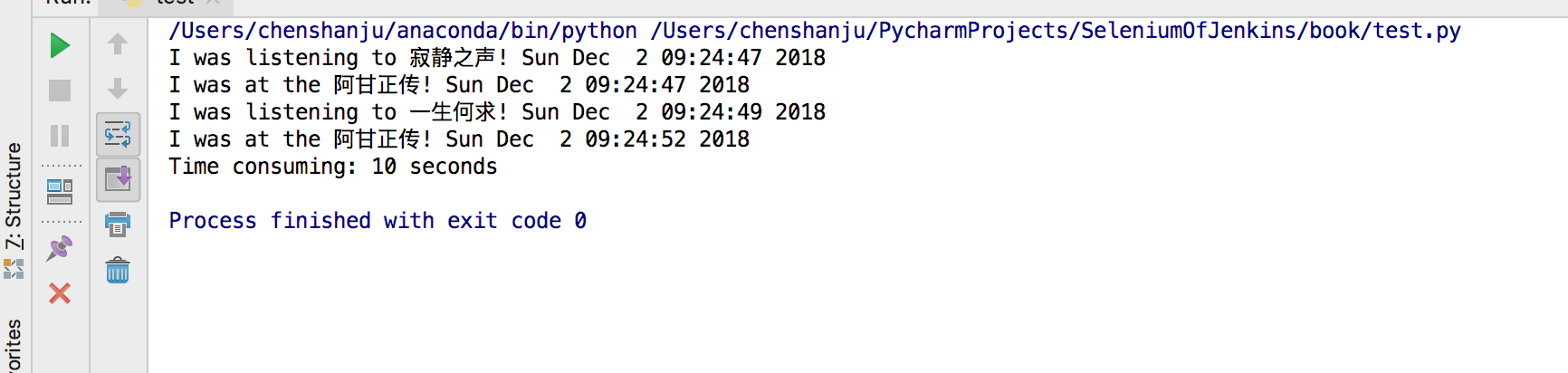
2.自定义线程类
from time import ctime,sleep,time
import threading
class MyThread(threading.Thread):
def __init__(self, func, args, name=''):
threading.Thread.__init__(self)
self.func = func
self.args = args
self.name = name
def run(self):
self.func(*self.args)
def super_play(file_, t):
for i in range(2):
print("Start playding: %s! %s"% (file_,ctime()))
sleep(t)
lists={"天边.mp3":3,"廊桥遗梦.rmvb":5,"壮志凌云。avi":4}
lists_len = range(len(lists))
threads = []
for file,t in lists.items():
t = MyThread(super_play,(file,t),super_play.__name__)
threads.append(t)
if __name__=="__main__":
start = time()
for i in lists_len:
threads[i].start()
for i in lists_len:
threads[i].join()
end = time()
ge = round((end-start),1)
print("共耗时%s秒"%ge)
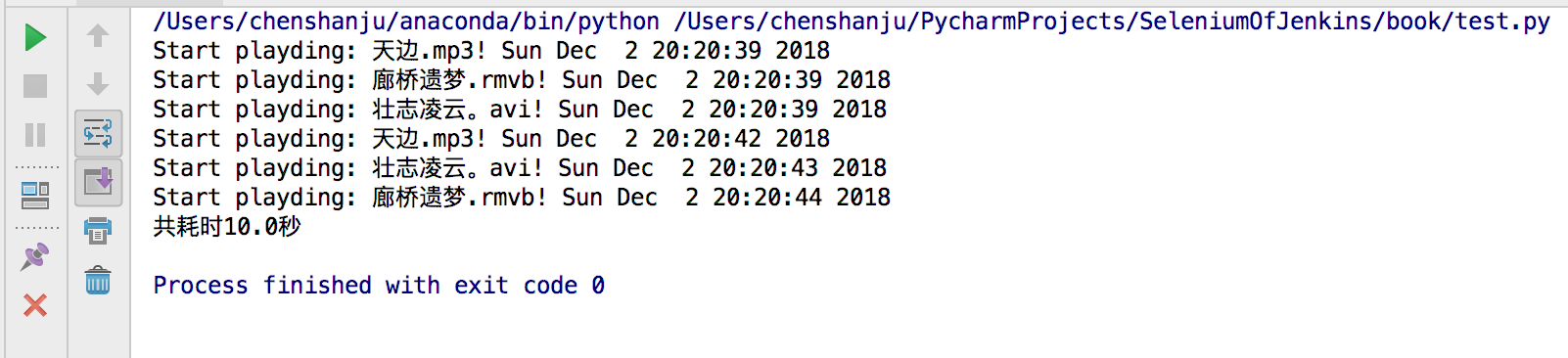
扩展:关于__name__
class TestName():
def func1(self):
print("执行func1")
print("func1.__name__:",func1.__name__)
def func2(self):
print("执行func2")
print("func2.__name__:",func2.__name__)
if __name__=="__main__":
print("TestName.__name__:",TestName.__name__)
print("py文件__name__:", __name__)
