一,创建command
1,创建command
liuhongdi@lhdpc:/data/php/admapi$ php think make:command SetOrderStatus setorderstatus Command:app\command\SetOrderStatus created successfully.
2,查看已创建的command程序
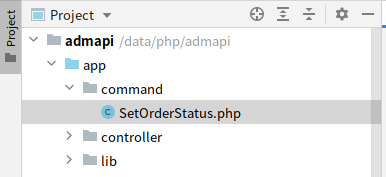
3,配置command可运行:
修改config/console.php
<?php // +---------------------------------------------------------------------- // | 控制台配置 // +---------------------------------------------------------------------- return [ // 指令定义 'commands' => [ 'setorderstatus' => 'app\command\SetOrderStatus', ], ];
4,在command\SetOrderStatus.php增加一行输出:
class SetOrderStatus extends Command { protected function configure() { // 指令配置 $this->setName('setorderstatus') ->setDescription('the setorderstatus command'); } protected function execute(Input $input, Output $output) { echo "this is in command\n"; // 指令输出 $output->writeln('setorderstatus'); } }
5,测试:
liuhongdi@lhdpc:/data/php/admapi$ php think setorderstatus this is in command setorderstatus
说明:刘宏缔的架构森林是一个专注架构的博客,地址:https://www.cnblogs.com/architectforest
对应的源码可以访问这里获取: https://github.com/liuhongdi/
或: https://gitee.com/liuhongdi
说明:作者:刘宏缔 邮箱: 371125307@qq.com
二,编写功能:查询所有超过半小时未支付的订单
1,创建order的model
liuhongdi@lhdpc:/data/php/admapi$ php think make:model Order Model:app\model\Order created successfully.
2,编写order的model类代码:
<?php declare (strict_types = 1); namespace app\model; use think\facade\Db; use think\Model; /** * @mixin \think\Model */ class Order extends Model { //类名与表名不一致时在这里指定数据表名 protected $table = "orderInfo"; public function getAllNotPayOrder(){ //得到30分钟以前的时间 $before30min = date("Y-m-d H:i:s",strtotime("-30 minutes")); //查询多条记录时用select方法 $result = Db::table("orderInfo")->where("orderStatus",0)->where("addTime","<",$before30min)->select(); return $result; } }
3,在command中调用:
command/SetOrderStatus.php
<?php declare (strict_types = 1); namespace app\command; use think\console\Command; use think\console\Input; use think\console\input\Argument; use think\console\input\Option; use think\console\Output; use app\model\Order as OrderModel; class SetOrderStatus extends Command { protected function configure() { // 指令配置 $this->setName('setorderstatus') ->setDescription('the setorderstatus command'); } protected function execute(Input $input, Output $output) { echo "this is in command\n"; //得到所有的未支付订单 $order = new OrderModel(); $rows = $order->getAllNotPayOrder(); //遍历订单,修改状态 foreach($rows as $k => $one) { echo "orderId:".$one['orderId']."\n"; echo "orderStatus:".$one['orderStatus']."\n"; } // 指令输出 $output->writeln('setorderstatus'); } }
4,测试效果:
liuhongdi@lhdpc:/data/php/admapi$ php think setorderstatus this is in command orderId:1 orderStatus:0 orderId:2 orderStatus:0 setorderstatus
三,测试添加command到crontab中执行:
1,确认crond服务是否在运行:
root@lhdpc:~# systemctl status cron.service ● cron.service - Regular background program processing daemon Loaded: loaded (/lib/systemd/system/cron.service; enabled; vendor preset: enabled) Active: active (running) since Sun 2022-01-02 10:19:23 CST; 4h 14min ago Docs: man:cron(8) Main PID: 637 (cron) Tasks: 1 (limit: 4588) Memory: 572.0K CPU: 158ms CGroup: /system.slice/cron.service └─637 /usr/sbin/cron -f -P 1月 02 13:49:32 lhdpc CRON[2645]: pam_unix(cron:session): session closed for user root 1月 02 13:49:32 lhdpc CRON[2647]: pam_unix(cron:session): session opened for user root by (uid=0) 1月 02 13:49:32 lhdpc CRON[2648]: (root) CMD ([ -x /etc/init.d/anacron ] && if [ ! -d /run/systemd/system ]; then /> 1月 02 13:49:32 lhdpc CRON[2647]: pam_unix(cron:session): session closed for user root 1月 02 14:17:01 lhdpc CRON[3128]: pam_unix(cron:session): session opened for user root by (uid=0) 1月 02 14:17:01 lhdpc CRON[3129]: (root) CMD ( cd / && run-parts --report /etc/cron.hourly) 1月 02 14:17:01 lhdpc CRON[3128]: pam_unix(cron:session): session closed for user root 1月 02 14:30:01 lhdpc CRON[3225]: pam_unix(cron:session): session opened for user root by (uid=0) 1月 02 14:30:01 lhdpc CRON[3226]: (root) CMD ([ -x /etc/init.d/anacron ] && if [ ! -d /run/systemd/system ]; then /> 1月 02 14:30:01 lhdpc CRON[3225]: pam_unix(cron:session): session closed for user root
2,添加到crontab
root@lhdpc:~# crontab -u www-data -e no crontab for www-data - using an empty one Select an editor. To change later, run 'select-editor'. 1. /bin/nano <---- easiest 2. /usr/bin/vim.basic 3. /usr/bin/vim.tiny 4. /bin/ed Choose 1-4 [1]: 2
3,查看内容:
root@lhdpc:~# crontab -u www-data -l # ... # m h dom mon dow command 1,11,21,31,41,51 * * * * /usr/local/soft/php8/bin/php /data/php/admapi/think setorderstatus >>/data/phplog/commandlog/setorderstatus_`date -d 'today' +\%Y-\%m-\%d`.log 2>&1
4,查看日志:
liuhongdi@lhdpc:/data/phplog/commandlog$ more setorderstatus_2022-01-02.log this is in command orderId:1 orderStatus:0 orderId:2 orderStatus:0 setorderstatus
5,说明:crontab 用-u指定用户名
是为了和web的用户一致,
如果web和定时任务在一台机器上运行,可能会造成日志文件的所有者不同,
导致php运行失败
6,说明:命令 `date -d 'today' +\%Y-\%m-\%d` 的用途:
使日志按日期切分
四,查看php和thinkphp的版本:
php:
liuhongdi@lhdpc:/data/php/admapi$ php --version PHP 8.1.1 (cli) (built: Dec 20 2021 16:12:16) (NTS) Copyright (c) The PHP Group Zend Engine v4.1.1, Copyright (c) Zend Technologies with Zend OPcache v8.1.1, Copyright (c), by Zend Technologies
thinkphp:
liuhongdi@lhdpc:/var/www/html$ cd /data/php/admapi/ liuhongdi@lhdpc:/data/php/admapi$ php think version v6.0.10LTS