题目:
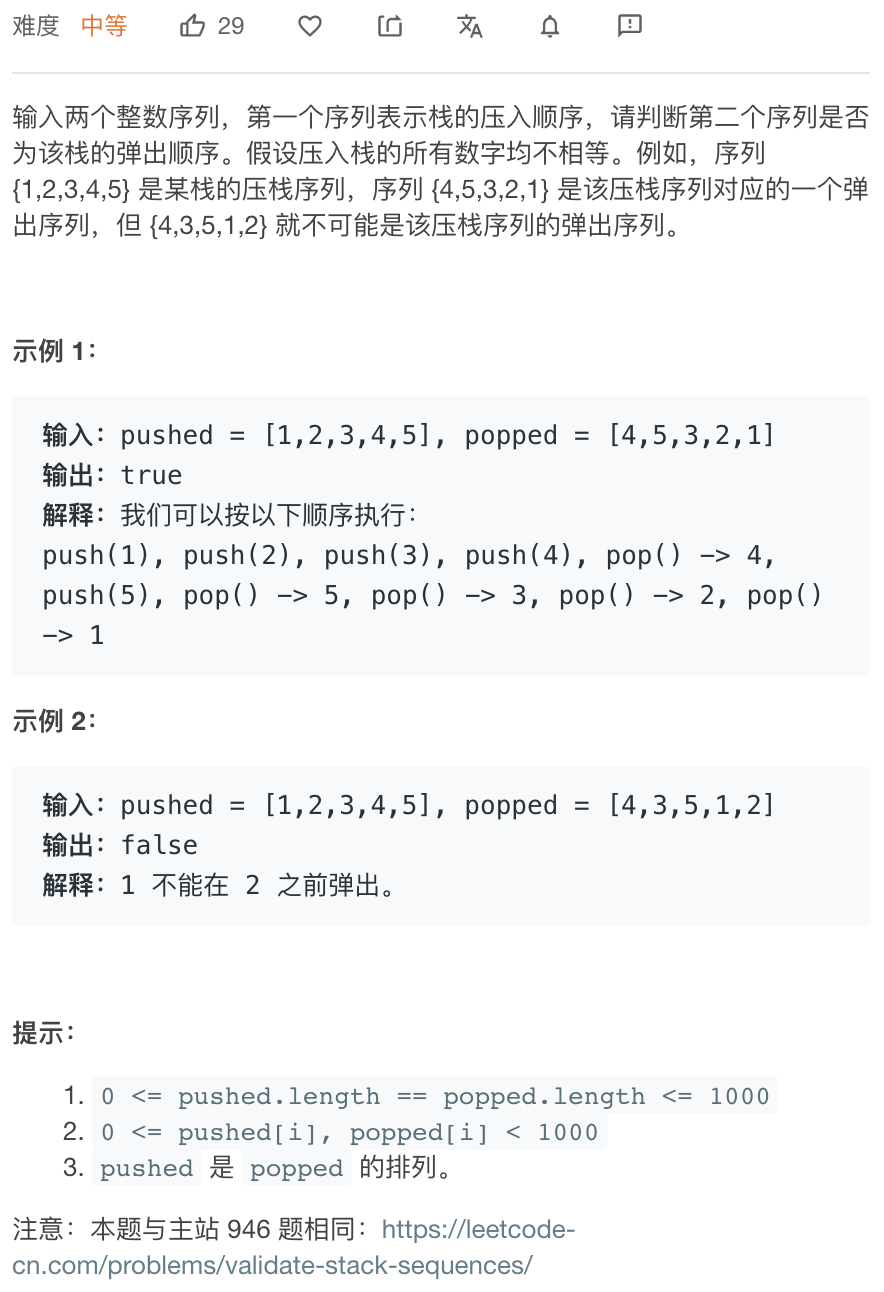
思路:
贪心思想按照popped的顺序模拟栈的弹出,如果栈顶元素等于popped当前元素则立即弹出,逻辑思考。可以分别以popped和pushed作为主循环编写代码。
代码:
Python
# popped作为主循环
class Solution(object):
def validateStackSequences(self, pushed, popped):
"""
:type pushed: List[int]
:type popped: List[int]
:rtype: bool
"""
stack = []
if not pushed and not popped:
return True
if len(pushed) != len(popped):
return False
pushIndex = 0
popIndex = 0
while popIndex < len(popped):
# 栈为空或者栈顶元素不匹配则入栈
if not stack or popped[popIndex] != stack[-1]:
# 加入一个待匹配元素
if pushIndex < len(pushed):
stack.append(pushed[pushIndex])
pushIndex += 1
else:
return False
# 栈顶元素匹配上
else:
stack.pop()
popIndex += 1
return True if not stack else False
# pushed作为主循环(pushed和popped长度相同)
class Solution(object):
def validateStackSequences(self, pushed, popped):
"""
:type pushed: List[int]
:type popped: List[int]
:rtype: bool
"""
stack = []
if not pushed and not popped:
return True
if len(pushed) != len(popped):
return False
i = 0
for elem in pushed:
stack.append(elem)
while stack and popped[i] == stack[-1]:
stack.pop()
i += 1
return not stack