- 所需资源
- JavaMail API jar包
- mail.properties
文件:
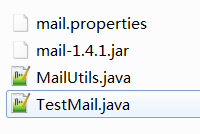
import java.io.BufferedInputStream;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.util.Date;
import java.util.Properties;
import javax.mail.Authenticator;
import javax.mail.Message;
import javax.mail.Message.RecipientType;
import javax.mail.MessagingException;
import javax.mail.PasswordAuthentication;
import javax.mail.Session;
import javax.mail.Transport;
import javax.mail.internet.AddressException;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeMessage;
public class MailUtils {
private static Session session = null;
private static String myEmailAccount;
private static String myEmailPassword;
private static String myEmailSMTPHost;
private MailUtils(){
}
private static Session getSession() throws IOException {
if (session == null) {
// 读取配置信息
Properties prop = new Properties();
InputStream ins = new BufferedInputStream(new FileInputStream("src/mail.properties"));
// InputStream ins =
// this.getClass().getResourceAsStream("/mail.properties");
prop.load(ins);
myEmailAccount = prop.getProperty("myEmailAccount");
myEmailPassword = prop.getProperty("myEmailPassword");
myEmailSMTPHost = prop.getProperty("myEmailSMTPHost");
// 1.创建连接对象,连接到邮件服务器
Properties props = new Properties();
// props.setProperty("host", value);
props.setProperty("mail.transport.protocol", "smtp");
props.setProperty("mail.smtp.host", myEmailSMTPHost);
props.setProperty("mail.smtp.auth", "true");
session = Session.getInstance(props, new Authenticator() {
@Override
protected PasswordAuthentication getPasswordAuthentication() {
// 发送帐号
return new PasswordAuthentication(myEmailAccount, myEmailPassword);
}
});
}
return session;
}
/**
* 根据配置文件发送邮件的方法
*
* @param toAddress
* :收件人地址
* @param code
* :激活码
* @throws IOException
* @throws MessagingException
* @throws AddressException
* @throws Exception
*/
public static void sendMailByNet2(String toAddress, String subject, String content)
throws IOException, AddressException, MessagingException {
Session session = getSession();
session.setDebug(true);
// 2.创建邮件对象
Message message = new MimeMessage(session);
// 2.1设置发件人
message.setFrom(new InternetAddress(myEmailAccount));
// 2.2设置收件人
message.setRecipient(RecipientType.TO, new InternetAddress(toAddress));
// 2.3设置邮件主题
message.setSubject(subject);
// 2.4设置邮件的正文
message.setContent(content, "text/html;charset=UTF-8");
message.setSentDate(new Date());
message.saveChanges();
// 3.发送激活邮件
Transport.send(message);
/*Transport transport = session.getTransport();
transport.connect(myEmailAccount, myEmailPassword);
transport.sendMessage(message, message.getAllRecipients());
transport.close();
*/
}
public static void main(String[] args) {
long startTime = System.currentTimeMillis();
try {
sendMailByNet2("bigzxxxx@qq.com", "测试邮件1", "Hello");
} catch (AddressException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} catch (MessagingException e) {
e.printStackTrace();
}
long endTime = System.currentTimeMillis();
System.out.println(endTime - startTime + "ms");
}
}
#mail.properties
mail.transport.protocol=smtp
myEmailSMTPHost=smtp.163.com
myEmailAccount=xxxx@163.com
myEmailPassword=xxx
#password可能不是网页邮箱的登录密码,注意查看,一般QQ需要设置第三方的授权登录密码,此处填写第三方的授权登录密码