1.GameObject.Find
函数原型:
public static GameObject Find(string name);
说明:1.GameObject只能查找到active的物体
2.如果name指定路径,则按路径查找;否则递归查找,直到查找到第一个符合条件的GameObject或者返回null
2.transform.Find
函数原型:
public Transform Find(string n);
说明:1.transform.Find用于查找子节点,它并不会递归的查找物体,也就是说它只会查找它的子节点,并不会查找子节点的子节点。
用代码验证:
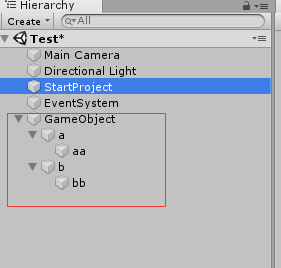
1 public class TestFind : MonoBehaviour 2 { 3 4 public string name = ""; 5 private void Start() 6 { 7 Transform t = transform.Find(name); 8 if(t != null) 9 print("找到了"); 10 else 11 { 12 print("没找到"); 13 } 14 } 15 }
说明:TestFind脚本挂在GameObject物体上。
1.name为a, 输出找到了
2.name为aa,输出没找到
3.name为b,输出找到了
4.name为bb,输出没找到
补充:在实际开发中可能遇到这样的情况,给定一个节点,在这个子节点中递归的查找符合条件的节点。transform.Find不能递归查找,不能直接使用,GameObject.Find又做了很多无用功,关键若是重名还不一定能满足需求。于是我们可以自己写一个递归程序:
1 public static Transform FindChildRecursively(Transform parent, string name) 2 { 3 Transform t = null; 4 t = parent.Find(name); 5 if (t == null) 6 { 7 foreach (Transform tran in parent) 8 { 9 t = FindChildRecursively(tran, name); 10 if (t != null) 11 { 12 return t; 13 } 14 } 15 } 16 17 return t; 18 } 19 }