最近学习asp.netcore 打算写出来和大家分享,我计划先写Identity部分,会从开始asp.netocre identity的简单实用开始,然后再去讲解主要的类和自定义这些类。
主题:asp.netcore Identity 的简单实用
创建项目:
使用asp.netcore 项目模版创建一个空(empty)项目,创建完成之后编辑.csproj文件,代码如下
<Project Sdk="Microsoft.NET.Sdk.Web"> <PropertyGroup> <TargetFramework>netcoreapp2.0</TargetFramework> </PropertyGroup> <ItemGroup> <Folder Include="wwwroot" /> </ItemGroup> <ItemGroup> <PackageReference Include="Microsoft.AspNetCore.All" Version="2.0.0" /> <DotNetCliToolReference Include="Microsoft.EntityFrameworkCore.Tools.DotNet" Version="2.0.0" /> </ItemGroup> </Project>
增加了
<DotNetCliToolReference Include="Microsoft.EntityFrameworkCore.Tools.DotNet"
Version="2.0.0" /> 这个行代码;
然后编辑 startup代码文件,增加mvc,和认证部分
public void ConfigureServices(IServiceCollection services) { services.AddMvc(); } public void Configure(IApplicationBuilder app) { app.UseStatusCodePages(); app.UseDeveloperExceptionPage(); app.UseStaticFiles(); app.UseMvcWithDefaultRoute(); }
配置Identity
现在我们配置identity的信息,包括配置的修改,添加user类
1创建user类
user类代表了用户的账号信息,比如登陆名,密码,角色,email等信息,代码如下:
using Microsoft.AspNetCore.Identity; namespace DemoUser.Models { public class AppUser:IdentityUser { } }
我们是继承了 identityuser这个类,这个类已经包含了基本的用户信息属性,比如 登陆名,密码 ,手机号等,该类的反编译之后信息如下:
// Decompiled with JetBrains decompiler // Type: Microsoft.AspNetCore.Identity.IdentityUser`1 // Assembly: Microsoft.Extensions.Identity.Stores, Version=2.0.1.0, Culture=neutral, PublicKeyToken=adb9793829ddae60 // MVID: 7E04453E-9787-4C8F-ACD1-4ADA9BB7C88C // Assembly location: /usr/local/share/dotnet/sdk/NuGetFallbackFolder/microsoft.extensions.identity.stores/2.0.1/lib/netstandard2.0/Microsoft.Extensions.Identity.Stores.dll using System; namespace Microsoft.AspNetCore.Identity { /// <summary>Represents a user in the identity system</summary> /// <typeparam name="TKey">The type used for the primary key for the user.</typeparam> public class IdentityUser<TKey> where TKey : IEquatable<TKey> { /// <summary> /// Initializes a new instance of <see cref="T:Microsoft.AspNetCore.Identity.IdentityUser`1" />. /// </summary> public IdentityUser() { } /// <summary> /// Initializes a new instance of <see cref="T:Microsoft.AspNetCore.Identity.IdentityUser`1" />. /// </summary> /// <param name="userName">The user name.</param> public IdentityUser(string userName) : this() { this.UserName = userName; } /// <summary>Gets or sets the primary key for this user.</summary> public virtual TKey Id { get; set; } /// <summary>Gets or sets the user name for this user.</summary> public virtual string UserName { get; set; } /// <summary>Gets or sets the normalized user name for this user.</summary> public virtual string NormalizedUserName { get; set; } /// <summary>Gets or sets the email address for this user.</summary> public virtual string Email { get; set; } /// <summary> /// Gets or sets the normalized email address for this user. /// </summary> public virtual string NormalizedEmail { get; set; } /// <summary> /// Gets or sets a flag indicating if a user has confirmed their email address. /// </summary> /// <value>True if the email address has been confirmed, otherwise false.