题目:
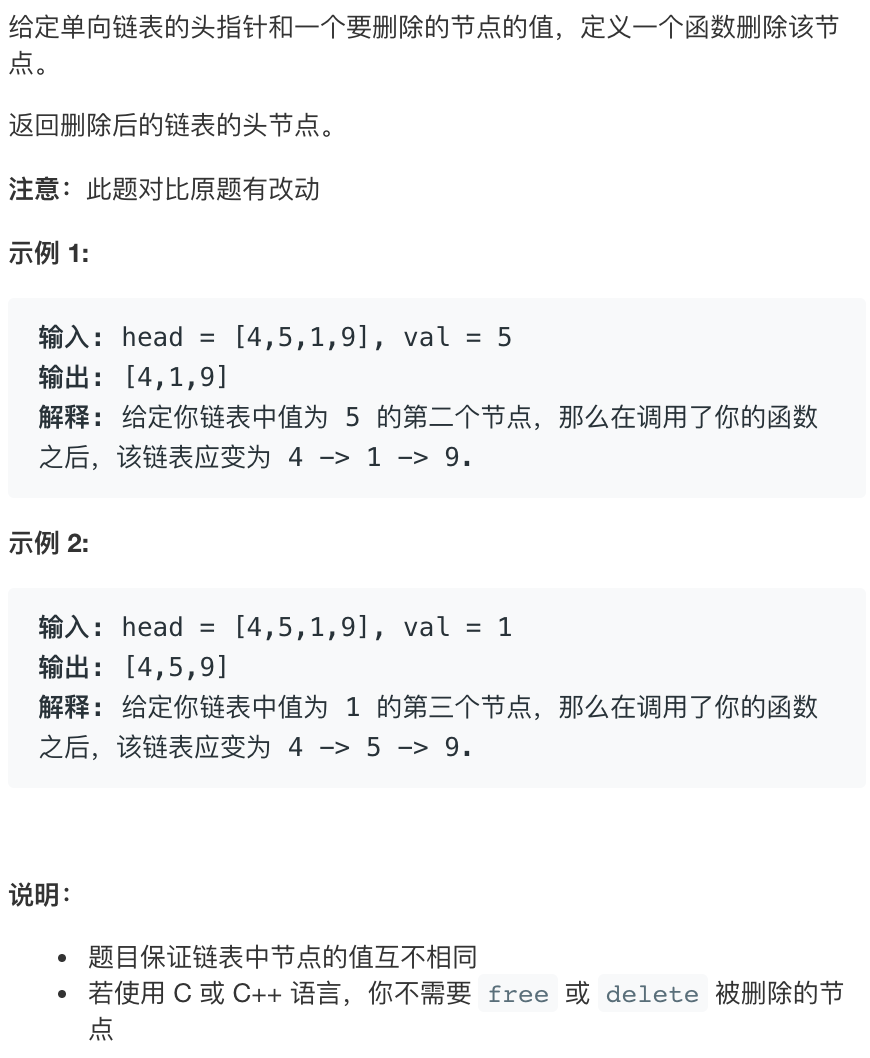
思路:
单链表的删除(无重复元素),特殊情况链表为空或链表头元素为要删除的元素(此时返回的head修改了),其它情况记录上个节点和当前节点,删除后返回head即可。
代码:
Python
# Definition for singly-linked list.
# class ListNode(object):
# def __init__(self, x):
# self.val = x
# self.next = None
class Solution(object):
def deleteNode(self, head, val):
"""
:type head: ListNode
:type val: int
:rtype: ListNode
"""
if head is None:
return None
if head.val == val:
return head.next
preElem = head
nowElem = head.next
while nowElem is not None:
if nowElem.val == val:
preElem.next = nowElem.next
nowElem.next = None
return head
else:
preElem = nowElem
nowElem = nowElem.next
return head