1.基础排序的介绍
2.基数排序的基本思想

图解:
假设给[53,3,542,748,14,214]排序
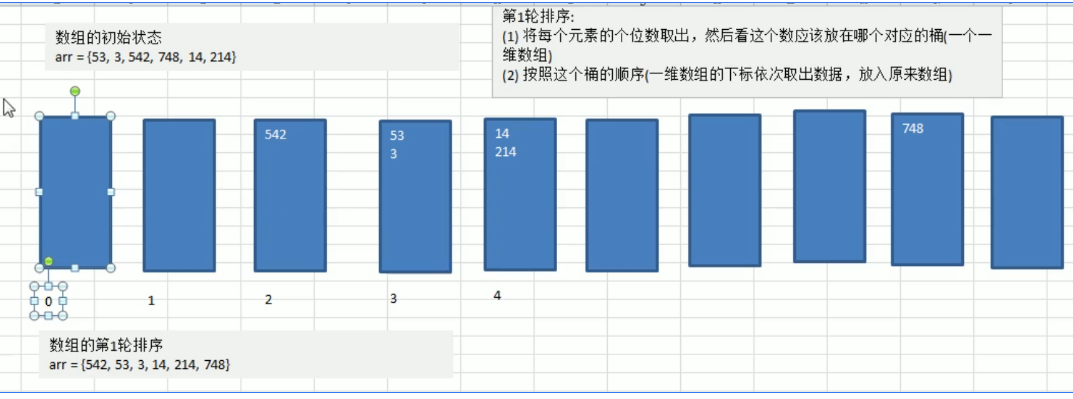
3.代码实现
1 // 基数排序 2 public class RadixSort { 3 public static void main(String[] args) { 4 // int[] arr = {53, 3, 542, 748, 14, 214,57,88,73,24,99,11,111,222,23423,1438}; 5 int[] arr = new int[1000000]; 6 for (int i = 0,j = 1000000; i < 1000000; i++,j--) { 7 arr[i] = j; 8 } 9 long start = System.currentTimeMillis(); 10 sort(arr); 11 long end = System.currentTimeMillis(); 12 System.out.println("用时:"+(end-start)+"ms"); 13 } 14 15 16 private static void sort(int[] arr) { 17 // 首先遍历一次数组,找出最大值 18 int max = arr[0]; 19 for (int i = 1; i < arr.length; i++) { 20 if (max < arr[i]) 21 max = arr[i]; 22 } 23 // 获得最大值的长度,这个值会决定需要进行几轮排序 24 int maxLength = (max + "").length(); 25 // 准备10个桶存放数据,用二维数组bucket[][]表示,bucket[0]表示所有基数为0的数,bucket[1]表示所有基数为1的数,依次递推... 26 int[][] bucket = new int[10][arr.length]; 27 // 准备一个一维数组来记录每个桶里存放了几个数据 28 int[] bucketLength = new int[10]; 29 // maxLength排序 30 for (int i = 0, n = 1; i < maxLength; i++, n = n * 10) { 31 // 遍历数组 32 for (int j = 0; j < arr.length; j++) { 33 // 第1轮取个位基数,第2轮取百位基数...递推 34 int base = arr[j] / n % 10; 35 // 把数据存入对应的桶中 36 bucket[base][bucketLength[base]] = arr[j]; 37 bucketLength[base]++; 38 } 39 40 int index = 0; 41 // 将桶中数据依次写回到数组中 42 for (int j = 0; j < 10; j++) { 43 if(bucketLength[j] == 0) 44 continue; 45 for (int jj = 0; jj < bucketLength[j]; jj++) { 46 arr[index++] = bucket[j][jj]; 47 } 48 // 清空bucketLength中的记录 49 bucketLength[j] = 0; 50 } 51 } 52 } 53 }
4.小结