</value> public virtual bool EmailConfirmed { get; set; } /// <summary> /// Gets or sets a salted and hashed representation of the password for this user. /// </summary> public virtual string PasswordHash { get; set; } /// <summary> /// A random value that must change whenever a users credentials change (password changed, login removed) /// </summary> public virtual string SecurityStamp { get; set; } /// <summary> /// A random value that must change whenever a user is persisted to the store /// </summary> public virtual string ConcurrencyStamp { get; set; } = Guid.NewGuid().ToString(); /// <summary>Gets or sets a telephone number for the user.</summary> public virtual string PhoneNumber { get; set; } /// <summary> /// Gets or sets a flag indicating if a user has confirmed their telephone address. /// </summary> /// <value>True if the telephone number has been confirmed, otherwise false.</value> public virtual bool PhoneNumberConfirmed { get; set; } /// <summary> /// Gets or sets a flag indicating if two factor authentication is enabled for this user. /// </summary> /// <value>True if 2fa is enabled, otherwise false.</value> public virtual bool TwoFactorEnabled { get; set; } /// <summary> /// Gets or sets the date and time, in UTC, when any user lockout ends. /// </summary> /// <remarks>A value in the past means the user is not locked out.</remarks> public virtual DateTimeOffset? LockoutEnd { get; set; } /// <summary> /// Gets or sets a flag indicating if the user could be locked out. /// </summary> /// <value>True if the user could be locked out, otherwise false.</value> public virtual bool LockoutEnabled { get; set; } /// <summary> /// Gets or sets the number of failed login attempts for the current user. /// </summary> public virtual int AccessFailedCount { get; set; } /// <summary>Returns the username for this user.</summary> public override string ToString() { return this.UserName; } } }
这个类已经定义好了一些关于账户的属性,,假如我们觉得还需要添加自定义的属性比如 用户的地址,我们只需要在我们继承的类添加自定义的属性即可;
创建数据库上下文:
下面我们要创建一个数据上下文用于连接数据库,代码如下:
using Microsoft.AspNetCore.Identity.EntityFrameworkCore; using Microsoft.EntityFrameworkCore; namespace DemoUser.Models { public class AppIdentityDbContext :IdentityDbContext<AppUser> { public AppIdentityDbContext(DbContextOptions<AppIdentityDbContext> options) : base(options) { } } }
配置数据库连接字符串:
为了连接数据库我们要配置一个连接字符串,修改appsettings.json文件如下:
{ "Data": { "AppStoreIdentity": { "ConnectionString": "Server=192.168.0.4;Database=IdentityUsers;User ID =SA; Password=!@#" } } }
下面我们需要在startup类配置连接字符串到数据库上下文;
public void ConfigureServices(IServiceCollection services) { services.AddDbContext<AppIdentityDbContext>(options => options.UseSqlServer( Configuration["Data:AppStoreIdentity:ConnectionString"])); services.AddIdentity<AppUser, IdentityRole>() .AddEntityFrameworkStores<AppIdentityDbContext>() .AddDefaultTokenProviders(); services.AddMvc(); }
创建数据库:
下面我们需要用EFCore去生成一个数据库:
打开控制台,定位到当前的项目,输入:
dotnet ef migrations add Initial
然后就会产生一个migrations文件夹,里面是我们要生成数据库的代码;
然后 在控制台输入:
dotnet ef database update
这样我们的数据库就生成了
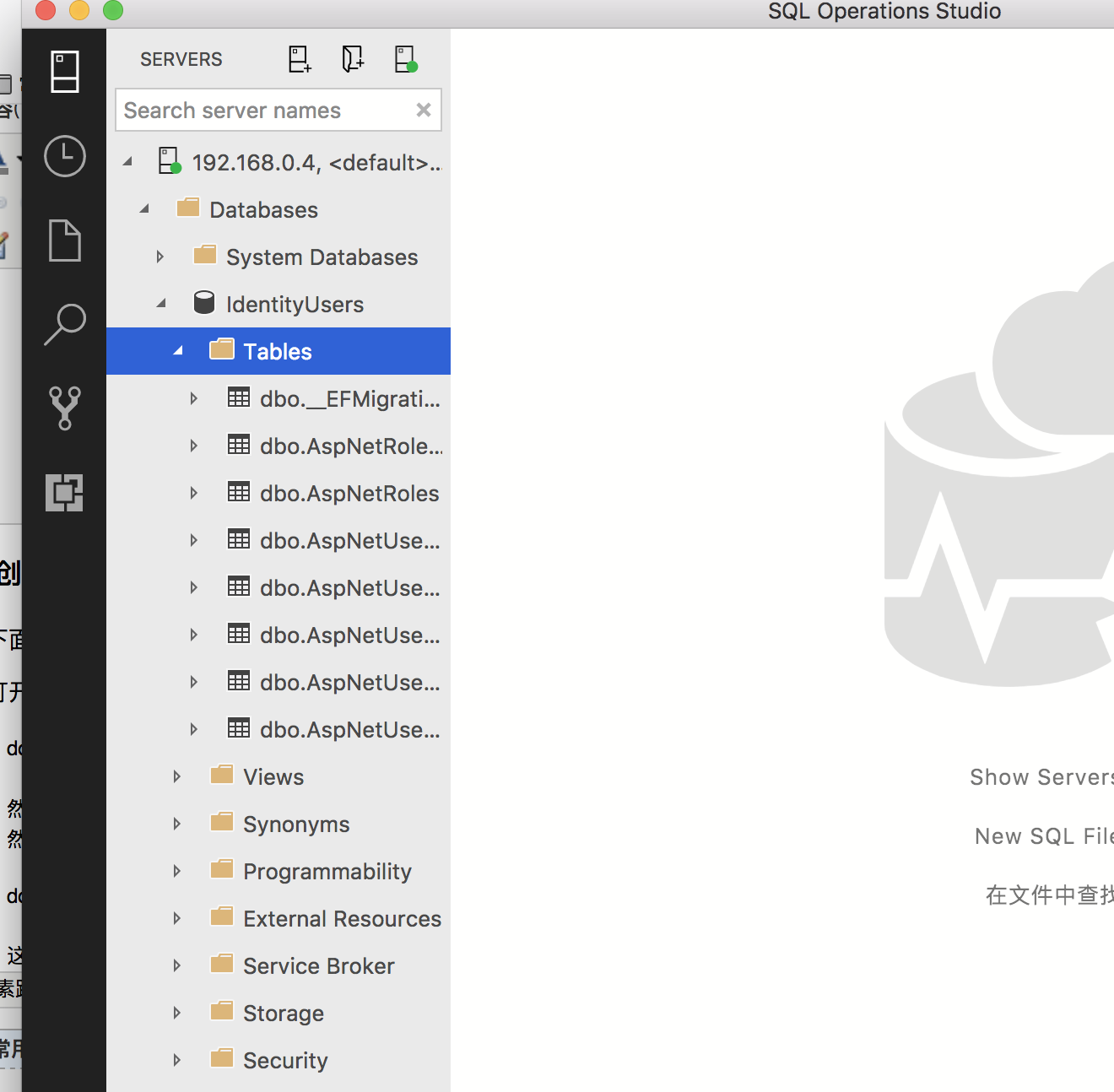
这里是我上传的github仓库地址:https://github.com/bluetianx/AspnetCoreExample
后续:
我会创建一个具有增删改查的账户管理界面