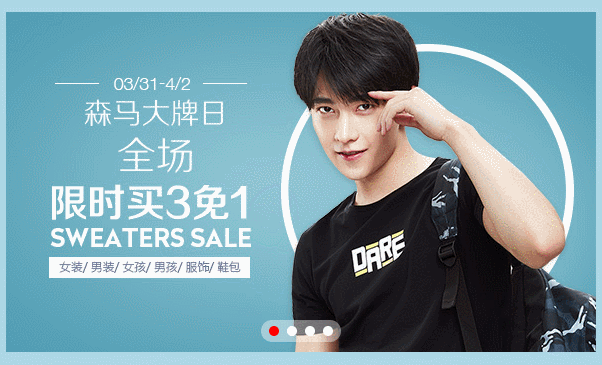
HTML
<!DOCTYPE html> <html> <head> <meta charset="utf-8" /> <title>轮播图</title> <link rel="stylesheet" href="css/common.css"/> <link rel="stylesheet" href="css/carousel.css"/> </head> <body> <div class="carousel"> <ul class="carousel-list"> <li><img src="img/1.jpg" /></li> <li><img src="img/2.jpg" /></li> <li><img src="img/3.jpg" /></li> <li><img src="img/4.jpg" /></li> </ul> <div class="direction-btn"> <a href="javascript:;" class="prev">></a> <a href="javascript:;" class="next"><</a> </div> <div class="dots"> <a href="javascript:;" class="active"></a> <a href="javascript:;"></a> <a href="javascript:;"></a> <a href="javascript:;"></a> </div> </div> <script src="js/common.js"></script> <script src="js/carousel.js"></script> </body> </html>
common.js
/* 缓冲运动 obj:运动的对象 target:运动属性和终点值的对象 fn:回调函数 ratio:运动系数,默认值为8 */ function bufferMove(obj,target,fn,ratio){ var ratio = ratio || 8; clearInterval(obj.timer); obj.timer = setInterval(function(){ var allOK = true; for(var attr in target){ var cur = 0; if(attr === 'opacity'){ cur = parseInt(getStyle(obj,'opacity') * 100); } else{ cur = parseInt(getStyle(obj,attr)); } var speed = (target[attr] - cur) / ratio; speed = speed > 0? Math.ceil(speed) : Math.floor(speed); var next = cur + speed; if(attr === 'opacity'){ obj.style.opacity = next /100; obj.style.filter = 'alpha( opacity = ' + next + ')'; } else{ obj.style[attr] = next + 'px'; } if(next !== target[attr]){ allOK = false; } } if(allOK){ clearInterval(obj.timer); if(fn){ fn(); } } },50); } // ///* // 获取当前样式值 //*/ function getStyle(obj, attr) { if(obj.currentStyle) { return obj.currentStyle[attr]; } else { return getComputedStyle(obj, false)[attr]; } } //多属性函数封装 //封装获取样式的函数 // function getStyle(obj,attr){ // if(obj.currentStyle){ // return obj.currentStyle[attr]; // } else { // return getComputedStyle(obj,false)[attr]; // } // } //封装类名的获取 function byClassName(sClassName){ if(document.getElementsByClassName){ return document.getElementsByClassName(sClassName); } else { var all = document.getElementsByTagName('*'); var result = []; for(var i = 0;i <all.length;i++){ if(all[i].className === sClassName){ result.push(all[i]); } } return result; } } carousel.js // 获取两个点击事件的元素 var oBtn = byClassName('direction-btn')[0], // 获取大盒子 oCarousel = byClassName('carousel')[0], // 获取存储图片的ul oCarouselList = byClassName('carousel-list')[0], // 获取ul下的li oCarouselLi = oCarouselList.children, // 获取上一个的点击按钮 oPrev = byClassName('prev')[0], // 获取下一个的点击按钮 oNext = byClassName('next')[0], // 记录图片下标 index = 0; // 定义点的下标 dotIndex = 0; // 定义常量图片的宽度 const iPerW = 590; // 定义定时器 var timer = null; var oDots = byClassName('dots')[0]; var oDotsA = Array.from(oDots.children); //遍历a oDotsA.forEach((v,k) =>{ v.onmouseover = function(){ index = k; dotIndex = k; bufferMove(oCarouselList,{left:-index * iPerW}); setDotClass(); } }) function setDotClass(){ //遍历每个a标签 oDotsA.forEach(v => v.className=''); // 右侧a判断 if(dotIndex >= oDotsA.length){ dotIndex = 0; } // 左侧a判断 if(dotIndex < 0){ dotIndex = oDotsA.length - 1; } oDotsA[dotIndex].className = 'active'; } //添加第一张图片到末尾 oCarouselList.innerHTML += oCarouselLi[0].outerHTML; //定义ul的总宽度 oCarouselList.style.width = iPerW * oCarouselLi.length + 'px'; oCarousel.onmouseover = function(){ clearInterval(timer); oBtn.style.display = 'block'; } oCarousel.onmouseout = function(){ autoMove(); oBtn.style.display = 'none'; } //添加点击事件 oNext.onclick = function(){ rightMove(); } function rightMove(){ index++; dotIndex++; if(index >= oCarouselLi.length){ index = 1; oCarouselList.style.left = '0px'; } bufferMove(oCarouselList,{left:-index * iPerW}); setDotClass(); } //添加左侧点击事件 oPrev.onclick = function(){ index--; dotIndex--; if(index < 0){ index = oCarouselLi.length - 2; oCarouselList.style.left = - (oCarouselLi.length - 1) * iPerW + 'px'; } bufferMove(oCarouselList,{left:-index * iPerW}); setDotClass(); } //自动播放 autoMove(); function autoMove(){ timer = setInterval(function(){ rightMove(); },3000); }
css文件
body,div,dl,dt,dd,ul,ol,li,h1,h2,h3,h4,h5,h6,pre,form,fieldset,input,textarea,p,blockquote,th,td{ margin: 0; padding: 0; } body{ font-family:"Mrcrosoft Yahei",Arial; } ul,ol{ list-style: none; } a{ text-decoration: none; } img{ border:none; } body{ background:lightblue; } .carousel{ 590px; height: 340px; margin: 50px auto; overflow: hidden; position: relative; } .carousel-list{ position: absolute; } .carousel-list li{ float: left; } .direction-btn{ display: none; } .direction-btn a{ position: absolute; top: 50%; margin-top: -30px; 30px; line-height: 60px; background: rgba(0, 0, 0, .3); color: #fff; font-size: 30px; text-align: center; } .prev { left: 0; } .next { right: 0; } .dots{ position: absolute; background:rgba(255,255,255,.4); border-radius: 12px; 72px; left: 0; right: 0; bottom: 10px; margin: 0 auto; font-size: 0; line-height: 0; padding: 0 4px; } .dots a{ display: inline-block; 10px; height: 10px; border-radius: 50%; background: #fff; margin: 6px 4px; } .dots .active{ background: red; }
图片更换成自己的路径即可