Spring MVC属于SpringFrameWork的后续产品,已经融合在Spring Web Flow里面。Spring 框架提供了构建 Web 应用程序的全功能 MVC 模块。使用 Spring 可插入的 MVC 架构,从而在使用Spring进行WEB开发时,可以选择使用Spring的SpringMVC框架或集成其他MVC开发框架,如Struts1,Struts2等。
通过策略接口,Spring 框架是高度可配置的,而且包含多种视图技术,例如 JavaServer Pages(JSP)技术、Velocity、Tiles、iText和POI。Spring MVC 框架并不知道使用的视图,所以不会强迫您只使用 JSP 技术。Spring MVC 分离了控制器、模型对象、过滤器以及处理程序对象的角色,这种分离让它们更容易进行定制。
Lifecycle for overriding binding, validation, etc,易于同其它View框架(Tiles等)无缝集成,采用IOC便于测试。
它是一个典型的教科书式的mvc构架,而不像struts等都是变种或者不是完全基于mvc系统的框架,对于初学者或者想了解mvc的人来说我觉得 spring是最好的,它的实现就是教科书!第二它和tapestry一样是一个纯正的servlet系统,这也是它和tapestry相比 struts所具有的优势。而且框架本身有代码,看起来容易理解。
搭建环境:
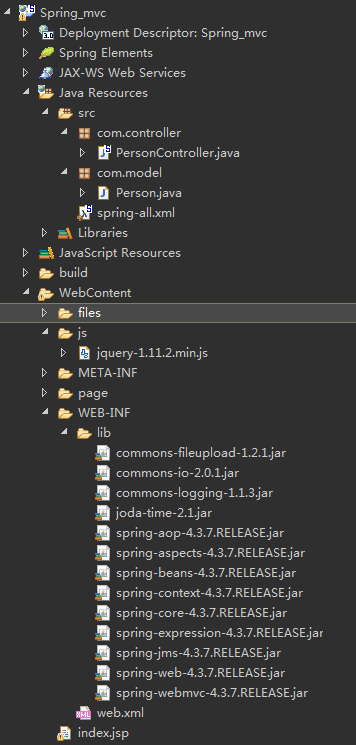
一、导包
二、配置
首先编写spring的配置文件 spring-all.xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xmlns:mvc="http://www.springframework.org/schema/mvc" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.3.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-4.3.xsd"> <context:component-scan base-package="com.*" /> <mvc:annotation-driven /> <!-- 视图解析器 --> <bean class="org.springframework.web.servlet.view.InternalResourceViewResolver"> <property name="prefix" value="/page/"></property> <property name="suffix" value=".jsp"></property> </bean> <!-- 文件上传需要配置的类 --> <bean id="multipartResolver" class="org.springframework.web.multipart.commons.CommonsMultipartResolver"> <!-- 文件总大小(单位是b) --> <property name="maxUploadSize" value="10000000"></property> <!-- 单个文件大小 --> <property name="maxUploadSizePerFile" value="2000000"></property> <!-- 字符集 --> <property name="defaultEncoding" value="utf-8"></property> </bean> <!-- 转换编码为utf-8的bean --> <bean class="org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerAdapter"> <property name="messageConverters"> <list> <bean class="org.springframework.http.converter.StringHttpMessageConverter"> <property name="supportedMediaTypes"> <list> <value>text/plain;charset=UTF-8</value> </list> </property> </bean> </list> </property> </bean> </beans>
编写web.xml
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0"> <filter> <filter-name>characterEncodingFilter</filter-name> <filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class> <init-param> <param-name>encoding</param-name> <param-value>UTF-8</param-value> </init-param> <init-param> <param-name>forceEncoding</param-name> <param-value>true</param-value> </init-param> </filter> <filter-mapping> <filter-name>characterEncodingFilter</filter-name> <url-pattern>/*</url-pattern> </filter-mapping> <!-- The front controller of this Spring Web application, responsible for handling all application requests --> <servlet> <servlet-name>springmvc</servlet-name> <servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class> <init-param> <param-name>contextConfigLocation</param-name> <param-value>classpath:spring-all.xml</param-value> </init-param> <!-- 启动时加载 --> <load-on-startup>1</load-on-startup> </servlet> <!-- Map all requests to the DispatcherServlet for handling --> <servlet-mapping> <servlet-name>springmvc</servlet-name> <url-pattern>*.do</url-pattern> </servlet-mapping> </web-app>
定义一个实体类
package com.model; import java.util.Date; import org.springframework.format.annotation.DateTimeFormat; public class Person { private Integer id; private String name; @DateTimeFormat(pattern="yyyyMMdd") private Date birthday; public Integer getId() { return id; } public void setId(Integer id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public Date getBirthday() { return birthday; } public void setBirthday(Date birthday) { this.birthday = birthday; } @Override public String toString() { return "Person [id=" + id + ", name=" + name + ", birthday=" + birthday + "]"; } }
编写具体实现的控制文件 PersonController.java
package com.controller; import java.io.File; import java.io.IOException; import java.util.Date; import javax.servlet.http.HttpServletRequest; import org.apache.commons.io.FileUtils; import org.springframework.format.annotation.DateTimeFormat; import org.springframework.http.HttpHeaders; import org.springframework.http.HttpStatus; import org.springframework.http.MediaType; import org.springframework.http.ResponseEntity; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import org.springframework.web.bind.annotation.RequestParam; import org.springframework.web.bind.annotation.ResponseBody; import org.springframework.web.multipart.MultipartFile; import com.model.Person; @Controller public class PersonController { //页面跳转 @RequestMapping("test") public String test(){ return "success"; } //传值,字符串和数字 @RequestMapping("test0/{ss}") public String test0(@PathVariable("ss")String str){ System.out.println(str); //System.out.println(num); return "error"; } @RequestMapping(value="test1",method={RequestMethod.POST,RequestMethod.GET}) public String test1(@RequestParam("str")String str,@RequestParam("num")int num){ System.out.println(str); System.out.println(num); return "error"; } @RequestMapping(value="test2") public String test2(@RequestParam("str")String str,@RequestParam("num")int num){ System.out.println(str); System.out.println(num); return "error"; } //传日期型参数 @RequestMapping("test3") public String test3(@RequestParam("date")@DateTimeFormat(pattern="yyyyMMdd")Date date){ System.out.println(date); return "success"; } //传递实体类 @RequestMapping("test4") public String test4(Person p){ System.out.println(p); return "success"; } //传递数组 @RequestMapping("test5") public String test5(@RequestParam("strs[]")String[] strs){ for(String s:strs){ System.out.println(s); } return "success"; } //带日期型参数的实体类 @RequestMapping("test6") public String test6(Person p){ System.out.println(p); return "success"; } @ResponseBody @RequestMapping("test7") public String test7(Person p){ System.out.println(p); String json = "{"success":true}"; return json; } //@ResponseBody @RequestMapping("test8") public String test8(@RequestParam("file")MultipartFile file,HttpServletRequest request){ String path = request.getServletContext().getRealPath("/files"); String fileName = file.getOriginalFilename(); System.out.println(fileName); fileName+=new Date().getTime(); File filenew = new File(path+"/"+fileName); try { file.transferTo(filenew); } catch (IllegalStateException e) { // TODO Auto-generated catch block e.printStackTrace(); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } return "success"; } @RequestMapping("testm") public String testm(@RequestParam("file")MultipartFile[] files,HttpServletRequest request){ String path = request.getServletContext().getRealPath("/files"); for(int i=0;i<files.length;i++){ String fileName = files[i].getOriginalFilename(); System.out.println(fileName); fileName+=new Date().getTime(); File filenew = new File(path+"/"+fileName); try { files[i].transferTo(filenew); } catch (IllegalStateException e) { // TODO Auto-generated catch block e.printStackTrace(); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } } return "success"; } @RequestMapping(value = "test9") // public ResponseEntity<byte[]> download(String fileName, File file) throws // IOException { public ResponseEntity<byte[]> download(HttpServletRequest request, @RequestParam("filename") String filename) throws IOException { //filename = new String(filename.getBytes("UTF-8"), "iso-8859-1");// 为了解决中文名称乱码问题 String path = request.getServletContext().getRealPath("/files"); path += "\" + filename; File file = new File(path); HttpHeaders headers = new HttpHeaders(); headers.setContentDispositionFormData("attachment",filename); headers.setContentType(MediaType.APPLICATION_OCTET_STREAM); return new ResponseEntity<byte[]>(FileUtils.readFileToByteArray(file), headers, HttpStatus.CREATED); } }
定义一个jsp页面进行显示
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> <script type="text/javascript" src="js/jquery-1.11.2.min.js"></script> </head> <body> <a href="test.do">测试</a> <a href="test0/haha.do">测试0</a><br> <a href="test1.do?str=nihao&num=123">测试1</a><br> <form action="test2.do" method="post"> <input type="text" name="str"> <input type="text" name="num"> <input type="submit" value="提交"> </form><br> <a href="test3.do?date=20170419">测试2</a><br> <form action="test4.do" method="post"> <input type="text" name="id"> <input type="text" name="name"> <input type="submit" value="提交"> </form><br> <form action="test5.do" method="post"> <input type="text" name="strs[]"> <input type="text" name="strs[]"> <input type="submit" value="提交"> </form><br> <form action="test6.do" method="post"> <input type="text" name="id"> <input type="text" name="name"> <input type="text" name="birthday"> <input type="submit" value="提交"> </form><br> <form id="form"> <input type="text" name="id"> <input type="text" name="name"> <input type="text" name="birthday"> <input id="btn" type="button" value="提交"> </form><br> <form action="test8.do" method="post" enctype="multipart/form-data"> <input type="file" name="file" /> <input type="submit" value="文件上传" /> </form><br> <form action="testm.do" method="post" enctype="multipart/form-data"> <input multiple="true" type="file" name="file" /> <input type="submit" value="文件上传" /> </form><br> <a href="test9.do?filename=jsonarray.rb">下载测试-jsonarray.rb</a><br> <a href="test9.do?filename=content.docx">下载测试-content.docx</a><br> <script type="text/javascript"> $(function(){ $("#btn").click(function(){ var f = $("#form").serializeArray(); $.ajax({ url:"test7.do", data:f, dataType:"json", type:"POST", success:function(d){ console.log(d); }, error:function(msg){ console.log(msg.responseText); } }); }); }); /* $(function(){ $("#btn_upload").click(function(){ var f = $("#formupload").serializeArray(); $.ajax({ url:"test8.do", data:f, dataType:"json", type:"POST", success:function(d){ console.log(d); }, error:function(msg){ console.log(msg.responseText); } }); }); }); */ </script> </body> </html>
实现效果如下: